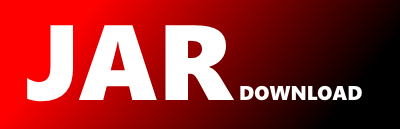
com.pulumi.linode.inputs.GetFirewallsFirewallOutboundArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
public final class GetFirewallsFirewallOutboundArgs extends com.pulumi.resources.ResourceArgs {
public static final GetFirewallsFirewallOutboundArgs Empty = new GetFirewallsFirewallOutboundArgs();
/**
* Controls whether traffic is accepted or dropped by this rule (ACCEPT, DROP).
*
*/
@Import(name="action", required=true)
private Output action;
/**
* @return Controls whether traffic is accepted or dropped by this rule (ACCEPT, DROP).
*
*/
public Output action() {
return this.action;
}
/**
* A list of IPv4 addresses or networks in IP/mask format.
*
*/
@Import(name="ipv4s", required=true)
private Output> ipv4s;
/**
* @return A list of IPv4 addresses or networks in IP/mask format.
*
*/
public Output> ipv4s() {
return this.ipv4s;
}
/**
* A list of IPv6 addresses or networks in IP/mask format.
*
*/
@Import(name="ipv6s", required=true)
private Output> ipv6s;
/**
* @return A list of IPv6 addresses or networks in IP/mask format.
*
*/
public Output> ipv6s() {
return this.ipv6s;
}
/**
* The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
*/
@Import(name="label", required=true)
private Output label;
/**
* @return The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
*/
public Output label() {
return this.label;
}
/**
* A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
*/
@Import(name="ports", required=true)
private Output ports;
/**
* @return A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
*/
public Output ports() {
return this.ports;
}
/**
* The network protocol this rule controls. (TCP, UDP, ICMP)
*
*/
@Import(name="protocol", required=true)
private Output protocol;
/**
* @return The network protocol this rule controls. (TCP, UDP, ICMP)
*
*/
public Output protocol() {
return this.protocol;
}
private GetFirewallsFirewallOutboundArgs() {}
private GetFirewallsFirewallOutboundArgs(GetFirewallsFirewallOutboundArgs $) {
this.action = $.action;
this.ipv4s = $.ipv4s;
this.ipv6s = $.ipv6s;
this.label = $.label;
this.ports = $.ports;
this.protocol = $.protocol;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFirewallsFirewallOutboundArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetFirewallsFirewallOutboundArgs $;
public Builder() {
$ = new GetFirewallsFirewallOutboundArgs();
}
public Builder(GetFirewallsFirewallOutboundArgs defaults) {
$ = new GetFirewallsFirewallOutboundArgs(Objects.requireNonNull(defaults));
}
/**
* @param action Controls whether traffic is accepted or dropped by this rule (ACCEPT, DROP).
*
* @return builder
*
*/
public Builder action(Output action) {
$.action = action;
return this;
}
/**
* @param action Controls whether traffic is accepted or dropped by this rule (ACCEPT, DROP).
*
* @return builder
*
*/
public Builder action(String action) {
return action(Output.of(action));
}
/**
* @param ipv4s A list of IPv4 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv4s(Output> ipv4s) {
$.ipv4s = ipv4s;
return this;
}
/**
* @param ipv4s A list of IPv4 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv4s(List ipv4s) {
return ipv4s(Output.of(ipv4s));
}
/**
* @param ipv4s A list of IPv4 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv4s(String... ipv4s) {
return ipv4s(List.of(ipv4s));
}
/**
* @param ipv6s A list of IPv6 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv6s(Output> ipv6s) {
$.ipv6s = ipv6s;
return this;
}
/**
* @param ipv6s A list of IPv6 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv6s(List ipv6s) {
return ipv6s(Output.of(ipv6s));
}
/**
* @param ipv6s A list of IPv6 addresses or networks in IP/mask format.
*
* @return builder
*
*/
public Builder ipv6s(String... ipv6s) {
return ipv6s(List.of(ipv6s));
}
/**
* @param label The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
* @return builder
*
*/
public Builder label(Output label) {
$.label = label;
return this;
}
/**
* @param label The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
* @return builder
*
*/
public Builder label(String label) {
return label(Output.of(label));
}
/**
* @param ports A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
* @return builder
*
*/
public Builder ports(Output ports) {
$.ports = ports;
return this;
}
/**
* @param ports A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
* @return builder
*
*/
public Builder ports(String ports) {
return ports(Output.of(ports));
}
/**
* @param protocol The network protocol this rule controls. (TCP, UDP, ICMP)
*
* @return builder
*
*/
public Builder protocol(Output protocol) {
$.protocol = protocol;
return this;
}
/**
* @param protocol The network protocol this rule controls. (TCP, UDP, ICMP)
*
* @return builder
*
*/
public Builder protocol(String protocol) {
return protocol(Output.of(protocol));
}
public GetFirewallsFirewallOutboundArgs build() {
if ($.action == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "action");
}
if ($.ipv4s == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "ipv4s");
}
if ($.ipv6s == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "ipv6s");
}
if ($.label == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "label");
}
if ($.ports == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "ports");
}
if ($.protocol == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewallOutboundArgs", "protocol");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy