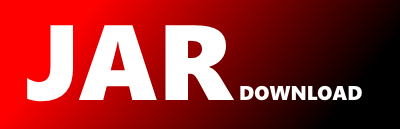
com.pulumi.linode.inputs.GetImagesImage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.inputs;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.linode.inputs.GetImagesImageReplication;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetImagesImage extends com.pulumi.resources.InvokeArgs {
public static final GetImagesImage Empty = new GetImagesImage();
/**
* The capabilities of this Image.
*
*/
@Import(name="capabilities", required=true)
private List capabilities;
/**
* @return The capabilities of this Image.
*
*/
public List capabilities() {
return this.capabilities;
}
/**
* When this Image was created.
*
*/
@Import(name="created", required=true)
private String created;
/**
* @return When this Image was created.
*
*/
public String created() {
return this.created;
}
/**
* The name of the User who created this Image, or "linode" for official Images.
*
*/
@Import(name="createdBy", required=true)
private String createdBy;
/**
* @return The name of the User who created this Image, or "linode" for official Images.
*
*/
public String createdBy() {
return this.createdBy;
}
/**
* Whether or not this Image is deprecated. Will only be true for deprecated public Images.
*
*/
@Import(name="deprecated", required=true)
private Boolean deprecated;
/**
* @return Whether or not this Image is deprecated. Will only be true for deprecated public Images.
*
*/
public Boolean deprecated() {
return this.deprecated;
}
/**
* A detailed description of this Image.
*
*/
@Import(name="description", required=true)
private String description;
/**
* @return A detailed description of this Image.
*
*/
public String description() {
return this.description;
}
/**
* Only Images created automatically (from a deleted Linode; type=automatic) will expire.
*
*/
@Import(name="expiry", required=true)
private String expiry;
/**
* @return Only Images created automatically (from a deleted Linode; type=automatic) will expire.
*
*/
public String expiry() {
return this.expiry;
}
/**
* The unique ID of this Image. The ID of private images begin with `private/` followed by the numeric identifier of the private image, for example `private/12345`.
*
*/
@Import(name="id", required=true)
private String id;
/**
* @return The unique ID of this Image. The ID of private images begin with `private/` followed by the numeric identifier of the private image, for example `private/12345`.
*
*/
public String id() {
return this.id;
}
/**
* True if the Image is public.
*
*/
@Import(name="isPublic", required=true)
private Boolean isPublic;
/**
* @return True if the Image is public.
*
*/
public Boolean isPublic() {
return this.isPublic;
}
/**
* A short description of the Image.
*
*/
@Import(name="label", required=true)
private String label;
/**
* @return A short description of the Image.
*
*/
public String label() {
return this.label;
}
/**
* A list of image replication regions and corresponding status.
*
*/
@Import(name="replications")
private @Nullable List replications;
/**
* @return A list of image replication regions and corresponding status.
*
*/
public Optional> replications() {
return Optional.ofNullable(this.replications);
}
/**
* The minimum size this Image needs to deploy. Size is in MB. example: 2500
*
*/
@Import(name="size", required=true)
private Integer size;
/**
* @return The minimum size this Image needs to deploy. Size is in MB. example: 2500
*
*/
public Integer size() {
return this.size;
}
/**
* The status of an image replica.
*
*/
@Import(name="status", required=true)
private String status;
/**
* @return The status of an image replica.
*
*/
public String status() {
return this.status;
}
/**
* A list of customized tags.
*
*/
@Import(name="tags", required=true)
private List tags;
/**
* @return A list of customized tags.
*
*/
public List tags() {
return this.tags;
}
/**
* The total size of the image in all available regions.
*
*/
@Import(name="totalSize", required=true)
private Integer totalSize;
/**
* @return The total size of the image in all available regions.
*
*/
public Integer totalSize() {
return this.totalSize;
}
/**
* How the Image was created. Manual Images can be created at any time. "Automatic" Images are created automatically from a deleted Linode. (`manual`, `automatic`)
*
*/
@Import(name="type", required=true)
private String type;
/**
* @return How the Image was created. Manual Images can be created at any time. "Automatic" Images are created automatically from a deleted Linode. (`manual`, `automatic`)
*
*/
public String type() {
return this.type;
}
/**
* The upstream distribution vendor. `None` for private Images.
*
*/
@Import(name="vendor", required=true)
private String vendor;
/**
* @return The upstream distribution vendor. `None` for private Images.
*
*/
public String vendor() {
return this.vendor;
}
private GetImagesImage() {}
private GetImagesImage(GetImagesImage $) {
this.capabilities = $.capabilities;
this.created = $.created;
this.createdBy = $.createdBy;
this.deprecated = $.deprecated;
this.description = $.description;
this.expiry = $.expiry;
this.id = $.id;
this.isPublic = $.isPublic;
this.label = $.label;
this.replications = $.replications;
this.size = $.size;
this.status = $.status;
this.tags = $.tags;
this.totalSize = $.totalSize;
this.type = $.type;
this.vendor = $.vendor;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetImagesImage defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetImagesImage $;
public Builder() {
$ = new GetImagesImage();
}
public Builder(GetImagesImage defaults) {
$ = new GetImagesImage(Objects.requireNonNull(defaults));
}
/**
* @param capabilities The capabilities of this Image.
*
* @return builder
*
*/
public Builder capabilities(List capabilities) {
$.capabilities = capabilities;
return this;
}
/**
* @param capabilities The capabilities of this Image.
*
* @return builder
*
*/
public Builder capabilities(String... capabilities) {
return capabilities(List.of(capabilities));
}
/**
* @param created When this Image was created.
*
* @return builder
*
*/
public Builder created(String created) {
$.created = created;
return this;
}
/**
* @param createdBy The name of the User who created this Image, or "linode" for official Images.
*
* @return builder
*
*/
public Builder createdBy(String createdBy) {
$.createdBy = createdBy;
return this;
}
/**
* @param deprecated Whether or not this Image is deprecated. Will only be true for deprecated public Images.
*
* @return builder
*
*/
public Builder deprecated(Boolean deprecated) {
$.deprecated = deprecated;
return this;
}
/**
* @param description A detailed description of this Image.
*
* @return builder
*
*/
public Builder description(String description) {
$.description = description;
return this;
}
/**
* @param expiry Only Images created automatically (from a deleted Linode; type=automatic) will expire.
*
* @return builder
*
*/
public Builder expiry(String expiry) {
$.expiry = expiry;
return this;
}
/**
* @param id The unique ID of this Image. The ID of private images begin with `private/` followed by the numeric identifier of the private image, for example `private/12345`.
*
* @return builder
*
*/
public Builder id(String id) {
$.id = id;
return this;
}
/**
* @param isPublic True if the Image is public.
*
* @return builder
*
*/
public Builder isPublic(Boolean isPublic) {
$.isPublic = isPublic;
return this;
}
/**
* @param label A short description of the Image.
*
* @return builder
*
*/
public Builder label(String label) {
$.label = label;
return this;
}
/**
* @param replications A list of image replication regions and corresponding status.
*
* @return builder
*
*/
public Builder replications(@Nullable List replications) {
$.replications = replications;
return this;
}
/**
* @param replications A list of image replication regions and corresponding status.
*
* @return builder
*
*/
public Builder replications(GetImagesImageReplication... replications) {
return replications(List.of(replications));
}
/**
* @param size The minimum size this Image needs to deploy. Size is in MB. example: 2500
*
* @return builder
*
*/
public Builder size(Integer size) {
$.size = size;
return this;
}
/**
* @param status The status of an image replica.
*
* @return builder
*
*/
public Builder status(String status) {
$.status = status;
return this;
}
/**
* @param tags A list of customized tags.
*
* @return builder
*
*/
public Builder tags(List tags) {
$.tags = tags;
return this;
}
/**
* @param tags A list of customized tags.
*
* @return builder
*
*/
public Builder tags(String... tags) {
return tags(List.of(tags));
}
/**
* @param totalSize The total size of the image in all available regions.
*
* @return builder
*
*/
public Builder totalSize(Integer totalSize) {
$.totalSize = totalSize;
return this;
}
/**
* @param type How the Image was created. Manual Images can be created at any time. "Automatic" Images are created automatically from a deleted Linode. (`manual`, `automatic`)
*
* @return builder
*
*/
public Builder type(String type) {
$.type = type;
return this;
}
/**
* @param vendor The upstream distribution vendor. `None` for private Images.
*
* @return builder
*
*/
public Builder vendor(String vendor) {
$.vendor = vendor;
return this;
}
public GetImagesImage build() {
if ($.capabilities == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "capabilities");
}
if ($.created == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "created");
}
if ($.createdBy == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "createdBy");
}
if ($.deprecated == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "deprecated");
}
if ($.description == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "description");
}
if ($.expiry == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "expiry");
}
if ($.id == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "id");
}
if ($.isPublic == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "isPublic");
}
if ($.label == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "label");
}
if ($.size == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "size");
}
if ($.status == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "status");
}
if ($.tags == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "tags");
}
if ($.totalSize == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "totalSize");
}
if ($.type == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "type");
}
if ($.vendor == null) {
throw new MissingRequiredPropertyException("GetImagesImage", "vendor");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy