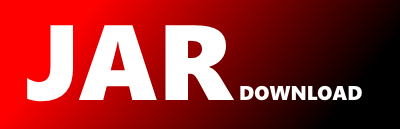
com.pulumi.linode.outputs.GetNetworkingIpResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetNetworkingIpResult {
/**
* @return The IP address.
*
*/
private String address;
/**
* @return The default gateway for this address.
*
*/
private String gateway;
private String id;
/**
* @return The ID of the Linode this address currently belongs to.
*
*/
private Integer linodeId;
/**
* @return The number of bits set in the subnet mask.
*
*/
private Integer prefix;
/**
* @return Whether this is a public or private IP address.
*
*/
private Boolean public_;
/**
* @return The reverse DNS assigned to this address. For public IPv4 addresses, this will be set to a default value provided by Linode if not explicitly set.
*
*/
private String rdns;
/**
* @return The Region this IP address resides in. See all regions [here](https://api.linode.com/v4/regions).
*
*/
private String region;
/**
* @return The mask that separates host bits from network bits for this address.
*
*/
private String subnetMask;
/**
* @return The type of address this is (ipv4, ipv6, ipv6/pool, ipv6/range).
*
*/
private String type;
private GetNetworkingIpResult() {}
/**
* @return The IP address.
*
*/
public String address() {
return this.address;
}
/**
* @return The default gateway for this address.
*
*/
public String gateway() {
return this.gateway;
}
public String id() {
return this.id;
}
/**
* @return The ID of the Linode this address currently belongs to.
*
*/
public Integer linodeId() {
return this.linodeId;
}
/**
* @return The number of bits set in the subnet mask.
*
*/
public Integer prefix() {
return this.prefix;
}
/**
* @return Whether this is a public or private IP address.
*
*/
public Boolean public_() {
return this.public_;
}
/**
* @return The reverse DNS assigned to this address. For public IPv4 addresses, this will be set to a default value provided by Linode if not explicitly set.
*
*/
public String rdns() {
return this.rdns;
}
/**
* @return The Region this IP address resides in. See all regions [here](https://api.linode.com/v4/regions).
*
*/
public String region() {
return this.region;
}
/**
* @return The mask that separates host bits from network bits for this address.
*
*/
public String subnetMask() {
return this.subnetMask;
}
/**
* @return The type of address this is (ipv4, ipv6, ipv6/pool, ipv6/range).
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNetworkingIpResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String address;
private String gateway;
private String id;
private Integer linodeId;
private Integer prefix;
private Boolean public_;
private String rdns;
private String region;
private String subnetMask;
private String type;
public Builder() {}
public Builder(GetNetworkingIpResult defaults) {
Objects.requireNonNull(defaults);
this.address = defaults.address;
this.gateway = defaults.gateway;
this.id = defaults.id;
this.linodeId = defaults.linodeId;
this.prefix = defaults.prefix;
this.public_ = defaults.public_;
this.rdns = defaults.rdns;
this.region = defaults.region;
this.subnetMask = defaults.subnetMask;
this.type = defaults.type;
}
@CustomType.Setter
public Builder address(String address) {
if (address == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "address");
}
this.address = address;
return this;
}
@CustomType.Setter
public Builder gateway(String gateway) {
if (gateway == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "gateway");
}
this.gateway = gateway;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder linodeId(Integer linodeId) {
if (linodeId == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "linodeId");
}
this.linodeId = linodeId;
return this;
}
@CustomType.Setter
public Builder prefix(Integer prefix) {
if (prefix == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "prefix");
}
this.prefix = prefix;
return this;
}
@CustomType.Setter("public")
public Builder public_(Boolean public_) {
if (public_ == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "public_");
}
this.public_ = public_;
return this;
}
@CustomType.Setter
public Builder rdns(String rdns) {
if (rdns == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "rdns");
}
this.rdns = rdns;
return this;
}
@CustomType.Setter
public Builder region(String region) {
if (region == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "region");
}
this.region = region;
return this;
}
@CustomType.Setter
public Builder subnetMask(String subnetMask) {
if (subnetMask == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "subnetMask");
}
this.subnetMask = subnetMask;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetNetworkingIpResult", "type");
}
this.type = type;
return this;
}
public GetNetworkingIpResult build() {
final var _resultValue = new GetNetworkingIpResult();
_resultValue.address = address;
_resultValue.gateway = gateway;
_resultValue.id = id;
_resultValue.linodeId = linodeId;
_resultValue.prefix = prefix;
_resultValue.public_ = public_;
_resultValue.rdns = rdns;
_resultValue.region = region;
_resultValue.subnetMask = subnetMask;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy