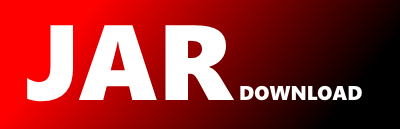
com.pulumi.linode.outputs.GetPlacementGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.linode.outputs.GetPlacementGroupMember;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetPlacementGroupResult {
private Integer id;
/**
* @return Whether this Linode is currently compliant with the group's placement group type.
*
*/
private Boolean isCompliant;
/**
* @return The label of the Placement Group. This field can only contain ASCII letters, digits and dashes.
*
*/
private String label;
/**
* @return A set of Linodes currently assigned to this Placement Group.
*
*/
private @Nullable List members;
/**
* @return Whether Linodes must be able to become compliant during assignment. (Default `strict`)
*
*/
private String placementGroupPolicy;
/**
* @return The placement group type to use when placing Linodes in this group.
*
*/
private String placementGroupType;
/**
* @return The region of the Placement Group.
*
*/
private String region;
private GetPlacementGroupResult() {}
public Integer id() {
return this.id;
}
/**
* @return Whether this Linode is currently compliant with the group's placement group type.
*
*/
public Boolean isCompliant() {
return this.isCompliant;
}
/**
* @return The label of the Placement Group. This field can only contain ASCII letters, digits and dashes.
*
*/
public String label() {
return this.label;
}
/**
* @return A set of Linodes currently assigned to this Placement Group.
*
*/
public List members() {
return this.members == null ? List.of() : this.members;
}
/**
* @return Whether Linodes must be able to become compliant during assignment. (Default `strict`)
*
*/
public String placementGroupPolicy() {
return this.placementGroupPolicy;
}
/**
* @return The placement group type to use when placing Linodes in this group.
*
*/
public String placementGroupType() {
return this.placementGroupType;
}
/**
* @return The region of the Placement Group.
*
*/
public String region() {
return this.region;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPlacementGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer id;
private Boolean isCompliant;
private String label;
private @Nullable List members;
private String placementGroupPolicy;
private String placementGroupType;
private String region;
public Builder() {}
public Builder(GetPlacementGroupResult defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.isCompliant = defaults.isCompliant;
this.label = defaults.label;
this.members = defaults.members;
this.placementGroupPolicy = defaults.placementGroupPolicy;
this.placementGroupType = defaults.placementGroupType;
this.region = defaults.region;
}
@CustomType.Setter
public Builder id(Integer id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isCompliant(Boolean isCompliant) {
if (isCompliant == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "isCompliant");
}
this.isCompliant = isCompliant;
return this;
}
@CustomType.Setter
public Builder label(String label) {
if (label == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "label");
}
this.label = label;
return this;
}
@CustomType.Setter
public Builder members(@Nullable List members) {
this.members = members;
return this;
}
public Builder members(GetPlacementGroupMember... members) {
return members(List.of(members));
}
@CustomType.Setter
public Builder placementGroupPolicy(String placementGroupPolicy) {
if (placementGroupPolicy == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "placementGroupPolicy");
}
this.placementGroupPolicy = placementGroupPolicy;
return this;
}
@CustomType.Setter
public Builder placementGroupType(String placementGroupType) {
if (placementGroupType == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "placementGroupType");
}
this.placementGroupType = placementGroupType;
return this;
}
@CustomType.Setter
public Builder region(String region) {
if (region == null) {
throw new MissingRequiredPropertyException("GetPlacementGroupResult", "region");
}
this.region = region;
return this;
}
public GetPlacementGroupResult build() {
final var _resultValue = new GetPlacementGroupResult();
_resultValue.id = id;
_resultValue.isCompliant = isCompliant;
_resultValue.label = label;
_resultValue.members = members;
_resultValue.placementGroupPolicy = placementGroupPolicy;
_resultValue.placementGroupType = placementGroupType;
_resultValue.region = region;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy