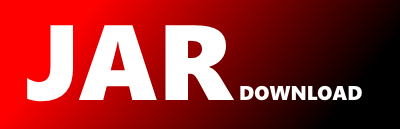
com.pulumi.linode.outputs.InstanceConfigDevices Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.linode.outputs.InstanceConfigDevicesSda;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdb;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdc;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdd;
import com.pulumi.linode.outputs.InstanceConfigDevicesSde;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdf;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdg;
import com.pulumi.linode.outputs.InstanceConfigDevicesSdh;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InstanceConfigDevices {
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSda sda;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdb sdb;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdc sdc;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdd sdd;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSde sde;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdf sdf;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdg sdg;
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
private @Nullable InstanceConfigDevicesSdh sdh;
private InstanceConfigDevices() {}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sda() {
return Optional.ofNullable(this.sda);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdb() {
return Optional.ofNullable(this.sdb);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdc() {
return Optional.ofNullable(this.sdc);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdd() {
return Optional.ofNullable(this.sdd);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sde() {
return Optional.ofNullable(this.sde);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdf() {
return Optional.ofNullable(this.sdf);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdg() {
return Optional.ofNullable(this.sdg);
}
/**
* @return Device can be either a Disk or Volume identified by disk_id or volume_id. Only one type per slot allowed.
*
*/
public Optional sdh() {
return Optional.ofNullable(this.sdh);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InstanceConfigDevices defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable InstanceConfigDevicesSda sda;
private @Nullable InstanceConfigDevicesSdb sdb;
private @Nullable InstanceConfigDevicesSdc sdc;
private @Nullable InstanceConfigDevicesSdd sdd;
private @Nullable InstanceConfigDevicesSde sde;
private @Nullable InstanceConfigDevicesSdf sdf;
private @Nullable InstanceConfigDevicesSdg sdg;
private @Nullable InstanceConfigDevicesSdh sdh;
public Builder() {}
public Builder(InstanceConfigDevices defaults) {
Objects.requireNonNull(defaults);
this.sda = defaults.sda;
this.sdb = defaults.sdb;
this.sdc = defaults.sdc;
this.sdd = defaults.sdd;
this.sde = defaults.sde;
this.sdf = defaults.sdf;
this.sdg = defaults.sdg;
this.sdh = defaults.sdh;
}
@CustomType.Setter
public Builder sda(@Nullable InstanceConfigDevicesSda sda) {
this.sda = sda;
return this;
}
@CustomType.Setter
public Builder sdb(@Nullable InstanceConfigDevicesSdb sdb) {
this.sdb = sdb;
return this;
}
@CustomType.Setter
public Builder sdc(@Nullable InstanceConfigDevicesSdc sdc) {
this.sdc = sdc;
return this;
}
@CustomType.Setter
public Builder sdd(@Nullable InstanceConfigDevicesSdd sdd) {
this.sdd = sdd;
return this;
}
@CustomType.Setter
public Builder sde(@Nullable InstanceConfigDevicesSde sde) {
this.sde = sde;
return this;
}
@CustomType.Setter
public Builder sdf(@Nullable InstanceConfigDevicesSdf sdf) {
this.sdf = sdf;
return this;
}
@CustomType.Setter
public Builder sdg(@Nullable InstanceConfigDevicesSdg sdg) {
this.sdg = sdg;
return this;
}
@CustomType.Setter
public Builder sdh(@Nullable InstanceConfigDevicesSdh sdh) {
this.sdh = sdh;
return this;
}
public InstanceConfigDevices build() {
final var _resultValue = new InstanceConfigDevices();
_resultValue.sda = sda;
_resultValue.sdb = sdb;
_resultValue.sdc = sdc;
_resultValue.sdd = sdd;
_resultValue.sde = sde;
_resultValue.sdf = sdf;
_resultValue.sdg = sdg;
_resultValue.sdh = sdh;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy