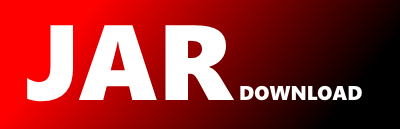
com.pulumi.linode.ImageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.linode.inputs.ImageTimeoutsArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ImageArgs extends com.pulumi.resources.ResourceArgs {
public static final ImageArgs Empty = new ImageArgs();
/**
* Whether this image supports cloud-init.
*
*/
@Import(name="cloudInit")
private @Nullable Output cloudInit;
/**
* @return Whether this image supports cloud-init.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy