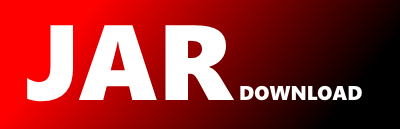
com.pulumi.linode.LkeClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.linode.inputs.LkeClusterControlPlaneArgs;
import com.pulumi.linode.inputs.LkeClusterPoolArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LkeClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final LkeClusterArgs Empty = new LkeClusterArgs();
/**
* Defines settings for the Kubernetes Control Plane.
*
*/
@Import(name="controlPlane")
private @Nullable Output controlPlane;
/**
* @return Defines settings for the Kubernetes Control Plane.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy