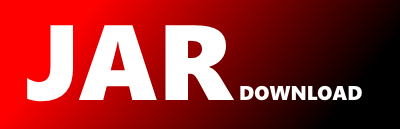
com.pulumi.linode.inputs.GetFirewallsFirewall Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.inputs;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.linode.inputs.GetFirewallsFirewallDevice;
import com.pulumi.linode.inputs.GetFirewallsFirewallInbound;
import com.pulumi.linode.inputs.GetFirewallsFirewallOutbound;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetFirewallsFirewall extends com.pulumi.resources.InvokeArgs {
public static final GetFirewallsFirewall Empty = new GetFirewallsFirewall();
/**
* When this firewall was created.
*
*/
@Import(name="created", required=true)
private String created;
/**
* @return When this firewall was created.
*
*/
public String created() {
return this.created;
}
/**
* The devices associated with this firewall.
*
*/
@Import(name="devices")
private @Nullable List devices;
/**
* @return The devices associated with this firewall.
*
*/
public Optional> devices() {
return Optional.ofNullable(this.devices);
}
/**
* If true, the Firewall is inactive.
*
*/
@Import(name="disabled", required=true)
private Boolean disabled;
/**
* @return If true, the Firewall is inactive.
*
*/
public Boolean disabled() {
return this.disabled;
}
/**
* The unique ID assigned to this Firewall.
*
*/
@Import(name="id", required=true)
private Integer id;
/**
* @return The unique ID assigned to this Firewall.
*
*/
public Integer id() {
return this.id;
}
/**
* The default behavior for inbound traffic.
*
*/
@Import(name="inboundPolicy", required=true)
private String inboundPolicy;
/**
* @return The default behavior for inbound traffic.
*
*/
public String inboundPolicy() {
return this.inboundPolicy;
}
/**
* A set of firewall rules that specify what inbound network traffic is allowed.
*
*/
@Import(name="inbounds")
private @Nullable List inbounds;
/**
* @return A set of firewall rules that specify what inbound network traffic is allowed.
*
*/
public Optional> inbounds() {
return Optional.ofNullable(this.inbounds);
}
/**
* The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
*/
@Import(name="label", required=true)
private String label;
/**
* @return The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
*/
public String label() {
return this.label;
}
/**
* The IDs of Linodes this firewall is applied to.
*
*/
@Import(name="linodes", required=true)
private List linodes;
/**
* @return The IDs of Linodes this firewall is applied to.
*
*/
public List linodes() {
return this.linodes;
}
/**
* The IDs of NodeBalancers assigned to this Firewall..
*
*/
@Import(name="nodebalancers", required=true)
private List nodebalancers;
/**
* @return The IDs of NodeBalancers assigned to this Firewall..
*
*/
public List nodebalancers() {
return this.nodebalancers;
}
/**
* The default behavior for outbound traffic.
*
*/
@Import(name="outboundPolicy", required=true)
private String outboundPolicy;
/**
* @return The default behavior for outbound traffic.
*
*/
public String outboundPolicy() {
return this.outboundPolicy;
}
/**
* A set of firewall rules that specify what outbound network traffic is allowed.
*
*/
@Import(name="outbounds")
private @Nullable List outbounds;
/**
* @return A set of firewall rules that specify what outbound network traffic is allowed.
*
*/
public Optional> outbounds() {
return Optional.ofNullable(this.outbounds);
}
/**
* The status of the firewall.
*
*/
@Import(name="status", required=true)
private String status;
/**
* @return The status of the firewall.
*
*/
public String status() {
return this.status;
}
/**
* An array of tags applied to this object. Tags are case-insensitive and are for organizational purposes only.
*
*/
@Import(name="tags", required=true)
private List tags;
/**
* @return An array of tags applied to this object. Tags are case-insensitive and are for organizational purposes only.
*
*/
public List tags() {
return this.tags;
}
/**
* When this firewall was last updated.
*
*/
@Import(name="updated", required=true)
private String updated;
/**
* @return When this firewall was last updated.
*
*/
public String updated() {
return this.updated;
}
private GetFirewallsFirewall() {}
private GetFirewallsFirewall(GetFirewallsFirewall $) {
this.created = $.created;
this.devices = $.devices;
this.disabled = $.disabled;
this.id = $.id;
this.inboundPolicy = $.inboundPolicy;
this.inbounds = $.inbounds;
this.label = $.label;
this.linodes = $.linodes;
this.nodebalancers = $.nodebalancers;
this.outboundPolicy = $.outboundPolicy;
this.outbounds = $.outbounds;
this.status = $.status;
this.tags = $.tags;
this.updated = $.updated;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFirewallsFirewall defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetFirewallsFirewall $;
public Builder() {
$ = new GetFirewallsFirewall();
}
public Builder(GetFirewallsFirewall defaults) {
$ = new GetFirewallsFirewall(Objects.requireNonNull(defaults));
}
/**
* @param created When this firewall was created.
*
* @return builder
*
*/
public Builder created(String created) {
$.created = created;
return this;
}
/**
* @param devices The devices associated with this firewall.
*
* @return builder
*
*/
public Builder devices(@Nullable List devices) {
$.devices = devices;
return this;
}
/**
* @param devices The devices associated with this firewall.
*
* @return builder
*
*/
public Builder devices(GetFirewallsFirewallDevice... devices) {
return devices(List.of(devices));
}
/**
* @param disabled If true, the Firewall is inactive.
*
* @return builder
*
*/
public Builder disabled(Boolean disabled) {
$.disabled = disabled;
return this;
}
/**
* @param id The unique ID assigned to this Firewall.
*
* @return builder
*
*/
public Builder id(Integer id) {
$.id = id;
return this;
}
/**
* @param inboundPolicy The default behavior for inbound traffic.
*
* @return builder
*
*/
public Builder inboundPolicy(String inboundPolicy) {
$.inboundPolicy = inboundPolicy;
return this;
}
/**
* @param inbounds A set of firewall rules that specify what inbound network traffic is allowed.
*
* @return builder
*
*/
public Builder inbounds(@Nullable List inbounds) {
$.inbounds = inbounds;
return this;
}
/**
* @param inbounds A set of firewall rules that specify what inbound network traffic is allowed.
*
* @return builder
*
*/
public Builder inbounds(GetFirewallsFirewallInbound... inbounds) {
return inbounds(List.of(inbounds));
}
/**
* @param label The label for the Firewall. For display purposes only. If no label is provided, a default will be assigned.
*
* @return builder
*
*/
public Builder label(String label) {
$.label = label;
return this;
}
/**
* @param linodes The IDs of Linodes this firewall is applied to.
*
* @return builder
*
*/
public Builder linodes(List linodes) {
$.linodes = linodes;
return this;
}
/**
* @param linodes The IDs of Linodes this firewall is applied to.
*
* @return builder
*
*/
public Builder linodes(Integer... linodes) {
return linodes(List.of(linodes));
}
/**
* @param nodebalancers The IDs of NodeBalancers assigned to this Firewall..
*
* @return builder
*
*/
public Builder nodebalancers(List nodebalancers) {
$.nodebalancers = nodebalancers;
return this;
}
/**
* @param nodebalancers The IDs of NodeBalancers assigned to this Firewall..
*
* @return builder
*
*/
public Builder nodebalancers(Integer... nodebalancers) {
return nodebalancers(List.of(nodebalancers));
}
/**
* @param outboundPolicy The default behavior for outbound traffic.
*
* @return builder
*
*/
public Builder outboundPolicy(String outboundPolicy) {
$.outboundPolicy = outboundPolicy;
return this;
}
/**
* @param outbounds A set of firewall rules that specify what outbound network traffic is allowed.
*
* @return builder
*
*/
public Builder outbounds(@Nullable List outbounds) {
$.outbounds = outbounds;
return this;
}
/**
* @param outbounds A set of firewall rules that specify what outbound network traffic is allowed.
*
* @return builder
*
*/
public Builder outbounds(GetFirewallsFirewallOutbound... outbounds) {
return outbounds(List.of(outbounds));
}
/**
* @param status The status of the firewall.
*
* @return builder
*
*/
public Builder status(String status) {
$.status = status;
return this;
}
/**
* @param tags An array of tags applied to this object. Tags are case-insensitive and are for organizational purposes only.
*
* @return builder
*
*/
public Builder tags(List tags) {
$.tags = tags;
return this;
}
/**
* @param tags An array of tags applied to this object. Tags are case-insensitive and are for organizational purposes only.
*
* @return builder
*
*/
public Builder tags(String... tags) {
return tags(List.of(tags));
}
/**
* @param updated When this firewall was last updated.
*
* @return builder
*
*/
public Builder updated(String updated) {
$.updated = updated;
return this;
}
public GetFirewallsFirewall build() {
if ($.created == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "created");
}
if ($.disabled == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "disabled");
}
if ($.id == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "id");
}
if ($.inboundPolicy == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "inboundPolicy");
}
if ($.label == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "label");
}
if ($.linodes == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "linodes");
}
if ($.nodebalancers == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "nodebalancers");
}
if ($.outboundPolicy == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "outboundPolicy");
}
if ($.status == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "status");
}
if ($.tags == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "tags");
}
if ($.updated == null) {
throw new MissingRequiredPropertyException("GetFirewallsFirewall", "updated");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy