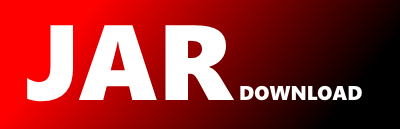
com.pulumi.linode.inputs.UserState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.linode.inputs.UserDomainGrantArgs;
import com.pulumi.linode.inputs.UserFirewallGrantArgs;
import com.pulumi.linode.inputs.UserGlobalGrantsArgs;
import com.pulumi.linode.inputs.UserImageGrantArgs;
import com.pulumi.linode.inputs.UserLinodeGrantArgs;
import com.pulumi.linode.inputs.UserLongviewGrantArgs;
import com.pulumi.linode.inputs.UserNodebalancerGrantArgs;
import com.pulumi.linode.inputs.UserStackscriptGrantArgs;
import com.pulumi.linode.inputs.UserVolumeGrantArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserState extends com.pulumi.resources.ResourceArgs {
public static final UserState Empty = new UserState();
/**
* The domains the user has permissions access to.
*
*/
@Import(name="domainGrants")
private @Nullable Output> domainGrants;
/**
* @return The domains the user has permissions access to.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy