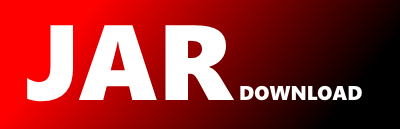
com.pulumi.linode.outputs.GetIpv6RangeResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetIpv6RangeResult {
private String id;
private Boolean isBgp;
/**
* @return A set of Linodes targeted by this IPv6 range. Includes Linodes with IP sharing.
*
*/
private List linodes;
/**
* @return The prefix length of the address, denoting how many addresses can be assigned from this range.
*
*/
private Integer prefix;
private String range;
/**
* @return The region for this range of IPv6 addresses.
*
*/
private String region;
private GetIpv6RangeResult() {}
public String id() {
return this.id;
}
public Boolean isBgp() {
return this.isBgp;
}
/**
* @return A set of Linodes targeted by this IPv6 range. Includes Linodes with IP sharing.
*
*/
public List linodes() {
return this.linodes;
}
/**
* @return The prefix length of the address, denoting how many addresses can be assigned from this range.
*
*/
public Integer prefix() {
return this.prefix;
}
public String range() {
return this.range;
}
/**
* @return The region for this range of IPv6 addresses.
*
*/
public String region() {
return this.region;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetIpv6RangeResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String id;
private Boolean isBgp;
private List linodes;
private Integer prefix;
private String range;
private String region;
public Builder() {}
public Builder(GetIpv6RangeResult defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.isBgp = defaults.isBgp;
this.linodes = defaults.linodes;
this.prefix = defaults.prefix;
this.range = defaults.range;
this.region = defaults.region;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isBgp(Boolean isBgp) {
if (isBgp == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "isBgp");
}
this.isBgp = isBgp;
return this;
}
@CustomType.Setter
public Builder linodes(List linodes) {
if (linodes == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "linodes");
}
this.linodes = linodes;
return this;
}
public Builder linodes(Integer... linodes) {
return linodes(List.of(linodes));
}
@CustomType.Setter
public Builder prefix(Integer prefix) {
if (prefix == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "prefix");
}
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder range(String range) {
if (range == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "range");
}
this.range = range;
return this;
}
@CustomType.Setter
public Builder region(String region) {
if (region == null) {
throw new MissingRequiredPropertyException("GetIpv6RangeResult", "region");
}
this.region = region;
return this;
}
public GetIpv6RangeResult build() {
final var _resultValue = new GetIpv6RangeResult();
_resultValue.id = id;
_resultValue.isBgp = isBgp;
_resultValue.linodes = linodes;
_resultValue.prefix = prefix;
_resultValue.range = range;
_resultValue.region = region;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy