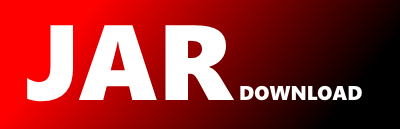
com.pulumi.linode.outputs.GetVpcIpsVpcIp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetVpcIpsVpcIp {
/**
* @return True if the VPC interface is in use, meaning that the Linode was powered on using the config_id to which the interface belongs. Otherwise false.
*
*/
private Boolean active;
/**
* @return An IPv4 address configured for this VPC interface. These follow the RFC 1918 private address format. Null if an address_range.
*
*/
private String address;
/**
* @return A range of IPv4 addresses configured for this VPC interface. Null if a single address.
*
*/
private String addressRange;
/**
* @return The globally general entity identifier for the Linode configuration profile where the VPC is included.
*
*/
private Integer configId;
/**
* @return The default gateway for the VPC subnet that the IP or IP range belongs to.
*
*/
private String gateway;
/**
* @return The globally general API entity identifier for the Linode interface.
*
*/
private Integer interfaceId;
/**
* @return The identifier for the Linode the VPC interface currently belongs to.
*
*/
private Integer linodeId;
/**
* @return The public IP address used for NAT 1:1 with the VPC. This is empty if NAT 1:1 isn't used.
*
*/
private String nat11;
/**
* @return The number of bits set in the subnet mask.
*
*/
private Integer prefix;
/**
* @return The region of the VPC.
*
*/
private String region;
/**
* @return The id of the VPC Subnet for this interface.
*
*/
private Integer subnetId;
/**
* @return The mask that separates host bits from network bits for the address or address_range.
*
*/
private String subnetMask;
/**
* @return The id of the parent VPC for the list of VPC IPs.
*
* * `filter` - (Optional) A set of filters used to select Linode VPC IPs that meet certain requirements.
*
*/
private Integer vpcId;
private GetVpcIpsVpcIp() {}
/**
* @return True if the VPC interface is in use, meaning that the Linode was powered on using the config_id to which the interface belongs. Otherwise false.
*
*/
public Boolean active() {
return this.active;
}
/**
* @return An IPv4 address configured for this VPC interface. These follow the RFC 1918 private address format. Null if an address_range.
*
*/
public String address() {
return this.address;
}
/**
* @return A range of IPv4 addresses configured for this VPC interface. Null if a single address.
*
*/
public String addressRange() {
return this.addressRange;
}
/**
* @return The globally general entity identifier for the Linode configuration profile where the VPC is included.
*
*/
public Integer configId() {
return this.configId;
}
/**
* @return The default gateway for the VPC subnet that the IP or IP range belongs to.
*
*/
public String gateway() {
return this.gateway;
}
/**
* @return The globally general API entity identifier for the Linode interface.
*
*/
public Integer interfaceId() {
return this.interfaceId;
}
/**
* @return The identifier for the Linode the VPC interface currently belongs to.
*
*/
public Integer linodeId() {
return this.linodeId;
}
/**
* @return The public IP address used for NAT 1:1 with the VPC. This is empty if NAT 1:1 isn't used.
*
*/
public String nat11() {
return this.nat11;
}
/**
* @return The number of bits set in the subnet mask.
*
*/
public Integer prefix() {
return this.prefix;
}
/**
* @return The region of the VPC.
*
*/
public String region() {
return this.region;
}
/**
* @return The id of the VPC Subnet for this interface.
*
*/
public Integer subnetId() {
return this.subnetId;
}
/**
* @return The mask that separates host bits from network bits for the address or address_range.
*
*/
public String subnetMask() {
return this.subnetMask;
}
/**
* @return The id of the parent VPC for the list of VPC IPs.
*
* * `filter` - (Optional) A set of filters used to select Linode VPC IPs that meet certain requirements.
*
*/
public Integer vpcId() {
return this.vpcId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVpcIpsVpcIp defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean active;
private String address;
private String addressRange;
private Integer configId;
private String gateway;
private Integer interfaceId;
private Integer linodeId;
private String nat11;
private Integer prefix;
private String region;
private Integer subnetId;
private String subnetMask;
private Integer vpcId;
public Builder() {}
public Builder(GetVpcIpsVpcIp defaults) {
Objects.requireNonNull(defaults);
this.active = defaults.active;
this.address = defaults.address;
this.addressRange = defaults.addressRange;
this.configId = defaults.configId;
this.gateway = defaults.gateway;
this.interfaceId = defaults.interfaceId;
this.linodeId = defaults.linodeId;
this.nat11 = defaults.nat11;
this.prefix = defaults.prefix;
this.region = defaults.region;
this.subnetId = defaults.subnetId;
this.subnetMask = defaults.subnetMask;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder active(Boolean active) {
if (active == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "active");
}
this.active = active;
return this;
}
@CustomType.Setter
public Builder address(String address) {
if (address == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "address");
}
this.address = address;
return this;
}
@CustomType.Setter
public Builder addressRange(String addressRange) {
if (addressRange == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "addressRange");
}
this.addressRange = addressRange;
return this;
}
@CustomType.Setter
public Builder configId(Integer configId) {
if (configId == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "configId");
}
this.configId = configId;
return this;
}
@CustomType.Setter
public Builder gateway(String gateway) {
if (gateway == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "gateway");
}
this.gateway = gateway;
return this;
}
@CustomType.Setter
public Builder interfaceId(Integer interfaceId) {
if (interfaceId == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "interfaceId");
}
this.interfaceId = interfaceId;
return this;
}
@CustomType.Setter
public Builder linodeId(Integer linodeId) {
if (linodeId == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "linodeId");
}
this.linodeId = linodeId;
return this;
}
@CustomType.Setter
public Builder nat11(String nat11) {
if (nat11 == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "nat11");
}
this.nat11 = nat11;
return this;
}
@CustomType.Setter
public Builder prefix(Integer prefix) {
if (prefix == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "prefix");
}
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder region(String region) {
if (region == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "region");
}
this.region = region;
return this;
}
@CustomType.Setter
public Builder subnetId(Integer subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "subnetId");
}
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder subnetMask(String subnetMask) {
if (subnetMask == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "subnetMask");
}
this.subnetMask = subnetMask;
return this;
}
@CustomType.Setter
public Builder vpcId(Integer vpcId) {
if (vpcId == null) {
throw new MissingRequiredPropertyException("GetVpcIpsVpcIp", "vpcId");
}
this.vpcId = vpcId;
return this;
}
public GetVpcIpsVpcIp build() {
final var _resultValue = new GetVpcIpsVpcIp();
_resultValue.active = active;
_resultValue.address = address;
_resultValue.addressRange = addressRange;
_resultValue.configId = configId;
_resultValue.gateway = gateway;
_resultValue.interfaceId = interfaceId;
_resultValue.linodeId = linodeId;
_resultValue.nat11 = nat11;
_resultValue.prefix = prefix;
_resultValue.region = region;
_resultValue.subnetId = subnetId;
_resultValue.subnetMask = subnetMask;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy