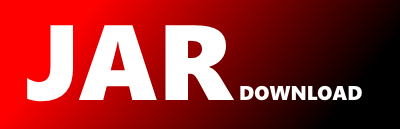
com.pulumi.linode.outputs.NodeBalancerFirewallOutbound Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linode Show documentation
Show all versions of linode Show documentation
A Pulumi package for creating and managing linode cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.linode.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class NodeBalancerFirewallOutbound {
/**
* @return Controls whether traffic is accepted or dropped by this rule. Overrides the Firewall’s inbound_policy if this is an inbound rule, or the outbound_policy if this is an outbound rule.
*
*/
private String action;
private String description;
/**
* @return A list of IPv4 addresses or networks. Must be in IP/mask format.
*
*/
private List ipv4s;
/**
* @return A list of IPv6 addresses or networks. Must be in IP/mask format.
*
*/
private List ipv6s;
/**
* @return The label of the Linode NodeBalancer
*
*/
private String label;
/**
* @return A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
*/
private String ports;
/**
* @return The network protocol this rule controls. (`TCP`, `UDP`, `ICMP`)
*
*/
private String protocol;
private NodeBalancerFirewallOutbound() {}
/**
* @return Controls whether traffic is accepted or dropped by this rule. Overrides the Firewall’s inbound_policy if this is an inbound rule, or the outbound_policy if this is an outbound rule.
*
*/
public String action() {
return this.action;
}
public String description() {
return this.description;
}
/**
* @return A list of IPv4 addresses or networks. Must be in IP/mask format.
*
*/
public List ipv4s() {
return this.ipv4s;
}
/**
* @return A list of IPv6 addresses or networks. Must be in IP/mask format.
*
*/
public List ipv6s() {
return this.ipv6s;
}
/**
* @return The label of the Linode NodeBalancer
*
*/
public String label() {
return this.label;
}
/**
* @return A string representation of ports and/or port ranges (i.e. "443" or "80-90, 91").
*
*/
public String ports() {
return this.ports;
}
/**
* @return The network protocol this rule controls. (`TCP`, `UDP`, `ICMP`)
*
*/
public String protocol() {
return this.protocol;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NodeBalancerFirewallOutbound defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String action;
private String description;
private List ipv4s;
private List ipv6s;
private String label;
private String ports;
private String protocol;
public Builder() {}
public Builder(NodeBalancerFirewallOutbound defaults) {
Objects.requireNonNull(defaults);
this.action = defaults.action;
this.description = defaults.description;
this.ipv4s = defaults.ipv4s;
this.ipv6s = defaults.ipv6s;
this.label = defaults.label;
this.ports = defaults.ports;
this.protocol = defaults.protocol;
}
@CustomType.Setter
public Builder action(String action) {
if (action == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "action");
}
this.action = action;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder ipv4s(List ipv4s) {
if (ipv4s == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "ipv4s");
}
this.ipv4s = ipv4s;
return this;
}
public Builder ipv4s(String... ipv4s) {
return ipv4s(List.of(ipv4s));
}
@CustomType.Setter
public Builder ipv6s(List ipv6s) {
if (ipv6s == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "ipv6s");
}
this.ipv6s = ipv6s;
return this;
}
public Builder ipv6s(String... ipv6s) {
return ipv6s(List.of(ipv6s));
}
@CustomType.Setter
public Builder label(String label) {
if (label == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "label");
}
this.label = label;
return this;
}
@CustomType.Setter
public Builder ports(String ports) {
if (ports == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "ports");
}
this.ports = ports;
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("NodeBalancerFirewallOutbound", "protocol");
}
this.protocol = protocol;
return this;
}
public NodeBalancerFirewallOutbound build() {
final var _resultValue = new NodeBalancerFirewallOutbound();
_resultValue.action = action;
_resultValue.description = description;
_resultValue.ipv4s = ipv4s;
_resultValue.ipv6s = ipv6s;
_resultValue.label = label;
_resultValue.ports = ports;
_resultValue.protocol = protocol;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy