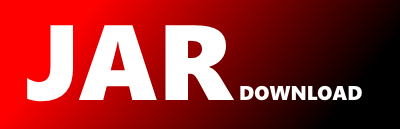
com.pulumi.mongodbatlas.GlobalClusterConfig Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.mongodbatlas.GlobalClusterConfigArgs;
import com.pulumi.mongodbatlas.Utilities;
import com.pulumi.mongodbatlas.inputs.GlobalClusterConfigState;
import com.pulumi.mongodbatlas.outputs.GlobalClusterConfigCustomZoneMapping;
import com.pulumi.mongodbatlas.outputs.GlobalClusterConfigManagedNamespace;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## # Resource: mongodbatlas.GlobalClusterConfig
*
* `mongodbatlas.GlobalClusterConfig` provides a Global Cluster Configuration resource.
*
* > **NOTE:** Groups and projects are synonymous terms. You may find group_id in the official documentation.
*
* > **NOTE:** This resource can only be used with Atlas-managed clusters. See doc for `global_cluster_self_managed_sharding` attribute in `mongodbatlas.AdvancedCluster` resource for more info.
*
* > **IMPORTANT:** A Global Cluster Configuration, once created, can only be deleted. You can recreate the Global Cluster with the same data only in the Atlas UI. This is because the configuration and its related collection with shard key and indexes are managed separately and they would end up in an inconsistent state. [Read more about Global Cluster Configuration](https://www.mongodb.com/docs/atlas/global-clusters/)
*
* ## Examples Usage
*
* ### Example Global cluster
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.mongodbatlas.AdvancedCluster;
* import com.pulumi.mongodbatlas.AdvancedClusterArgs;
* import com.pulumi.mongodbatlas.inputs.AdvancedClusterReplicationSpecArgs;
* import com.pulumi.mongodbatlas.GlobalClusterConfig;
* import com.pulumi.mongodbatlas.GlobalClusterConfigArgs;
* import com.pulumi.mongodbatlas.inputs.GlobalClusterConfigManagedNamespaceArgs;
* import com.pulumi.mongodbatlas.inputs.GlobalClusterConfigCustomZoneMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new AdvancedCluster("test", AdvancedClusterArgs.builder()
* .projectId("")
* .name("")
* .clusterType("GEOSHARDED")
* .backupEnabled(true)
* .replicationSpecs(
* AdvancedClusterReplicationSpecArgs.builder()
* .zoneName("Zone 1")
* .regionConfigs(AdvancedClusterReplicationSpecRegionConfigArgs.builder()
* .electableSpecs(AdvancedClusterReplicationSpecRegionConfigElectableSpecsArgs.builder()
* .instanceSize("M30")
* .nodeCount(3)
* .build())
* .providerName("AWS")
* .priority(7)
* .regionName("EU_CENTRAL_1")
* .build())
* .build(),
* AdvancedClusterReplicationSpecArgs.builder()
* .zoneName("Zone 2")
* .regionConfigs(AdvancedClusterReplicationSpecRegionConfigArgs.builder()
* .electableSpecs(AdvancedClusterReplicationSpecRegionConfigElectableSpecsArgs.builder()
* .instanceSize("M30")
* .nodeCount(3)
* .build())
* .providerName("AWS")
* .priority(7)
* .regionName("US_EAST_2")
* .build())
* .build())
* .build());
*
* var config = new GlobalClusterConfig("config", GlobalClusterConfigArgs.builder()
* .projectId(test.projectId())
* .clusterName(test.name())
* .managedNamespaces(GlobalClusterConfigManagedNamespaceArgs.builder()
* .db("mydata")
* .collection("publishers")
* .customShardKey("city")
* .isCustomShardKeyHashed(false)
* .isShardKeyUnique(false)
* .build())
* .customZoneMappings(GlobalClusterConfigCustomZoneMappingArgs.builder()
* .location("CA")
* .zone("Zone 1")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Global Clusters can be imported using project ID and cluster name, in the format `PROJECTID-CLUSTER_NAME`, e.g.
*
* ```sh
* $ pulumi import mongodbatlas:index/globalClusterConfig:GlobalClusterConfig config 1112222b3bf99403840e8934-Cluster0
* ```
* See detailed information for arguments and attributes: [MongoDB API Global Clusters](https://docs.atlas.mongodb.com/reference/api/global-clusters/)
*
*/
@ResourceType(type="mongodbatlas:index/globalClusterConfig:GlobalClusterConfig")
public class GlobalClusterConfig extends com.pulumi.resources.CustomResource {
/**
* The name of the Global Cluster.
*
*/
@Export(name="clusterName", refs={String.class}, tree="[0]")
private Output clusterName;
/**
* @return The name of the Global Cluster.
*
*/
public Output clusterName() {
return this.clusterName;
}
/**
* A map of all custom zone mappings defined for the Global Cluster. Atlas automatically maps each location code to the closest geographical zone. Custom zone mappings allow administrators to override these automatic mappings. If your Global Cluster does not have any custom zone mappings, this document is empty.
*
*/
@Export(name="customZoneMapping", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy