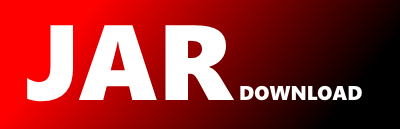
com.pulumi.mongodbatlas.OnlineArchiveArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.mongodbatlas.inputs.OnlineArchiveCriteriaArgs;
import com.pulumi.mongodbatlas.inputs.OnlineArchiveDataExpirationRuleArgs;
import com.pulumi.mongodbatlas.inputs.OnlineArchiveDataProcessRegionArgs;
import com.pulumi.mongodbatlas.inputs.OnlineArchivePartitionFieldArgs;
import com.pulumi.mongodbatlas.inputs.OnlineArchiveScheduleArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OnlineArchiveArgs extends com.pulumi.resources.ResourceArgs {
public static final OnlineArchiveArgs Empty = new OnlineArchiveArgs();
/**
* Name of the cluster that contains the collection.
*
*/
@Import(name="clusterName", required=true)
private Output clusterName;
/**
* @return Name of the cluster that contains the collection.
*
*/
public Output clusterName() {
return this.clusterName;
}
/**
* Name of the collection.
*
*/
@Import(name="collName", required=true)
private Output collName;
/**
* @return Name of the collection.
*
*/
public Output collName() {
return this.collName;
}
/**
* Type of MongoDB collection that you want to return. This value can be "TIMESERIES" or "STANDARD". Default is "STANDARD".
*
*/
@Import(name="collectionType")
private @Nullable Output collectionType;
/**
* @return Type of MongoDB collection that you want to return. This value can be "TIMESERIES" or "STANDARD". Default is "STANDARD".
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy