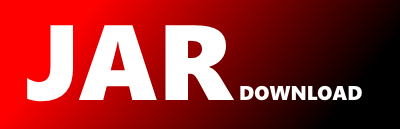
com.pulumi.mongodbatlas.OrganizationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OrganizationArgs extends com.pulumi.resources.ResourceArgs {
public static final OrganizationArgs Empty = new OrganizationArgs();
/**
* Flag that indicates whether to require API operations to originate from an IP Address added to the API access list for the specified organization.
*
*/
@Import(name="apiAccessListRequired")
private @Nullable Output apiAccessListRequired;
/**
* @return Flag that indicates whether to require API operations to originate from an IP Address added to the API access list for the specified organization.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy