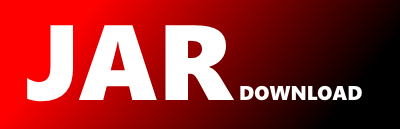
com.pulumi.mongodbatlas.StreamProcessorArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.mongodbatlas.inputs.StreamProcessorOptionsArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StreamProcessorArgs extends com.pulumi.resources.ResourceArgs {
public static final StreamProcessorArgs Empty = new StreamProcessorArgs();
/**
* Human-readable label that identifies the stream instance.
*
*/
@Import(name="instanceName", required=true)
private Output instanceName;
/**
* @return Human-readable label that identifies the stream instance.
*
*/
public Output instanceName() {
return this.instanceName;
}
/**
* Optional configuration for the stream processor.
*
*/
@Import(name="options")
private @Nullable Output options;
/**
* @return Optional configuration for the stream processor.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy