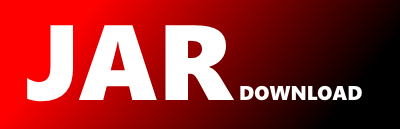
com.pulumi.mongodbatlas.inputs.FederatedSettingsOrgConfigState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.mongodbatlas.inputs.FederatedSettingsOrgConfigUserConflictArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FederatedSettingsOrgConfigState extends com.pulumi.resources.ResourceArgs {
public static final FederatedSettingsOrgConfigState Empty = new FederatedSettingsOrgConfigState();
/**
* The collection of unique ids representing the identity providers that can be used for data access in this organization.
*
*/
@Import(name="dataAccessIdentityProviderIds")
private @Nullable Output> dataAccessIdentityProviderIds;
/**
* @return The collection of unique ids representing the identity providers that can be used for data access in this organization.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy