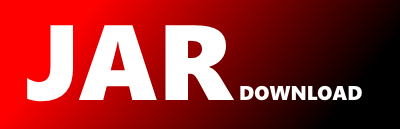
com.pulumi.mongodbatlas.inputs.PrivateLinkEndpointServiceState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.mongodbatlas.inputs.PrivateLinkEndpointServiceEndpointArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PrivateLinkEndpointServiceState extends com.pulumi.resources.ResourceArgs {
public static final PrivateLinkEndpointServiceState Empty = new PrivateLinkEndpointServiceState();
/**
* Status of the interface endpoint for AWS.
* Returns one of the following values:
* * `NONE` - Atlas created the network load balancer and VPC endpoint service, but AWS hasn’t yet created the VPC endpoint.
* * `PENDING_ACCEPTANCE` - AWS has received the connection request from your VPC endpoint to the Atlas VPC endpoint service.
* * `PENDING` - AWS is establishing the connection between your VPC endpoint and the Atlas VPC endpoint service.
* * `AVAILABLE` - Atlas VPC resources are connected to the VPC endpoint in your VPC. You can connect to Atlas clusters in this region using AWS PrivateLink.
* * `REJECTED` - AWS failed to establish a connection between Atlas VPC resources to the VPC endpoint in your VPC.
* * `DELETING` - Atlas is removing the interface endpoint from the private endpoint connection.
*
*/
@Import(name="awsConnectionStatus")
private @Nullable Output awsConnectionStatus;
/**
* @return Status of the interface endpoint for AWS.
* Returns one of the following values:
* * `NONE` - Atlas created the network load balancer and VPC endpoint service, but AWS hasn’t yet created the VPC endpoint.
* * `PENDING_ACCEPTANCE` - AWS has received the connection request from your VPC endpoint to the Atlas VPC endpoint service.
* * `PENDING` - AWS is establishing the connection between your VPC endpoint and the Atlas VPC endpoint service.
* * `AVAILABLE` - Atlas VPC resources are connected to the VPC endpoint in your VPC. You can connect to Atlas clusters in this region using AWS PrivateLink.
* * `REJECTED` - AWS failed to establish a connection between Atlas VPC resources to the VPC endpoint in your VPC.
* * `DELETING` - Atlas is removing the interface endpoint from the private endpoint connection.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy