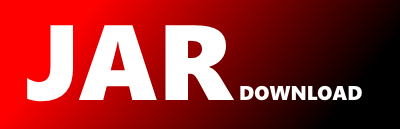
com.pulumi.mongodbatlas.outputs.GetClusterResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.mongodbatlas.outputs.GetClusterAdvancedConfiguration;
import com.pulumi.mongodbatlas.outputs.GetClusterBiConnectorConfig;
import com.pulumi.mongodbatlas.outputs.GetClusterConnectionString;
import com.pulumi.mongodbatlas.outputs.GetClusterLabel;
import com.pulumi.mongodbatlas.outputs.GetClusterReplicationSpec;
import com.pulumi.mongodbatlas.outputs.GetClusterSnapshotBackupPolicy;
import com.pulumi.mongodbatlas.outputs.GetClusterTag;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetClusterResult {
/**
* @return Get the advanced configuration options. See Advanced Configuration below for more details.
*
*/
private List advancedConfigurations;
/**
* @return Specifies whether cluster tier auto-scaling is enabled. The default is false.
*
*/
private Boolean autoScalingComputeEnabled;
/**
* @return Specifies whether cluster tier auto-down-scaling is enabled.
*
*/
private Boolean autoScalingComputeScaleDownEnabled;
/**
* @return Indicates whether disk auto-scaling is enabled.
*
*/
private Boolean autoScalingDiskGbEnabled;
/**
* @return Indicates Cloud service provider on which the server for a multi-tenant cluster is provisioned.
*
*/
private String backingProviderName;
/**
* @return Legacy Option, Indicates whether Atlas continuous backups are enabled for the cluster.
*
*/
private Boolean backupEnabled;
/**
* @return Indicates BI Connector for Atlas configuration on this cluster. BI Connector for Atlas is only available for M10+ clusters. See BI Connector below for more details.
*
*/
private List biConnectorConfigs;
/**
* @return Indicates the type of the cluster that you want to modify. You cannot convert a sharded cluster deployment to a replica set deployment.
*
*/
private String clusterType;
/**
* @return Set of connection strings that your applications use to connect to this cluster. More info in [Connection-strings](https://docs.mongodb.com/manual/reference/connection-string/). Use the parameters in this object to connect your applications to this cluster. To learn more about the formats of connection strings, see [Connection String Options](https://docs.atlas.mongodb.com/reference/faq/connection-changes/).
*
*/
private List connectionStrings;
/**
* @return The Network Peering Container ID.
*
*/
private String containerId;
/**
* @return Indicates the size in gigabytes of the server’s root volume (AWS/GCP Only).
*
*/
private Double diskSizeGb;
/**
* @return Indicates whether Encryption at Rest is enabled or disabled.
*
*/
private String encryptionAtRestProvider;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Set that contains key-value pairs between 1 to 255 characters in length for tagging and categorizing the cluster. See below. **DEPRECATED** Use `tags` instead.
*
* @deprecated
* This parameter is deprecated and will be removed in the future. Please transition to tags
*
*/
@Deprecated /* This parameter is deprecated and will be removed in the future. Please transition to tags */
private List labels;
/**
* @return Indicates the version of the cluster to deploy.
*
*/
private String mongoDbMajorVersion;
/**
* @return Version of MongoDB the cluster runs, in `major-version`.`minor-version` format.
*
*/
private String mongoDbVersion;
/**
* @return Base connection string for the cluster. Atlas only displays this field after the cluster is operational, not while it builds the cluster.
*
*/
private String mongoUri;
/**
* @return Lists when the connection string was last updated. The connection string changes, for example, if you change a replica set to a sharded cluster.
*
*/
private String mongoUriUpdated;
/**
* @return Describes connection string for connecting to the Atlas cluster. Includes the replicaSet, ssl, and authSource query parameters in the connection string with values appropriate for the cluster.
*
*/
private String mongoUriWithOptions;
/**
* @return The name of the current plugin
*
*/
private String name;
/**
* @return Number of shards to deploy in the specified zone.
*
*/
private Integer numShards;
/**
* @return Flag that indicates whether the cluster is paused or not.
*
*/
private Boolean paused;
/**
* @return Flag that indicates if the cluster uses Continuous Cloud Backup.
*
*/
private Boolean pitEnabled;
private String projectId;
/**
* @return Maximum instance size to which your cluster can automatically scale.
*
*/
private String providerAutoScalingComputeMaxInstanceSize;
/**
* @return Minimum instance size to which your cluster can automatically scale.
*
*/
private String providerAutoScalingComputeMinInstanceSize;
private Boolean providerBackupEnabled;
/**
* @return Indicates the maximum input/output operations per second (IOPS) the system can perform. The possible values depend on the selected providerSettings.instanceSizeName and diskSizeGB.
*
*/
private Integer providerDiskIops;
/**
* @return Describes Azure disk type of the server’s root volume (Azure Only).
*
*/
private String providerDiskTypeName;
/**
* @return **(DEPRECATED)** Indicates whether the Amazon EBS encryption is enabled. This feature encrypts the server’s root volume for both data at rest within the volume and data moving between the volume and the instance. By default this attribute is always enabled, per deprecation process showing the real value at `provider_encrypt_ebs_volume_flag` computed attribute.
*
*/
private Boolean providerEncryptEbsVolume;
private Boolean providerEncryptEbsVolumeFlag;
/**
* @return Atlas provides different instance sizes, each with a default storage capacity and RAM size.
*
*/
private String providerInstanceSizeName;
/**
* @return Indicates the cloud service provider on which the servers are provisioned.
*
*/
private String providerName;
/**
* @return Indicates Physical location of your MongoDB cluster. The region you choose can affect network latency for clients accessing your databases. Requires the Atlas Region name, see the reference list for [AWS](https://docs.atlas.mongodb.com/reference/amazon-aws/), [GCP](https://docs.atlas.mongodb.com/reference/google-gcp/), [Azure](https://docs.atlas.mongodb.com/reference/microsoft-azure/).
*
*/
private String providerRegionName;
/**
* @return Indicates the type of the volume. The possible values are: `STANDARD` and `PROVISIONED`.
* > **NOTE:** `STANDARD` is not available for NVME clusters.
*
*/
private String providerVolumeType;
/**
* @return (Deprecated) Number of replica set members. Each member keeps a copy of your databases, providing high availability and data redundancy. The possible values are 3, 5, or 7. The default value is 3.
*
*/
private Integer replicationFactor;
/**
* @return Configuration for cluster regions. See Replication Spec below for more details.
*
*/
private List replicationSpecs;
/**
* @return current snapshot schedule and retention settings for the cluster.
*
*/
private List snapshotBackupPolicies;
/**
* @return Connection string for connecting to the Atlas cluster. The +srv modifier forces the connection to use TLS/SSL. See the mongoURI for additional options.
*
*/
private String srvAddress;
/**
* @return Indicates the current state of the cluster. The possible states are:
* - IDLE
* - CREATING
* - UPDATING
* - DELETING
* - DELETED
* - REPAIRING
*
*/
private String stateName;
/**
* @return Set that contains key-value pairs between 1 to 255 characters in length for tagging and categorizing the cluster. See below.
*
*/
private List tags;
/**
* @return Flag that indicates whether termination protection is enabled on the cluster. If set to true, MongoDB Cloud won't delete the cluster. If set to false, MongoDB Cloud will delete the cluster.
*
*/
private Boolean terminationProtectionEnabled;
/**
* @return Release cadence that Atlas uses for this cluster.
*
*/
private String versionReleaseSystem;
private GetClusterResult() {}
/**
* @return Get the advanced configuration options. See Advanced Configuration below for more details.
*
*/
public List advancedConfigurations() {
return this.advancedConfigurations;
}
/**
* @return Specifies whether cluster tier auto-scaling is enabled. The default is false.
*
*/
public Boolean autoScalingComputeEnabled() {
return this.autoScalingComputeEnabled;
}
/**
* @return Specifies whether cluster tier auto-down-scaling is enabled.
*
*/
public Boolean autoScalingComputeScaleDownEnabled() {
return this.autoScalingComputeScaleDownEnabled;
}
/**
* @return Indicates whether disk auto-scaling is enabled.
*
*/
public Boolean autoScalingDiskGbEnabled() {
return this.autoScalingDiskGbEnabled;
}
/**
* @return Indicates Cloud service provider on which the server for a multi-tenant cluster is provisioned.
*
*/
public String backingProviderName() {
return this.backingProviderName;
}
/**
* @return Legacy Option, Indicates whether Atlas continuous backups are enabled for the cluster.
*
*/
public Boolean backupEnabled() {
return this.backupEnabled;
}
/**
* @return Indicates BI Connector for Atlas configuration on this cluster. BI Connector for Atlas is only available for M10+ clusters. See BI Connector below for more details.
*
*/
public List biConnectorConfigs() {
return this.biConnectorConfigs;
}
/**
* @return Indicates the type of the cluster that you want to modify. You cannot convert a sharded cluster deployment to a replica set deployment.
*
*/
public String clusterType() {
return this.clusterType;
}
/**
* @return Set of connection strings that your applications use to connect to this cluster. More info in [Connection-strings](https://docs.mongodb.com/manual/reference/connection-string/). Use the parameters in this object to connect your applications to this cluster. To learn more about the formats of connection strings, see [Connection String Options](https://docs.atlas.mongodb.com/reference/faq/connection-changes/).
*
*/
public List connectionStrings() {
return this.connectionStrings;
}
/**
* @return The Network Peering Container ID.
*
*/
public String containerId() {
return this.containerId;
}
/**
* @return Indicates the size in gigabytes of the server’s root volume (AWS/GCP Only).
*
*/
public Double diskSizeGb() {
return this.diskSizeGb;
}
/**
* @return Indicates whether Encryption at Rest is enabled or disabled.
*
*/
public String encryptionAtRestProvider() {
return this.encryptionAtRestProvider;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Set that contains key-value pairs between 1 to 255 characters in length for tagging and categorizing the cluster. See below. **DEPRECATED** Use `tags` instead.
*
* @deprecated
* This parameter is deprecated and will be removed in the future. Please transition to tags
*
*/
@Deprecated /* This parameter is deprecated and will be removed in the future. Please transition to tags */
public List labels() {
return this.labels;
}
/**
* @return Indicates the version of the cluster to deploy.
*
*/
public String mongoDbMajorVersion() {
return this.mongoDbMajorVersion;
}
/**
* @return Version of MongoDB the cluster runs, in `major-version`.`minor-version` format.
*
*/
public String mongoDbVersion() {
return this.mongoDbVersion;
}
/**
* @return Base connection string for the cluster. Atlas only displays this field after the cluster is operational, not while it builds the cluster.
*
*/
public String mongoUri() {
return this.mongoUri;
}
/**
* @return Lists when the connection string was last updated. The connection string changes, for example, if you change a replica set to a sharded cluster.
*
*/
public String mongoUriUpdated() {
return this.mongoUriUpdated;
}
/**
* @return Describes connection string for connecting to the Atlas cluster. Includes the replicaSet, ssl, and authSource query parameters in the connection string with values appropriate for the cluster.
*
*/
public String mongoUriWithOptions() {
return this.mongoUriWithOptions;
}
/**
* @return The name of the current plugin
*
*/
public String name() {
return this.name;
}
/**
* @return Number of shards to deploy in the specified zone.
*
*/
public Integer numShards() {
return this.numShards;
}
/**
* @return Flag that indicates whether the cluster is paused or not.
*
*/
public Boolean paused() {
return this.paused;
}
/**
* @return Flag that indicates if the cluster uses Continuous Cloud Backup.
*
*/
public Boolean pitEnabled() {
return this.pitEnabled;
}
public String projectId() {
return this.projectId;
}
/**
* @return Maximum instance size to which your cluster can automatically scale.
*
*/
public String providerAutoScalingComputeMaxInstanceSize() {
return this.providerAutoScalingComputeMaxInstanceSize;
}
/**
* @return Minimum instance size to which your cluster can automatically scale.
*
*/
public String providerAutoScalingComputeMinInstanceSize() {
return this.providerAutoScalingComputeMinInstanceSize;
}
public Boolean providerBackupEnabled() {
return this.providerBackupEnabled;
}
/**
* @return Indicates the maximum input/output operations per second (IOPS) the system can perform. The possible values depend on the selected providerSettings.instanceSizeName and diskSizeGB.
*
*/
public Integer providerDiskIops() {
return this.providerDiskIops;
}
/**
* @return Describes Azure disk type of the server’s root volume (Azure Only).
*
*/
public String providerDiskTypeName() {
return this.providerDiskTypeName;
}
/**
* @return **(DEPRECATED)** Indicates whether the Amazon EBS encryption is enabled. This feature encrypts the server’s root volume for both data at rest within the volume and data moving between the volume and the instance. By default this attribute is always enabled, per deprecation process showing the real value at `provider_encrypt_ebs_volume_flag` computed attribute.
*
*/
public Boolean providerEncryptEbsVolume() {
return this.providerEncryptEbsVolume;
}
public Boolean providerEncryptEbsVolumeFlag() {
return this.providerEncryptEbsVolumeFlag;
}
/**
* @return Atlas provides different instance sizes, each with a default storage capacity and RAM size.
*
*/
public String providerInstanceSizeName() {
return this.providerInstanceSizeName;
}
/**
* @return Indicates the cloud service provider on which the servers are provisioned.
*
*/
public String providerName() {
return this.providerName;
}
/**
* @return Indicates Physical location of your MongoDB cluster. The region you choose can affect network latency for clients accessing your databases. Requires the Atlas Region name, see the reference list for [AWS](https://docs.atlas.mongodb.com/reference/amazon-aws/), [GCP](https://docs.atlas.mongodb.com/reference/google-gcp/), [Azure](https://docs.atlas.mongodb.com/reference/microsoft-azure/).
*
*/
public String providerRegionName() {
return this.providerRegionName;
}
/**
* @return Indicates the type of the volume. The possible values are: `STANDARD` and `PROVISIONED`.
* > **NOTE:** `STANDARD` is not available for NVME clusters.
*
*/
public String providerVolumeType() {
return this.providerVolumeType;
}
/**
* @return (Deprecated) Number of replica set members. Each member keeps a copy of your databases, providing high availability and data redundancy. The possible values are 3, 5, or 7. The default value is 3.
*
*/
public Integer replicationFactor() {
return this.replicationFactor;
}
/**
* @return Configuration for cluster regions. See Replication Spec below for more details.
*
*/
public List replicationSpecs() {
return this.replicationSpecs;
}
/**
* @return current snapshot schedule and retention settings for the cluster.
*
*/
public List snapshotBackupPolicies() {
return this.snapshotBackupPolicies;
}
/**
* @return Connection string for connecting to the Atlas cluster. The +srv modifier forces the connection to use TLS/SSL. See the mongoURI for additional options.
*
*/
public String srvAddress() {
return this.srvAddress;
}
/**
* @return Indicates the current state of the cluster. The possible states are:
* - IDLE
* - CREATING
* - UPDATING
* - DELETING
* - DELETED
* - REPAIRING
*
*/
public String stateName() {
return this.stateName;
}
/**
* @return Set that contains key-value pairs between 1 to 255 characters in length for tagging and categorizing the cluster. See below.
*
*/
public List tags() {
return this.tags;
}
/**
* @return Flag that indicates whether termination protection is enabled on the cluster. If set to true, MongoDB Cloud won't delete the cluster. If set to false, MongoDB Cloud will delete the cluster.
*
*/
public Boolean terminationProtectionEnabled() {
return this.terminationProtectionEnabled;
}
/**
* @return Release cadence that Atlas uses for this cluster.
*
*/
public String versionReleaseSystem() {
return this.versionReleaseSystem;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List advancedConfigurations;
private Boolean autoScalingComputeEnabled;
private Boolean autoScalingComputeScaleDownEnabled;
private Boolean autoScalingDiskGbEnabled;
private String backingProviderName;
private Boolean backupEnabled;
private List biConnectorConfigs;
private String clusterType;
private List connectionStrings;
private String containerId;
private Double diskSizeGb;
private String encryptionAtRestProvider;
private String id;
private List labels;
private String mongoDbMajorVersion;
private String mongoDbVersion;
private String mongoUri;
private String mongoUriUpdated;
private String mongoUriWithOptions;
private String name;
private Integer numShards;
private Boolean paused;
private Boolean pitEnabled;
private String projectId;
private String providerAutoScalingComputeMaxInstanceSize;
private String providerAutoScalingComputeMinInstanceSize;
private Boolean providerBackupEnabled;
private Integer providerDiskIops;
private String providerDiskTypeName;
private Boolean providerEncryptEbsVolume;
private Boolean providerEncryptEbsVolumeFlag;
private String providerInstanceSizeName;
private String providerName;
private String providerRegionName;
private String providerVolumeType;
private Integer replicationFactor;
private List replicationSpecs;
private List snapshotBackupPolicies;
private String srvAddress;
private String stateName;
private List tags;
private Boolean terminationProtectionEnabled;
private String versionReleaseSystem;
public Builder() {}
public Builder(GetClusterResult defaults) {
Objects.requireNonNull(defaults);
this.advancedConfigurations = defaults.advancedConfigurations;
this.autoScalingComputeEnabled = defaults.autoScalingComputeEnabled;
this.autoScalingComputeScaleDownEnabled = defaults.autoScalingComputeScaleDownEnabled;
this.autoScalingDiskGbEnabled = defaults.autoScalingDiskGbEnabled;
this.backingProviderName = defaults.backingProviderName;
this.backupEnabled = defaults.backupEnabled;
this.biConnectorConfigs = defaults.biConnectorConfigs;
this.clusterType = defaults.clusterType;
this.connectionStrings = defaults.connectionStrings;
this.containerId = defaults.containerId;
this.diskSizeGb = defaults.diskSizeGb;
this.encryptionAtRestProvider = defaults.encryptionAtRestProvider;
this.id = defaults.id;
this.labels = defaults.labels;
this.mongoDbMajorVersion = defaults.mongoDbMajorVersion;
this.mongoDbVersion = defaults.mongoDbVersion;
this.mongoUri = defaults.mongoUri;
this.mongoUriUpdated = defaults.mongoUriUpdated;
this.mongoUriWithOptions = defaults.mongoUriWithOptions;
this.name = defaults.name;
this.numShards = defaults.numShards;
this.paused = defaults.paused;
this.pitEnabled = defaults.pitEnabled;
this.projectId = defaults.projectId;
this.providerAutoScalingComputeMaxInstanceSize = defaults.providerAutoScalingComputeMaxInstanceSize;
this.providerAutoScalingComputeMinInstanceSize = defaults.providerAutoScalingComputeMinInstanceSize;
this.providerBackupEnabled = defaults.providerBackupEnabled;
this.providerDiskIops = defaults.providerDiskIops;
this.providerDiskTypeName = defaults.providerDiskTypeName;
this.providerEncryptEbsVolume = defaults.providerEncryptEbsVolume;
this.providerEncryptEbsVolumeFlag = defaults.providerEncryptEbsVolumeFlag;
this.providerInstanceSizeName = defaults.providerInstanceSizeName;
this.providerName = defaults.providerName;
this.providerRegionName = defaults.providerRegionName;
this.providerVolumeType = defaults.providerVolumeType;
this.replicationFactor = defaults.replicationFactor;
this.replicationSpecs = defaults.replicationSpecs;
this.snapshotBackupPolicies = defaults.snapshotBackupPolicies;
this.srvAddress = defaults.srvAddress;
this.stateName = defaults.stateName;
this.tags = defaults.tags;
this.terminationProtectionEnabled = defaults.terminationProtectionEnabled;
this.versionReleaseSystem = defaults.versionReleaseSystem;
}
@CustomType.Setter
public Builder advancedConfigurations(List advancedConfigurations) {
if (advancedConfigurations == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "advancedConfigurations");
}
this.advancedConfigurations = advancedConfigurations;
return this;
}
public Builder advancedConfigurations(GetClusterAdvancedConfiguration... advancedConfigurations) {
return advancedConfigurations(List.of(advancedConfigurations));
}
@CustomType.Setter
public Builder autoScalingComputeEnabled(Boolean autoScalingComputeEnabled) {
if (autoScalingComputeEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "autoScalingComputeEnabled");
}
this.autoScalingComputeEnabled = autoScalingComputeEnabled;
return this;
}
@CustomType.Setter
public Builder autoScalingComputeScaleDownEnabled(Boolean autoScalingComputeScaleDownEnabled) {
if (autoScalingComputeScaleDownEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "autoScalingComputeScaleDownEnabled");
}
this.autoScalingComputeScaleDownEnabled = autoScalingComputeScaleDownEnabled;
return this;
}
@CustomType.Setter
public Builder autoScalingDiskGbEnabled(Boolean autoScalingDiskGbEnabled) {
if (autoScalingDiskGbEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "autoScalingDiskGbEnabled");
}
this.autoScalingDiskGbEnabled = autoScalingDiskGbEnabled;
return this;
}
@CustomType.Setter
public Builder backingProviderName(String backingProviderName) {
if (backingProviderName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "backingProviderName");
}
this.backingProviderName = backingProviderName;
return this;
}
@CustomType.Setter
public Builder backupEnabled(Boolean backupEnabled) {
if (backupEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "backupEnabled");
}
this.backupEnabled = backupEnabled;
return this;
}
@CustomType.Setter
public Builder biConnectorConfigs(List biConnectorConfigs) {
if (biConnectorConfigs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "biConnectorConfigs");
}
this.biConnectorConfigs = biConnectorConfigs;
return this;
}
public Builder biConnectorConfigs(GetClusterBiConnectorConfig... biConnectorConfigs) {
return biConnectorConfigs(List.of(biConnectorConfigs));
}
@CustomType.Setter
public Builder clusterType(String clusterType) {
if (clusterType == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "clusterType");
}
this.clusterType = clusterType;
return this;
}
@CustomType.Setter
public Builder connectionStrings(List connectionStrings) {
if (connectionStrings == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "connectionStrings");
}
this.connectionStrings = connectionStrings;
return this;
}
public Builder connectionStrings(GetClusterConnectionString... connectionStrings) {
return connectionStrings(List.of(connectionStrings));
}
@CustomType.Setter
public Builder containerId(String containerId) {
if (containerId == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "containerId");
}
this.containerId = containerId;
return this;
}
@CustomType.Setter
public Builder diskSizeGb(Double diskSizeGb) {
if (diskSizeGb == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "diskSizeGb");
}
this.diskSizeGb = diskSizeGb;
return this;
}
@CustomType.Setter
public Builder encryptionAtRestProvider(String encryptionAtRestProvider) {
if (encryptionAtRestProvider == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "encryptionAtRestProvider");
}
this.encryptionAtRestProvider = encryptionAtRestProvider;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder labels(List labels) {
if (labels == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "labels");
}
this.labels = labels;
return this;
}
public Builder labels(GetClusterLabel... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder mongoDbMajorVersion(String mongoDbMajorVersion) {
if (mongoDbMajorVersion == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "mongoDbMajorVersion");
}
this.mongoDbMajorVersion = mongoDbMajorVersion;
return this;
}
@CustomType.Setter
public Builder mongoDbVersion(String mongoDbVersion) {
if (mongoDbVersion == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "mongoDbVersion");
}
this.mongoDbVersion = mongoDbVersion;
return this;
}
@CustomType.Setter
public Builder mongoUri(String mongoUri) {
if (mongoUri == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "mongoUri");
}
this.mongoUri = mongoUri;
return this;
}
@CustomType.Setter
public Builder mongoUriUpdated(String mongoUriUpdated) {
if (mongoUriUpdated == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "mongoUriUpdated");
}
this.mongoUriUpdated = mongoUriUpdated;
return this;
}
@CustomType.Setter
public Builder mongoUriWithOptions(String mongoUriWithOptions) {
if (mongoUriWithOptions == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "mongoUriWithOptions");
}
this.mongoUriWithOptions = mongoUriWithOptions;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder numShards(Integer numShards) {
if (numShards == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "numShards");
}
this.numShards = numShards;
return this;
}
@CustomType.Setter
public Builder paused(Boolean paused) {
if (paused == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "paused");
}
this.paused = paused;
return this;
}
@CustomType.Setter
public Builder pitEnabled(Boolean pitEnabled) {
if (pitEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "pitEnabled");
}
this.pitEnabled = pitEnabled;
return this;
}
@CustomType.Setter
public Builder projectId(String projectId) {
if (projectId == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "projectId");
}
this.projectId = projectId;
return this;
}
@CustomType.Setter
public Builder providerAutoScalingComputeMaxInstanceSize(String providerAutoScalingComputeMaxInstanceSize) {
if (providerAutoScalingComputeMaxInstanceSize == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerAutoScalingComputeMaxInstanceSize");
}
this.providerAutoScalingComputeMaxInstanceSize = providerAutoScalingComputeMaxInstanceSize;
return this;
}
@CustomType.Setter
public Builder providerAutoScalingComputeMinInstanceSize(String providerAutoScalingComputeMinInstanceSize) {
if (providerAutoScalingComputeMinInstanceSize == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerAutoScalingComputeMinInstanceSize");
}
this.providerAutoScalingComputeMinInstanceSize = providerAutoScalingComputeMinInstanceSize;
return this;
}
@CustomType.Setter
public Builder providerBackupEnabled(Boolean providerBackupEnabled) {
if (providerBackupEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerBackupEnabled");
}
this.providerBackupEnabled = providerBackupEnabled;
return this;
}
@CustomType.Setter
public Builder providerDiskIops(Integer providerDiskIops) {
if (providerDiskIops == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerDiskIops");
}
this.providerDiskIops = providerDiskIops;
return this;
}
@CustomType.Setter
public Builder providerDiskTypeName(String providerDiskTypeName) {
if (providerDiskTypeName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerDiskTypeName");
}
this.providerDiskTypeName = providerDiskTypeName;
return this;
}
@CustomType.Setter
public Builder providerEncryptEbsVolume(Boolean providerEncryptEbsVolume) {
if (providerEncryptEbsVolume == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerEncryptEbsVolume");
}
this.providerEncryptEbsVolume = providerEncryptEbsVolume;
return this;
}
@CustomType.Setter
public Builder providerEncryptEbsVolumeFlag(Boolean providerEncryptEbsVolumeFlag) {
if (providerEncryptEbsVolumeFlag == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerEncryptEbsVolumeFlag");
}
this.providerEncryptEbsVolumeFlag = providerEncryptEbsVolumeFlag;
return this;
}
@CustomType.Setter
public Builder providerInstanceSizeName(String providerInstanceSizeName) {
if (providerInstanceSizeName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerInstanceSizeName");
}
this.providerInstanceSizeName = providerInstanceSizeName;
return this;
}
@CustomType.Setter
public Builder providerName(String providerName) {
if (providerName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerName");
}
this.providerName = providerName;
return this;
}
@CustomType.Setter
public Builder providerRegionName(String providerRegionName) {
if (providerRegionName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerRegionName");
}
this.providerRegionName = providerRegionName;
return this;
}
@CustomType.Setter
public Builder providerVolumeType(String providerVolumeType) {
if (providerVolumeType == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "providerVolumeType");
}
this.providerVolumeType = providerVolumeType;
return this;
}
@CustomType.Setter
public Builder replicationFactor(Integer replicationFactor) {
if (replicationFactor == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "replicationFactor");
}
this.replicationFactor = replicationFactor;
return this;
}
@CustomType.Setter
public Builder replicationSpecs(List replicationSpecs) {
if (replicationSpecs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "replicationSpecs");
}
this.replicationSpecs = replicationSpecs;
return this;
}
public Builder replicationSpecs(GetClusterReplicationSpec... replicationSpecs) {
return replicationSpecs(List.of(replicationSpecs));
}
@CustomType.Setter
public Builder snapshotBackupPolicies(List snapshotBackupPolicies) {
if (snapshotBackupPolicies == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "snapshotBackupPolicies");
}
this.snapshotBackupPolicies = snapshotBackupPolicies;
return this;
}
public Builder snapshotBackupPolicies(GetClusterSnapshotBackupPolicy... snapshotBackupPolicies) {
return snapshotBackupPolicies(List.of(snapshotBackupPolicies));
}
@CustomType.Setter
public Builder srvAddress(String srvAddress) {
if (srvAddress == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "srvAddress");
}
this.srvAddress = srvAddress;
return this;
}
@CustomType.Setter
public Builder stateName(String stateName) {
if (stateName == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "stateName");
}
this.stateName = stateName;
return this;
}
@CustomType.Setter
public Builder tags(List tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "tags");
}
this.tags = tags;
return this;
}
public Builder tags(GetClusterTag... tags) {
return tags(List.of(tags));
}
@CustomType.Setter
public Builder terminationProtectionEnabled(Boolean terminationProtectionEnabled) {
if (terminationProtectionEnabled == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "terminationProtectionEnabled");
}
this.terminationProtectionEnabled = terminationProtectionEnabled;
return this;
}
@CustomType.Setter
public Builder versionReleaseSystem(String versionReleaseSystem) {
if (versionReleaseSystem == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "versionReleaseSystem");
}
this.versionReleaseSystem = versionReleaseSystem;
return this;
}
public GetClusterResult build() {
final var _resultValue = new GetClusterResult();
_resultValue.advancedConfigurations = advancedConfigurations;
_resultValue.autoScalingComputeEnabled = autoScalingComputeEnabled;
_resultValue.autoScalingComputeScaleDownEnabled = autoScalingComputeScaleDownEnabled;
_resultValue.autoScalingDiskGbEnabled = autoScalingDiskGbEnabled;
_resultValue.backingProviderName = backingProviderName;
_resultValue.backupEnabled = backupEnabled;
_resultValue.biConnectorConfigs = biConnectorConfigs;
_resultValue.clusterType = clusterType;
_resultValue.connectionStrings = connectionStrings;
_resultValue.containerId = containerId;
_resultValue.diskSizeGb = diskSizeGb;
_resultValue.encryptionAtRestProvider = encryptionAtRestProvider;
_resultValue.id = id;
_resultValue.labels = labels;
_resultValue.mongoDbMajorVersion = mongoDbMajorVersion;
_resultValue.mongoDbVersion = mongoDbVersion;
_resultValue.mongoUri = mongoUri;
_resultValue.mongoUriUpdated = mongoUriUpdated;
_resultValue.mongoUriWithOptions = mongoUriWithOptions;
_resultValue.name = name;
_resultValue.numShards = numShards;
_resultValue.paused = paused;
_resultValue.pitEnabled = pitEnabled;
_resultValue.projectId = projectId;
_resultValue.providerAutoScalingComputeMaxInstanceSize = providerAutoScalingComputeMaxInstanceSize;
_resultValue.providerAutoScalingComputeMinInstanceSize = providerAutoScalingComputeMinInstanceSize;
_resultValue.providerBackupEnabled = providerBackupEnabled;
_resultValue.providerDiskIops = providerDiskIops;
_resultValue.providerDiskTypeName = providerDiskTypeName;
_resultValue.providerEncryptEbsVolume = providerEncryptEbsVolume;
_resultValue.providerEncryptEbsVolumeFlag = providerEncryptEbsVolumeFlag;
_resultValue.providerInstanceSizeName = providerInstanceSizeName;
_resultValue.providerName = providerName;
_resultValue.providerRegionName = providerRegionName;
_resultValue.providerVolumeType = providerVolumeType;
_resultValue.replicationFactor = replicationFactor;
_resultValue.replicationSpecs = replicationSpecs;
_resultValue.snapshotBackupPolicies = snapshotBackupPolicies;
_resultValue.srvAddress = srvAddress;
_resultValue.stateName = stateName;
_resultValue.tags = tags;
_resultValue.terminationProtectionEnabled = terminationProtectionEnabled;
_resultValue.versionReleaseSystem = versionReleaseSystem;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy