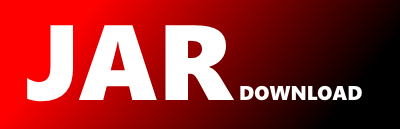
com.pulumi.mongodbatlas.outputs.GetDataLakePipelineRunsResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.mongodbatlas.outputs.GetDataLakePipelineRunsResultStat;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetDataLakePipelineRunsResult {
/**
* @return Backup schedule interval of the Data Lake Pipeline.
*
*/
private String backupFrequencyType;
/**
* @return Timestamp that indicates when the pipeline run was created.
*
*/
private String createdDate;
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
private String id;
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
private String lastUpdatedDate;
/**
* @return Processing phase of the Data Lake Pipeline.
*
*/
private String phase;
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline.
*
*/
private String pipelineId;
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
private String pipelineRunId;
/**
* @return Unique 24-hexadecimal character string that identifies the snapshot of a cluster.
*
*/
private String snapshotId;
/**
* @return State of the pipeline run.
*
*/
private String state;
/**
* @return Runtime statistics for this Data Lake Pipeline run.
*
*/
private List stats;
private GetDataLakePipelineRunsResult() {}
/**
* @return Backup schedule interval of the Data Lake Pipeline.
*
*/
public String backupFrequencyType() {
return this.backupFrequencyType;
}
/**
* @return Timestamp that indicates when the pipeline run was created.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
public String id() {
return this.id;
}
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
public String lastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
* @return Processing phase of the Data Lake Pipeline.
*
*/
public String phase() {
return this.phase;
}
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline.
*
*/
public String pipelineId() {
return this.pipelineId;
}
/**
* @return Unique 24-hexadecimal character string that identifies a Data Lake Pipeline run.
*
*/
public String pipelineRunId() {
return this.pipelineRunId;
}
/**
* @return Unique 24-hexadecimal character string that identifies the snapshot of a cluster.
*
*/
public String snapshotId() {
return this.snapshotId;
}
/**
* @return State of the pipeline run.
*
*/
public String state() {
return this.state;
}
/**
* @return Runtime statistics for this Data Lake Pipeline run.
*
*/
public List stats() {
return this.stats;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDataLakePipelineRunsResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String backupFrequencyType;
private String createdDate;
private String id;
private String lastUpdatedDate;
private String phase;
private String pipelineId;
private String pipelineRunId;
private String snapshotId;
private String state;
private List stats;
public Builder() {}
public Builder(GetDataLakePipelineRunsResult defaults) {
Objects.requireNonNull(defaults);
this.backupFrequencyType = defaults.backupFrequencyType;
this.createdDate = defaults.createdDate;
this.id = defaults.id;
this.lastUpdatedDate = defaults.lastUpdatedDate;
this.phase = defaults.phase;
this.pipelineId = defaults.pipelineId;
this.pipelineRunId = defaults.pipelineRunId;
this.snapshotId = defaults.snapshotId;
this.state = defaults.state;
this.stats = defaults.stats;
}
@CustomType.Setter
public Builder backupFrequencyType(String backupFrequencyType) {
if (backupFrequencyType == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "backupFrequencyType");
}
this.backupFrequencyType = backupFrequencyType;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastUpdatedDate(String lastUpdatedDate) {
if (lastUpdatedDate == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "lastUpdatedDate");
}
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
@CustomType.Setter
public Builder phase(String phase) {
if (phase == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "phase");
}
this.phase = phase;
return this;
}
@CustomType.Setter
public Builder pipelineId(String pipelineId) {
if (pipelineId == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "pipelineId");
}
this.pipelineId = pipelineId;
return this;
}
@CustomType.Setter
public Builder pipelineRunId(String pipelineRunId) {
if (pipelineRunId == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "pipelineRunId");
}
this.pipelineRunId = pipelineRunId;
return this;
}
@CustomType.Setter
public Builder snapshotId(String snapshotId) {
if (snapshotId == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "snapshotId");
}
this.snapshotId = snapshotId;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder stats(List stats) {
if (stats == null) {
throw new MissingRequiredPropertyException("GetDataLakePipelineRunsResult", "stats");
}
this.stats = stats;
return this;
}
public Builder stats(GetDataLakePipelineRunsResultStat... stats) {
return stats(List.of(stats));
}
public GetDataLakePipelineRunsResult build() {
final var _resultValue = new GetDataLakePipelineRunsResult();
_resultValue.backupFrequencyType = backupFrequencyType;
_resultValue.createdDate = createdDate;
_resultValue.id = id;
_resultValue.lastUpdatedDate = lastUpdatedDate;
_resultValue.phase = phase;
_resultValue.pipelineId = pipelineId;
_resultValue.pipelineRunId = pipelineRunId;
_resultValue.snapshotId = snapshotId;
_resultValue.state = state;
_resultValue.stats = stats;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy