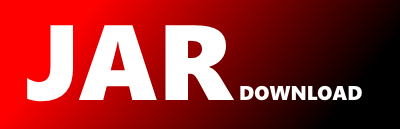
com.pulumi.mongodbatlas.outputs.GetEventTriggersResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.mongodbatlas.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.mongodbatlas.outputs.GetEventTriggersResultEventProcessor;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetEventTriggersResult {
/**
* @return The name of the MongoDB collection that the trigger watches for change events.
*
*/
private String configCollection;
/**
* @return The name of the MongoDB database to watch.
*
*/
private String configDatabase;
/**
* @return If true, indicates that `UPDATE` change events should include the most current [majority-committed](https://docs.mongodb.com/manual/reference/read-concern-majority/) version of the modified document in the fullDocument field.
*
*/
private Boolean configFullDocument;
private Boolean configFullDocumentBefore;
/**
* @return A [$match](https://docs.mongodb.com/manual/reference/operator/aggregation/match/) expression document that MongoDB Realm includes in the underlying change stream pipeline for the trigger.
*
*/
private String configMatch;
/**
* @return The [authentication operation type](https://docs.mongodb.com/realm/triggers/authentication-triggers/#std-label-authentication-event-operation-types) to listen for.
*
*/
private String configOperationType;
/**
* @return The [database event operation types](https://docs.mongodb.com/realm/triggers/database-triggers/#std-label-database-events) to listen for.
*
*/
private List configOperationTypes;
/**
* @return A [$project](https://docs.mongodb.com/manual/reference/operator/aggregation/project/) expression document that Realm uses to filter the fields that appear in change event objects.
*
*/
private String configProject;
/**
* @return A list of one or more [authentication provider](https://docs.mongodb.com/realm/authentication/providers/) id values. The trigger will only listen for authentication events produced by these providers.
*
*/
private List configProviders;
/**
* @return A [cron expression](https://docs.mongodb.com/realm/triggers/cron-expressions/) that defines the trigger schedule.
*
*/
private String configSchedule;
private String configScheduleType;
/**
* @return The ID of the MongoDB Service associated with the trigger.
*
*/
private String configServiceId;
/**
* @return Status of a trigger.
*
*/
private Boolean disabled;
/**
* @return An object where each field name is an event processor ID and each value is an object that configures its corresponding event processor.
*
*/
private List eventProcessors;
/**
* @return The ID of the function associated with the trigger.
*
*/
private String functionId;
/**
* @return The name of the function associated with the trigger.
*
*/
private String functionName;
/**
* @return The name of the trigger.
*
*/
private String name;
private String triggerId;
/**
* @return The type of the trigger. Possible Values: `DATABASE`, `AUTHENTICATION`
*
*/
private String type;
/**
* @return Sort order for `DATABASE` type.
*
*/
private Boolean unordered;
private GetEventTriggersResult() {}
/**
* @return The name of the MongoDB collection that the trigger watches for change events.
*
*/
public String configCollection() {
return this.configCollection;
}
/**
* @return The name of the MongoDB database to watch.
*
*/
public String configDatabase() {
return this.configDatabase;
}
/**
* @return If true, indicates that `UPDATE` change events should include the most current [majority-committed](https://docs.mongodb.com/manual/reference/read-concern-majority/) version of the modified document in the fullDocument field.
*
*/
public Boolean configFullDocument() {
return this.configFullDocument;
}
public Boolean configFullDocumentBefore() {
return this.configFullDocumentBefore;
}
/**
* @return A [$match](https://docs.mongodb.com/manual/reference/operator/aggregation/match/) expression document that MongoDB Realm includes in the underlying change stream pipeline for the trigger.
*
*/
public String configMatch() {
return this.configMatch;
}
/**
* @return The [authentication operation type](https://docs.mongodb.com/realm/triggers/authentication-triggers/#std-label-authentication-event-operation-types) to listen for.
*
*/
public String configOperationType() {
return this.configOperationType;
}
/**
* @return The [database event operation types](https://docs.mongodb.com/realm/triggers/database-triggers/#std-label-database-events) to listen for.
*
*/
public List configOperationTypes() {
return this.configOperationTypes;
}
/**
* @return A [$project](https://docs.mongodb.com/manual/reference/operator/aggregation/project/) expression document that Realm uses to filter the fields that appear in change event objects.
*
*/
public String configProject() {
return this.configProject;
}
/**
* @return A list of one or more [authentication provider](https://docs.mongodb.com/realm/authentication/providers/) id values. The trigger will only listen for authentication events produced by these providers.
*
*/
public List configProviders() {
return this.configProviders;
}
/**
* @return A [cron expression](https://docs.mongodb.com/realm/triggers/cron-expressions/) that defines the trigger schedule.
*
*/
public String configSchedule() {
return this.configSchedule;
}
public String configScheduleType() {
return this.configScheduleType;
}
/**
* @return The ID of the MongoDB Service associated with the trigger.
*
*/
public String configServiceId() {
return this.configServiceId;
}
/**
* @return Status of a trigger.
*
*/
public Boolean disabled() {
return this.disabled;
}
/**
* @return An object where each field name is an event processor ID and each value is an object that configures its corresponding event processor.
*
*/
public List eventProcessors() {
return this.eventProcessors;
}
/**
* @return The ID of the function associated with the trigger.
*
*/
public String functionId() {
return this.functionId;
}
/**
* @return The name of the function associated with the trigger.
*
*/
public String functionName() {
return this.functionName;
}
/**
* @return The name of the trigger.
*
*/
public String name() {
return this.name;
}
public String triggerId() {
return this.triggerId;
}
/**
* @return The type of the trigger. Possible Values: `DATABASE`, `AUTHENTICATION`
*
*/
public String type() {
return this.type;
}
/**
* @return Sort order for `DATABASE` type.
*
*/
public Boolean unordered() {
return this.unordered;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEventTriggersResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String configCollection;
private String configDatabase;
private Boolean configFullDocument;
private Boolean configFullDocumentBefore;
private String configMatch;
private String configOperationType;
private List configOperationTypes;
private String configProject;
private List configProviders;
private String configSchedule;
private String configScheduleType;
private String configServiceId;
private Boolean disabled;
private List eventProcessors;
private String functionId;
private String functionName;
private String name;
private String triggerId;
private String type;
private Boolean unordered;
public Builder() {}
public Builder(GetEventTriggersResult defaults) {
Objects.requireNonNull(defaults);
this.configCollection = defaults.configCollection;
this.configDatabase = defaults.configDatabase;
this.configFullDocument = defaults.configFullDocument;
this.configFullDocumentBefore = defaults.configFullDocumentBefore;
this.configMatch = defaults.configMatch;
this.configOperationType = defaults.configOperationType;
this.configOperationTypes = defaults.configOperationTypes;
this.configProject = defaults.configProject;
this.configProviders = defaults.configProviders;
this.configSchedule = defaults.configSchedule;
this.configScheduleType = defaults.configScheduleType;
this.configServiceId = defaults.configServiceId;
this.disabled = defaults.disabled;
this.eventProcessors = defaults.eventProcessors;
this.functionId = defaults.functionId;
this.functionName = defaults.functionName;
this.name = defaults.name;
this.triggerId = defaults.triggerId;
this.type = defaults.type;
this.unordered = defaults.unordered;
}
@CustomType.Setter
public Builder configCollection(String configCollection) {
if (configCollection == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configCollection");
}
this.configCollection = configCollection;
return this;
}
@CustomType.Setter
public Builder configDatabase(String configDatabase) {
if (configDatabase == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configDatabase");
}
this.configDatabase = configDatabase;
return this;
}
@CustomType.Setter
public Builder configFullDocument(Boolean configFullDocument) {
if (configFullDocument == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configFullDocument");
}
this.configFullDocument = configFullDocument;
return this;
}
@CustomType.Setter
public Builder configFullDocumentBefore(Boolean configFullDocumentBefore) {
if (configFullDocumentBefore == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configFullDocumentBefore");
}
this.configFullDocumentBefore = configFullDocumentBefore;
return this;
}
@CustomType.Setter
public Builder configMatch(String configMatch) {
if (configMatch == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configMatch");
}
this.configMatch = configMatch;
return this;
}
@CustomType.Setter
public Builder configOperationType(String configOperationType) {
if (configOperationType == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configOperationType");
}
this.configOperationType = configOperationType;
return this;
}
@CustomType.Setter
public Builder configOperationTypes(List configOperationTypes) {
if (configOperationTypes == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configOperationTypes");
}
this.configOperationTypes = configOperationTypes;
return this;
}
public Builder configOperationTypes(String... configOperationTypes) {
return configOperationTypes(List.of(configOperationTypes));
}
@CustomType.Setter
public Builder configProject(String configProject) {
if (configProject == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configProject");
}
this.configProject = configProject;
return this;
}
@CustomType.Setter
public Builder configProviders(List configProviders) {
if (configProviders == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configProviders");
}
this.configProviders = configProviders;
return this;
}
public Builder configProviders(String... configProviders) {
return configProviders(List.of(configProviders));
}
@CustomType.Setter
public Builder configSchedule(String configSchedule) {
if (configSchedule == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configSchedule");
}
this.configSchedule = configSchedule;
return this;
}
@CustomType.Setter
public Builder configScheduleType(String configScheduleType) {
if (configScheduleType == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configScheduleType");
}
this.configScheduleType = configScheduleType;
return this;
}
@CustomType.Setter
public Builder configServiceId(String configServiceId) {
if (configServiceId == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "configServiceId");
}
this.configServiceId = configServiceId;
return this;
}
@CustomType.Setter
public Builder disabled(Boolean disabled) {
if (disabled == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "disabled");
}
this.disabled = disabled;
return this;
}
@CustomType.Setter
public Builder eventProcessors(List eventProcessors) {
if (eventProcessors == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "eventProcessors");
}
this.eventProcessors = eventProcessors;
return this;
}
public Builder eventProcessors(GetEventTriggersResultEventProcessor... eventProcessors) {
return eventProcessors(List.of(eventProcessors));
}
@CustomType.Setter
public Builder functionId(String functionId) {
if (functionId == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "functionId");
}
this.functionId = functionId;
return this;
}
@CustomType.Setter
public Builder functionName(String functionName) {
if (functionName == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "functionName");
}
this.functionName = functionName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder triggerId(String triggerId) {
if (triggerId == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "triggerId");
}
this.triggerId = triggerId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder unordered(Boolean unordered) {
if (unordered == null) {
throw new MissingRequiredPropertyException("GetEventTriggersResult", "unordered");
}
this.unordered = unordered;
return this;
}
public GetEventTriggersResult build() {
final var _resultValue = new GetEventTriggersResult();
_resultValue.configCollection = configCollection;
_resultValue.configDatabase = configDatabase;
_resultValue.configFullDocument = configFullDocument;
_resultValue.configFullDocumentBefore = configFullDocumentBefore;
_resultValue.configMatch = configMatch;
_resultValue.configOperationType = configOperationType;
_resultValue.configOperationTypes = configOperationTypes;
_resultValue.configProject = configProject;
_resultValue.configProviders = configProviders;
_resultValue.configSchedule = configSchedule;
_resultValue.configScheduleType = configScheduleType;
_resultValue.configServiceId = configServiceId;
_resultValue.disabled = disabled;
_resultValue.eventProcessors = eventProcessors;
_resultValue.functionId = functionId;
_resultValue.functionName = functionName;
_resultValue.name = name;
_resultValue.triggerId = triggerId;
_resultValue.type = type;
_resultValue.unordered = unordered;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy