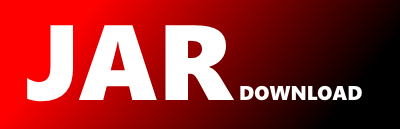
com.pulumi.ns1.inputs.UserState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ns1 Show documentation
Show all versions of ns1 Show documentation
A Pulumi package for creating and managing ns1 cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.ns1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.ns1.inputs.UserDnsRecordsAllowArgs;
import com.pulumi.ns1.inputs.UserDnsRecordsDenyArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserState extends com.pulumi.resources.ResourceArgs {
public static final UserState Empty = new UserState();
/**
* Whether the user can modify account settings.
*
*/
@Import(name="accountManageAccountSettings")
private @Nullable Output accountManageAccountSettings;
/**
* @return Whether the user can modify account settings.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy