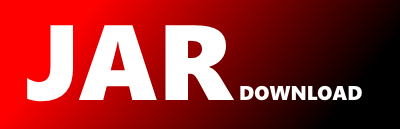
com.pulumi.ns1.Redirect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ns1 Show documentation
Show all versions of ns1 Show documentation
A Pulumi package for creating and managing ns1 cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.ns1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.ns1.RedirectArgs;
import com.pulumi.ns1.Utilities;
import com.pulumi.ns1.inputs.RedirectState;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a NS1 Redirect resource. This can be used to create, modify, and delete redirects.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ns1.Redirect;
* import com.pulumi.ns1.RedirectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Redirect("example", RedirectArgs.builder()
* .domain("www.example.com")
* .path("/from/path")
* .target("https://url.com/target/path")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Additional Examples
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ns1.RedirectCertificate;
* import com.pulumi.ns1.RedirectCertificateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new RedirectCertificate("example", RedirectCertificateArgs.builder()
* .domain("www.example.com")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## NS1 Documentation
*
* [Redirect Api Doc](https://ns1.com/api#redirect)
*
* # ns1\_redirect\_certificate
*
* Provides a NS1 Redirect Certificate resource. This can be used to create, modify, and delete redirect certificates.
*
* ## NS1 Documentation
*
* [Redirect Api Doc](https://ns1.com/api#redirect)
*
*/
@ResourceType(type="ns1:index/redirect:Redirect")
public class Redirect extends com.pulumi.resources.CustomResource {
@Export(name="certificateId", refs={String.class}, tree="[0]")
private Output certificateId;
public Output certificateId() {
return this.certificateId;
}
/**
* The domain the redirect refers to.
*
*/
@Export(name="domain", refs={String.class}, tree="[0]")
private Output domain;
/**
* @return The domain the redirect refers to.
*
*/
public Output domain() {
return this.domain;
}
/**
* How the target is interpreted:
* * __all__ appends the entire incoming path to the target destination;
* * __capture__ appends only the part of the incoming path corresponding to the wildcard (*);
* * __none__ does not append any part of the incoming path.
*
*/
@Export(name="forwardingMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forwardingMode;
/**
* @return How the target is interpreted:
* * __all__ appends the entire incoming path to the target destination;
* * __capture__ appends only the part of the incoming path corresponding to the wildcard (*);
* * __none__ does not append any part of the incoming path.
*
*/
public Output> forwardingMode() {
return Codegen.optional(this.forwardingMode);
}
/**
* How the redirect is executed:
* * __permanent__ (HTTP 301) indicates to search engines that they should remove the old page from
* their database and replace it with the new target page (this is recommended for SEO);
* * __temporary__ (HTTP 302) less common, indicates that search engines should keep the old domain or
* page indexed as the redirect is only temporary (while both pages might appear in the
* search results, a temporary redirect suggests to the search engine that it should
* prefer the new target page);
* * __masking__ preserves the redirected domain in the browser's address bar (this lets users see the
* address they entered, even though the displayed content comes from a different web page).
*
*/
@Export(name="forwardingType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forwardingType;
/**
* @return How the redirect is executed:
* * __permanent__ (HTTP 301) indicates to search engines that they should remove the old page from
* their database and replace it with the new target page (this is recommended for SEO);
* * __temporary__ (HTTP 302) less common, indicates that search engines should keep the old domain or
* page indexed as the redirect is only temporary (while both pages might appear in the
* search results, a temporary redirect suggests to the search engine that it should
* prefer the new target page);
* * __masking__ preserves the redirected domain in the browser's address bar (this lets users see the
* address they entered, even though the displayed content comes from a different web page).
*
*/
public Output> forwardingType() {
return Codegen.optional(this.forwardingType);
}
/**
* True if HTTPS is supported on the source domain by using Let's Encrypt certificates.
*
*/
@Export(name="httpsEnabled", refs={Boolean.class}, tree="[0]")
private Output httpsEnabled;
/**
* @return True if HTTPS is supported on the source domain by using Let's Encrypt certificates.
*
*/
public Output httpsEnabled() {
return this.httpsEnabled;
}
/**
* Forces redirect for users that try to visit HTTP domain to HTTPS instead.
*
*/
@Export(name="httpsForced", refs={Boolean.class}, tree="[0]")
private Output httpsForced;
/**
* @return Forces redirect for users that try to visit HTTP domain to HTTPS instead.
*
*/
public Output httpsForced() {
return this.httpsForced;
}
/**
* The Unix timestamp representing when the certificate was last signed.
*
*/
@Export(name="lastUpdated", refs={Integer.class}, tree="[0]")
private Output lastUpdated;
/**
* @return The Unix timestamp representing when the certificate was last signed.
*
*/
public Output lastUpdated() {
return this.lastUpdated;
}
/**
* The path on the domain to redirect from.
*
*/
@Export(name="path", refs={String.class}, tree="[0]")
private Output path;
/**
* @return The path on the domain to redirect from.
*
*/
public Output path() {
return this.path;
}
/**
* Enables the query string of a URL to be applied directly to the new target URL.
*
*/
@Export(name="queryForwarding", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> queryForwarding;
/**
* @return Enables the query string of a URL to be applied directly to the new target URL.
*
*/
public Output> queryForwarding() {
return Codegen.optional(this.queryForwarding);
}
/**
* Tags associated with the configuration.
*
*/
@Export(name="tags", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> tags;
/**
* @return Tags associated with the configuration.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The URL to redirect to.
*
*/
@Export(name="target", refs={String.class}, tree="[0]")
private Output target;
/**
* @return The URL to redirect to.
*
*/
public Output target() {
return this.target;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Redirect(java.lang.String name) {
this(name, RedirectArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Redirect(java.lang.String name, RedirectArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Redirect(java.lang.String name, RedirectArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ns1:index/redirect:Redirect", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Redirect(java.lang.String name, Output id, @Nullable RedirectState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ns1:index/redirect:Redirect", name, state, makeResourceOptions(options, id), false);
}
private static RedirectArgs makeArgs(RedirectArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? RedirectArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Redirect get(java.lang.String name, Output id, @Nullable RedirectState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Redirect(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy