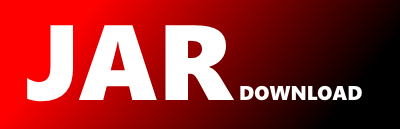
com.pulumi.okta.app.inputs.GetOauthPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.app.inputs;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetOauthPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetOauthPlainArgs Empty = new GetOauthPlainArgs();
/**
* Search only ACTIVE applications.
*
*/
@Import(name="activeOnly")
private @Nullable Boolean activeOnly;
/**
* @return Search only ACTIVE applications.
*
*/
public Optional activeOnly() {
return Optional.ofNullable(this.activeOnly);
}
/**
* Id of application to retrieve, conflicts with label and label_prefix.
*
*/
@Import(name="id")
private @Nullable String id;
/**
* @return Id of application to retrieve, conflicts with label and label_prefix.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* The label of the app to retrieve, conflicts with
* label_prefix and id. Label uses the ?q=\n\n query parameter exposed by
* Okta's List Apps API. The API will search both name and label using that
* query. Therefore similarily named and labeled apps may be returned in the query
* and have the unitended result of associating the wrong app with this data
* source. See:
* https://developer.okta.com/docs/reference/api/apps/#list-applications
*
*/
@Import(name="label")
private @Nullable String label;
/**
* @return The label of the app to retrieve, conflicts with
* label_prefix and id. Label uses the ?q=\n\n query parameter exposed by
* Okta's List Apps API. The API will search both name and label using that
* query. Therefore similarily named and labeled apps may be returned in the query
* and have the unitended result of associating the wrong app with this data
* source. See:
* https://developer.okta.com/docs/reference/api/apps/#list-applications
*
*/
public Optional label() {
return Optional.ofNullable(this.label);
}
/**
* Label prefix of the app to retrieve, conflicts with label and id. This will tell the
* provider to do a starts with query as opposed to an equals query.
*
*/
@Import(name="labelPrefix")
private @Nullable String labelPrefix;
/**
* @return Label prefix of the app to retrieve, conflicts with label and id. This will tell the
* provider to do a starts with query as opposed to an equals query.
*
*/
public Optional labelPrefix() {
return Optional.ofNullable(this.labelPrefix);
}
/**
* Ignore groups sync. This is a temporary solution until 'groups' field is supported in all the app-like resources
*
* @deprecated
* Because groups has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because groups has been removed, this attribute is a no op and will be removed */
@Import(name="skipGroups")
private @Nullable Boolean skipGroups;
/**
* @return Ignore groups sync. This is a temporary solution until 'groups' field is supported in all the app-like resources
*
* @deprecated
* Because groups has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because groups has been removed, this attribute is a no op and will be removed */
public Optional skipGroups() {
return Optional.ofNullable(this.skipGroups);
}
/**
* Ignore users sync. This is a temporary solution until 'users' field is supported in all the app-like resources
*
* @deprecated
* Because users has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because users has been removed, this attribute is a no op and will be removed */
@Import(name="skipUsers")
private @Nullable Boolean skipUsers;
/**
* @return Ignore users sync. This is a temporary solution until 'users' field is supported in all the app-like resources
*
* @deprecated
* Because users has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because users has been removed, this attribute is a no op and will be removed */
public Optional skipUsers() {
return Optional.ofNullable(this.skipUsers);
}
private GetOauthPlainArgs() {}
private GetOauthPlainArgs(GetOauthPlainArgs $) {
this.activeOnly = $.activeOnly;
this.id = $.id;
this.label = $.label;
this.labelPrefix = $.labelPrefix;
this.skipGroups = $.skipGroups;
this.skipUsers = $.skipUsers;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetOauthPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetOauthPlainArgs $;
public Builder() {
$ = new GetOauthPlainArgs();
}
public Builder(GetOauthPlainArgs defaults) {
$ = new GetOauthPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param activeOnly Search only ACTIVE applications.
*
* @return builder
*
*/
public Builder activeOnly(@Nullable Boolean activeOnly) {
$.activeOnly = activeOnly;
return this;
}
/**
* @param id Id of application to retrieve, conflicts with label and label_prefix.
*
* @return builder
*
*/
public Builder id(@Nullable String id) {
$.id = id;
return this;
}
/**
* @param label The label of the app to retrieve, conflicts with
* label_prefix and id. Label uses the ?q=\n\n query parameter exposed by
* Okta's List Apps API. The API will search both name and label using that
* query. Therefore similarily named and labeled apps may be returned in the query
* and have the unitended result of associating the wrong app with this data
* source. See:
* https://developer.okta.com/docs/reference/api/apps/#list-applications
*
* @return builder
*
*/
public Builder label(@Nullable String label) {
$.label = label;
return this;
}
/**
* @param labelPrefix Label prefix of the app to retrieve, conflicts with label and id. This will tell the
* provider to do a starts with query as opposed to an equals query.
*
* @return builder
*
*/
public Builder labelPrefix(@Nullable String labelPrefix) {
$.labelPrefix = labelPrefix;
return this;
}
/**
* @param skipGroups Ignore groups sync. This is a temporary solution until 'groups' field is supported in all the app-like resources
*
* @return builder
*
* @deprecated
* Because groups has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because groups has been removed, this attribute is a no op and will be removed */
public Builder skipGroups(@Nullable Boolean skipGroups) {
$.skipGroups = skipGroups;
return this;
}
/**
* @param skipUsers Ignore users sync. This is a temporary solution until 'users' field is supported in all the app-like resources
*
* @return builder
*
* @deprecated
* Because users has been removed, this attribute is a no op and will be removed
*
*/
@Deprecated /* Because users has been removed, this attribute is a no op and will be removed */
public Builder skipUsers(@Nullable Boolean skipUsers) {
$.skipUsers = skipUsers;
return this;
}
public GetOauthPlainArgs build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy