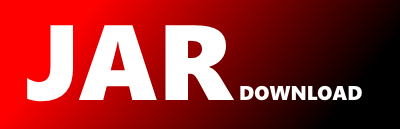
com.pulumi.okta.auth.ServerPolicyClaim Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.auth;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.okta.Utilities;
import com.pulumi.okta.auth.ServerPolicyClaimArgs;
import com.pulumi.okta.auth.inputs.ServerPolicyClaimState;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.okta.auth.ServerPolicyRule;
* import com.pulumi.okta.auth.ServerPolicyRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ServerPolicyRule("example", ServerPolicyRuleArgs.builder()
* .authServerId("")
* .policyId("")
* .status("ACTIVE")
* .name("example")
* .priority(1)
* .groupWhitelists("")
* .grantTypeWhitelists("implicit")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* ```sh
* $ pulumi import okta:auth/serverPolicyClaim:ServerPolicyClaim example <auth_server_id>/<policy_id>/<policy_rule_id>
* ```
*
* @deprecated
* okta.auth/serverpolicyclaim.ServerPolicyClaim has been deprecated in favor of okta.auth/serverpolicyrule.ServerPolicyRule
*
*/
@Deprecated /* okta.auth/serverpolicyclaim.ServerPolicyClaim has been deprecated in favor of okta.auth/serverpolicyrule.ServerPolicyRule */
@ResourceType(type="okta:auth/serverPolicyClaim:ServerPolicyClaim")
public class ServerPolicyClaim extends com.pulumi.resources.CustomResource {
/**
* Lifetime of access token. Can be set to a value between 5 and 1440 minutes. Default is `60`.
*
*/
@Export(name="accessTokenLifetimeMinutes", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> accessTokenLifetimeMinutes;
/**
* @return Lifetime of access token. Can be set to a value between 5 and 1440 minutes. Default is `60`.
*
*/
public Output> accessTokenLifetimeMinutes() {
return Codegen.optional(this.accessTokenLifetimeMinutes);
}
/**
* Auth server ID
*
*/
@Export(name="authServerId", refs={String.class}, tree="[0]")
private Output authServerId;
/**
* @return Auth server ID
*
*/
public Output authServerId() {
return this.authServerId;
}
/**
* Accepted grant type values, `authorization_code`, `implicit`, `password`, `client_credentials`, `urn:ietf:params:oauth:grant-type:saml2-bearer` (*Early Access Property*), `urn:ietf:params:oauth:grant-type:token-exchange` (*Early Access Property*),`urn:ietf:params:oauth:grant-type:device_code` (*Early Access Property*), `interaction_code` (*OIE only*). For `implicit` value either `user_whitelist` or `group_whitelist` should be set.
*
*/
@Export(name="grantTypeWhitelists", refs={List.class,String.class}, tree="[0,1]")
private Output> grantTypeWhitelists;
/**
* @return Accepted grant type values, `authorization_code`, `implicit`, `password`, `client_credentials`, `urn:ietf:params:oauth:grant-type:saml2-bearer` (*Early Access Property*), `urn:ietf:params:oauth:grant-type:token-exchange` (*Early Access Property*),`urn:ietf:params:oauth:grant-type:device_code` (*Early Access Property*), `interaction_code` (*OIE only*). For `implicit` value either `user_whitelist` or `group_whitelist` should be set.
*
*/
public Output> grantTypeWhitelists() {
return this.grantTypeWhitelists;
}
/**
* Specifies a set of Groups whose Users are to be excluded.
*
*/
@Export(name="groupBlacklists", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> groupBlacklists;
/**
* @return Specifies a set of Groups whose Users are to be excluded.
*
*/
public Output>> groupBlacklists() {
return Codegen.optional(this.groupBlacklists);
}
/**
* Specifies a set of Groups whose Users are to be included. Can be set to Group ID or to the following: `EVERYONE`.
*
*/
@Export(name="groupWhitelists", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> groupWhitelists;
/**
* @return Specifies a set of Groups whose Users are to be included. Can be set to Group ID or to the following: `EVERYONE`.
*
*/
public Output>> groupWhitelists() {
return Codegen.optional(this.groupWhitelists);
}
/**
* The ID of the inline token to trigger.
*
*/
@Export(name="inlineHookId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> inlineHookId;
/**
* @return The ID of the inline token to trigger.
*
*/
public Output> inlineHookId() {
return Codegen.optional(this.inlineHookId);
}
/**
* Auth server policy rule name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Auth server policy rule name
*
*/
public Output name() {
return this.name;
}
/**
* Auth server policy ID
*
*/
@Export(name="policyId", refs={String.class}, tree="[0]")
private Output policyId;
/**
* @return Auth server policy ID
*
*/
public Output policyId() {
return this.policyId;
}
/**
* Priority of the auth server policy rule
*
*/
@Export(name="priority", refs={Integer.class}, tree="[0]")
private Output priority;
/**
* @return Priority of the auth server policy rule
*
*/
public Output priority() {
return this.priority;
}
/**
* Lifetime of refresh token.
*
*/
@Export(name="refreshTokenLifetimeMinutes", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> refreshTokenLifetimeMinutes;
/**
* @return Lifetime of refresh token.
*
*/
public Output> refreshTokenLifetimeMinutes() {
return Codegen.optional(this.refreshTokenLifetimeMinutes);
}
/**
* Window in which a refresh token can be used. It can be a value between 5 and 2628000 (5 years) minutes. Default is `10080` (7 days).`refresh_token_window_minutes` must be between `access_token_lifetime_minutes` and `refresh_token_lifetime_minutes`.
*
*/
@Export(name="refreshTokenWindowMinutes", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> refreshTokenWindowMinutes;
/**
* @return Window in which a refresh token can be used. It can be a value between 5 and 2628000 (5 years) minutes. Default is `10080` (7 days).`refresh_token_window_minutes` must be between `access_token_lifetime_minutes` and `refresh_token_lifetime_minutes`.
*
*/
public Output> refreshTokenWindowMinutes() {
return Codegen.optional(this.refreshTokenWindowMinutes);
}
/**
* Scopes allowed for this policy rule. They can be whitelisted by name or all can be whitelisted with `*`
*
*/
@Export(name="scopeWhitelists", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> scopeWhitelists;
/**
* @return Scopes allowed for this policy rule. They can be whitelisted by name or all can be whitelisted with `*`
*
*/
public Output>> scopeWhitelists() {
return Codegen.optional(this.scopeWhitelists);
}
/**
* Default to `ACTIVE`
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> status;
/**
* @return Default to `ACTIVE`
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
* The rule is the system (default) rule for its associated policy
*
*/
@Export(name="system", refs={Boolean.class}, tree="[0]")
private Output system;
/**
* @return The rule is the system (default) rule for its associated policy
*
*/
public Output system() {
return this.system;
}
/**
* Auth server policy rule type, unlikely this will be anything other then the default
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> type;
/**
* @return Auth server policy rule type, unlikely this will be anything other then the default
*
*/
public Output> type() {
return Codegen.optional(this.type);
}
/**
* Specifies a set of Users to be excluded.
*
*/
@Export(name="userBlacklists", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> userBlacklists;
/**
* @return Specifies a set of Users to be excluded.
*
*/
public Output>> userBlacklists() {
return Codegen.optional(this.userBlacklists);
}
/**
* Specifies a set of Users to be included.
*
*/
@Export(name="userWhitelists", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> userWhitelists;
/**
* @return Specifies a set of Users to be included.
*
*/
public Output>> userWhitelists() {
return Codegen.optional(this.userWhitelists);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ServerPolicyClaim(java.lang.String name) {
this(name, ServerPolicyClaimArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ServerPolicyClaim(java.lang.String name, ServerPolicyClaimArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ServerPolicyClaim(java.lang.String name, ServerPolicyClaimArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("okta:auth/serverPolicyClaim:ServerPolicyClaim", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ServerPolicyClaim(java.lang.String name, Output id, @Nullable ServerPolicyClaimState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("okta:auth/serverPolicyClaim:ServerPolicyClaim", name, state, makeResourceOptions(options, id), false);
}
private static ServerPolicyClaimArgs makeArgs(ServerPolicyClaimArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ServerPolicyClaimArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ServerPolicyClaim get(java.lang.String name, Output id, @Nullable ServerPolicyClaimState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ServerPolicyClaim(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy