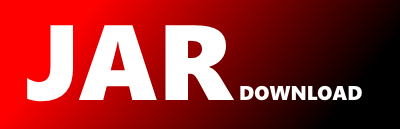
com.pulumi.okta.auth.inputs.ServerScopeState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.auth.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServerScopeState extends com.pulumi.resources.ResourceArgs {
public static final ServerScopeState Empty = new ServerScopeState();
/**
* Auth server ID
*
*/
@Import(name="authServerId")
private @Nullable Output authServerId;
/**
* @return Auth server ID
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy