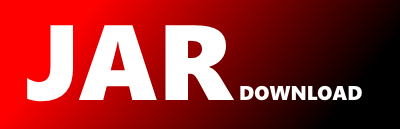
com.pulumi.okta.inputs.BrandState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BrandState extends com.pulumi.resources.ResourceArgs {
public static final BrandState Empty = new BrandState();
/**
* Is a required input flag with when changing custom*privacy*url, shouldn't be considered as a readable property
*
*/
@Import(name="agreeToCustomPrivacyPolicy")
private @Nullable Output agreeToCustomPrivacyPolicy;
/**
* @return Is a required input flag with when changing custom*privacy*url, shouldn't be considered as a readable property
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy