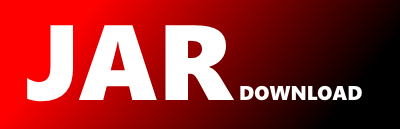
com.pulumi.okta.inputs.UserBaseSchemaPropertyState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserBaseSchemaPropertyState extends com.pulumi.resources.ResourceArgs {
public static final UserBaseSchemaPropertyState Empty = new UserBaseSchemaPropertyState();
/**
* Subschema unique string identifier
*
*/
@Import(name="index")
private @Nullable Output index;
/**
* @return Subschema unique string identifier
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy