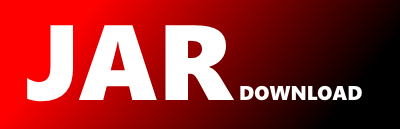
com.pulumi.okta.user.inputs.GetUserPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta Show documentation
Show all versions of okta Show documentation
A Pulumi package for creating and managing okta resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.okta.user.inputs;
import com.pulumi.core.annotations.Import;
import com.pulumi.okta.user.inputs.GetUserSearch;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetUserPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetUserPlainArgs Empty = new GetUserPlainArgs();
/**
* Search operator used when joining multiple search clauses
*
*/
@Import(name="compoundSearchOperator")
private @Nullable String compoundSearchOperator;
/**
* @return Search operator used when joining multiple search clauses
*
*/
public Optional compoundSearchOperator() {
return Optional.ofNullable(this.compoundSearchOperator);
}
/**
* Force delay of the user read by N seconds. Useful when eventual consistency of user information needs to be allowed for.
*
*/
@Import(name="delayReadSeconds")
private @Nullable String delayReadSeconds;
/**
* @return Force delay of the user read by N seconds. Useful when eventual consistency of user information needs to be allowed for.
*
*/
public Optional delayReadSeconds() {
return Optional.ofNullable(this.delayReadSeconds);
}
/**
* Filter to find user/users. Each filter will be concatenated with the compound search operator. Please be aware profile properties must match what is in Okta, which is likely camel case. Expression is a free form expression filter https://developer.okta.com/docs/reference/core-okta-api/#filter . The set name/value/comparison properties will be ignored if expression is present
*
*/
@Import(name="searches")
private @Nullable List searches;
/**
* @return Filter to find user/users. Each filter will be concatenated with the compound search operator. Please be aware profile properties must match what is in Okta, which is likely camel case. Expression is a free form expression filter https://developer.okta.com/docs/reference/core-okta-api/#filter . The set name/value/comparison properties will be ignored if expression is present
*
*/
public Optional> searches() {
return Optional.ofNullable(this.searches);
}
/**
* Do not populate user groups information (prevents additional API call)
*
*/
@Import(name="skipGroups")
private @Nullable Boolean skipGroups;
/**
* @return Do not populate user groups information (prevents additional API call)
*
*/
public Optional skipGroups() {
return Optional.ofNullable(this.skipGroups);
}
/**
* Do not populate user roles information (prevents additional API call)
*
*/
@Import(name="skipRoles")
private @Nullable Boolean skipRoles;
/**
* @return Do not populate user roles information (prevents additional API call)
*
*/
public Optional skipRoles() {
return Optional.ofNullable(this.skipRoles);
}
/**
* Retrieve a single user based on their id
*
*/
@Import(name="userId")
private @Nullable String userId;
/**
* @return Retrieve a single user based on their id
*
*/
public Optional userId() {
return Optional.ofNullable(this.userId);
}
private GetUserPlainArgs() {}
private GetUserPlainArgs(GetUserPlainArgs $) {
this.compoundSearchOperator = $.compoundSearchOperator;
this.delayReadSeconds = $.delayReadSeconds;
this.searches = $.searches;
this.skipGroups = $.skipGroups;
this.skipRoles = $.skipRoles;
this.userId = $.userId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetUserPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetUserPlainArgs $;
public Builder() {
$ = new GetUserPlainArgs();
}
public Builder(GetUserPlainArgs defaults) {
$ = new GetUserPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param compoundSearchOperator Search operator used when joining multiple search clauses
*
* @return builder
*
*/
public Builder compoundSearchOperator(@Nullable String compoundSearchOperator) {
$.compoundSearchOperator = compoundSearchOperator;
return this;
}
/**
* @param delayReadSeconds Force delay of the user read by N seconds. Useful when eventual consistency of user information needs to be allowed for.
*
* @return builder
*
*/
public Builder delayReadSeconds(@Nullable String delayReadSeconds) {
$.delayReadSeconds = delayReadSeconds;
return this;
}
/**
* @param searches Filter to find user/users. Each filter will be concatenated with the compound search operator. Please be aware profile properties must match what is in Okta, which is likely camel case. Expression is a free form expression filter https://developer.okta.com/docs/reference/core-okta-api/#filter . The set name/value/comparison properties will be ignored if expression is present
*
* @return builder
*
*/
public Builder searches(@Nullable List searches) {
$.searches = searches;
return this;
}
/**
* @param searches Filter to find user/users. Each filter will be concatenated with the compound search operator. Please be aware profile properties must match what is in Okta, which is likely camel case. Expression is a free form expression filter https://developer.okta.com/docs/reference/core-okta-api/#filter . The set name/value/comparison properties will be ignored if expression is present
*
* @return builder
*
*/
public Builder searches(GetUserSearch... searches) {
return searches(List.of(searches));
}
/**
* @param skipGroups Do not populate user groups information (prevents additional API call)
*
* @return builder
*
*/
public Builder skipGroups(@Nullable Boolean skipGroups) {
$.skipGroups = skipGroups;
return this;
}
/**
* @param skipRoles Do not populate user roles information (prevents additional API call)
*
* @return builder
*
*/
public Builder skipRoles(@Nullable Boolean skipRoles) {
$.skipRoles = skipRoles;
return this;
}
/**
* @param userId Retrieve a single user based on their id
*
* @return builder
*
*/
public Builder userId(@Nullable String userId) {
$.userId = userId;
return this;
}
public GetUserPlainArgs build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy