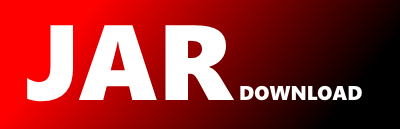
com.pulumi.openstack.sharedfilesystem.ShareNetworkArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.openstack.sharedfilesystem;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ShareNetworkArgs extends com.pulumi.resources.ResourceArgs {
public static final ShareNetworkArgs Empty = new ShareNetworkArgs();
/**
* The human-readable description for the share network.
* Changing this updates the description of the existing share network.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return The human-readable description for the share network.
* Changing this updates the description of the existing share network.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy