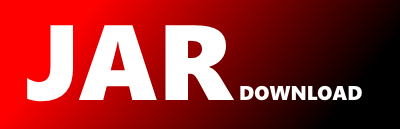
com.pulumi.postgresql.inputs.DefaultPrivilegesState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of postgresql Show documentation
Show all versions of postgresql Show documentation
A Pulumi package for creating and managing postgresql cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.postgresql.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DefaultPrivilegesState extends com.pulumi.resources.ResourceArgs {
public static final DefaultPrivilegesState Empty = new DefaultPrivilegesState();
/**
* The database to grant default privileges for this role.
*
*/
@Import(name="database")
private @Nullable Output database;
/**
* @return The database to grant default privileges for this role.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy