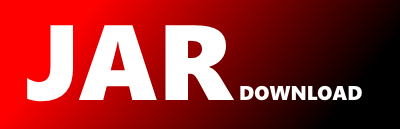
com.pulumi.scm.LdapServerProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.inputs.LdapServerProfileServerArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LdapServerProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final LdapServerProfileArgs Empty = new LdapServerProfileArgs();
/**
* The Base param. String length must not exceed 255 characters.
*
*/
@Import(name="base")
private @Nullable Output base;
/**
* @return The Base param. String length must not exceed 255 characters.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy