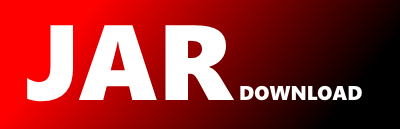
com.pulumi.scm.outputs.GetAuthenticationPortalListData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetAuthenticationPortalListData {
/**
* @return The AuthenticationProfile param.
*
*/
private String authenticationProfile;
/**
* @return The CertificateProfile param.
*
*/
private String certificateProfile;
/**
* @return The GpUdpPort param. Value must be between 1 and 65535.
*
*/
private Integer gpUdpPort;
/**
* @return UUID of the resource.
*
*/
private String id;
/**
* @return The IdleTimer param. Value must be between 1 and 1440.
*
*/
private Integer idleTimer;
/**
* @return The RedirectHost param.
*
*/
private String redirectHost;
/**
* @return The Timer param. Value must be between 1 and 1440.
*
*/
private Integer timer;
/**
* @return The TlsServiceProfile param.
*
*/
private String tlsServiceProfile;
private GetAuthenticationPortalListData() {}
/**
* @return The AuthenticationProfile param.
*
*/
public String authenticationProfile() {
return this.authenticationProfile;
}
/**
* @return The CertificateProfile param.
*
*/
public String certificateProfile() {
return this.certificateProfile;
}
/**
* @return The GpUdpPort param. Value must be between 1 and 65535.
*
*/
public Integer gpUdpPort() {
return this.gpUdpPort;
}
/**
* @return UUID of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The IdleTimer param. Value must be between 1 and 1440.
*
*/
public Integer idleTimer() {
return this.idleTimer;
}
/**
* @return The RedirectHost param.
*
*/
public String redirectHost() {
return this.redirectHost;
}
/**
* @return The Timer param. Value must be between 1 and 1440.
*
*/
public Integer timer() {
return this.timer;
}
/**
* @return The TlsServiceProfile param.
*
*/
public String tlsServiceProfile() {
return this.tlsServiceProfile;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAuthenticationPortalListData defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String authenticationProfile;
private String certificateProfile;
private Integer gpUdpPort;
private String id;
private Integer idleTimer;
private String redirectHost;
private Integer timer;
private String tlsServiceProfile;
public Builder() {}
public Builder(GetAuthenticationPortalListData defaults) {
Objects.requireNonNull(defaults);
this.authenticationProfile = defaults.authenticationProfile;
this.certificateProfile = defaults.certificateProfile;
this.gpUdpPort = defaults.gpUdpPort;
this.id = defaults.id;
this.idleTimer = defaults.idleTimer;
this.redirectHost = defaults.redirectHost;
this.timer = defaults.timer;
this.tlsServiceProfile = defaults.tlsServiceProfile;
}
@CustomType.Setter
public Builder authenticationProfile(String authenticationProfile) {
if (authenticationProfile == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "authenticationProfile");
}
this.authenticationProfile = authenticationProfile;
return this;
}
@CustomType.Setter
public Builder certificateProfile(String certificateProfile) {
if (certificateProfile == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "certificateProfile");
}
this.certificateProfile = certificateProfile;
return this;
}
@CustomType.Setter
public Builder gpUdpPort(Integer gpUdpPort) {
if (gpUdpPort == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "gpUdpPort");
}
this.gpUdpPort = gpUdpPort;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder idleTimer(Integer idleTimer) {
if (idleTimer == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "idleTimer");
}
this.idleTimer = idleTimer;
return this;
}
@CustomType.Setter
public Builder redirectHost(String redirectHost) {
if (redirectHost == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "redirectHost");
}
this.redirectHost = redirectHost;
return this;
}
@CustomType.Setter
public Builder timer(Integer timer) {
if (timer == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "timer");
}
this.timer = timer;
return this;
}
@CustomType.Setter
public Builder tlsServiceProfile(String tlsServiceProfile) {
if (tlsServiceProfile == null) {
throw new MissingRequiredPropertyException("GetAuthenticationPortalListData", "tlsServiceProfile");
}
this.tlsServiceProfile = tlsServiceProfile;
return this;
}
public GetAuthenticationPortalListData build() {
final var _resultValue = new GetAuthenticationPortalListData();
_resultValue.authenticationProfile = authenticationProfile;
_resultValue.certificateProfile = certificateProfile;
_resultValue.gpUdpPort = gpUdpPort;
_resultValue.id = id;
_resultValue.idleTimer = idleTimer;
_resultValue.redirectHost = redirectHost;
_resultValue.timer = timer;
_resultValue.tlsServiceProfile = tlsServiceProfile;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy