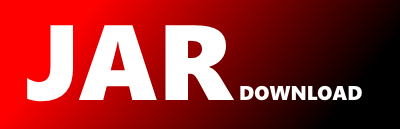
com.pulumi.scm.outputs.GetNatRuleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.outputs.GetNatRuleDestinationTranslation;
import com.pulumi.scm.outputs.GetNatRuleDynamicDestinationTranslation;
import com.pulumi.scm.outputs.GetNatRuleSourceTranslation;
import com.pulumi.scm.outputs.GetNatRuleTarget;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetNatRuleResult {
/**
* @return The ActiveActiveDeviceBinding param. String must be one of these: `"primary"`, `"both"`, `"0"`, `"1"`.
*
*/
private String activeActiveDeviceBinding;
/**
* @return The Description param.
*
*/
private String description;
/**
* @return Static destination translation parameter.
*
*/
private GetNatRuleDestinationTranslation destinationTranslation;
/**
* @return The destination address(es). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
private List destinations;
/**
* @return The device in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
private String device;
/**
* @return The Disabled param.
*
*/
private Boolean disabled;
/**
* @return Dynamic destination translation parameter.
*
*/
private GetNatRuleDynamicDestinationTranslation dynamicDestinationTranslation;
/**
* @return The folder in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
private String folder;
/**
* @return The source security zone(s). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
private List froms;
/**
* @return The GroupTag param.
*
*/
private String groupTag;
/**
* @return The Id param.
*
*/
private String id;
/**
* @return The Name param.
*
*/
private String name;
/**
* @return The NatType param. String must be one of these: `"ipv4"`, `"nat64"`, `"nptv6"`.
*
*/
private String natType;
/**
* @return The Service param.
*
*/
private String service;
/**
* @return The snippet in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
private String snippet;
/**
* @return The SourceTranslation param.
*
*/
private GetNatRuleSourceTranslation sourceTranslation;
/**
* @return The source address(es). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
private List sources;
/**
* @return The Tags param.
*
*/
private List tags;
/**
* @return The Target param.
*
*/
private GetNatRuleTarget target;
private String tfid;
/**
* @return The ToInterface param. String must be one of these: `"any"`.
*
*/
private String toInterface;
/**
* @return The destination security zone(s).
*
*/
private List tos;
private GetNatRuleResult() {}
/**
* @return The ActiveActiveDeviceBinding param. String must be one of these: `"primary"`, `"both"`, `"0"`, `"1"`.
*
*/
public String activeActiveDeviceBinding() {
return this.activeActiveDeviceBinding;
}
/**
* @return The Description param.
*
*/
public String description() {
return this.description;
}
/**
* @return Static destination translation parameter.
*
*/
public GetNatRuleDestinationTranslation destinationTranslation() {
return this.destinationTranslation;
}
/**
* @return The destination address(es). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
public List destinations() {
return this.destinations;
}
/**
* @return The device in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
public String device() {
return this.device;
}
/**
* @return The Disabled param.
*
*/
public Boolean disabled() {
return this.disabled;
}
/**
* @return Dynamic destination translation parameter.
*
*/
public GetNatRuleDynamicDestinationTranslation dynamicDestinationTranslation() {
return this.dynamicDestinationTranslation;
}
/**
* @return The folder in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
public String folder() {
return this.folder;
}
/**
* @return The source security zone(s). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
public List froms() {
return this.froms;
}
/**
* @return The GroupTag param.
*
*/
public String groupTag() {
return this.groupTag;
}
/**
* @return The Id param.
*
*/
public String id() {
return this.id;
}
/**
* @return The Name param.
*
*/
public String name() {
return this.name;
}
/**
* @return The NatType param. String must be one of these: `"ipv4"`, `"nat64"`, `"nptv6"`.
*
*/
public String natType() {
return this.natType;
}
/**
* @return The Service param.
*
*/
public String service() {
return this.service;
}
/**
* @return The snippet in which the resource is defined. String length must not exceed 64 characters. String validation regex: `^[a-zA-Z\d-_\. ]+$`.
*
*/
public String snippet() {
return this.snippet;
}
/**
* @return The SourceTranslation param.
*
*/
public GetNatRuleSourceTranslation sourceTranslation() {
return this.sourceTranslation;
}
/**
* @return The source address(es). Individual elements in this list are subject to additional validation. String must be one of these: `"any"`.
*
*/
public List sources() {
return this.sources;
}
/**
* @return The Tags param.
*
*/
public List tags() {
return this.tags;
}
/**
* @return The Target param.
*
*/
public GetNatRuleTarget target() {
return this.target;
}
public String tfid() {
return this.tfid;
}
/**
* @return The ToInterface param. String must be one of these: `"any"`.
*
*/
public String toInterface() {
return this.toInterface;
}
/**
* @return The destination security zone(s).
*
*/
public List tos() {
return this.tos;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNatRuleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String activeActiveDeviceBinding;
private String description;
private GetNatRuleDestinationTranslation destinationTranslation;
private List destinations;
private String device;
private Boolean disabled;
private GetNatRuleDynamicDestinationTranslation dynamicDestinationTranslation;
private String folder;
private List froms;
private String groupTag;
private String id;
private String name;
private String natType;
private String service;
private String snippet;
private GetNatRuleSourceTranslation sourceTranslation;
private List sources;
private List tags;
private GetNatRuleTarget target;
private String tfid;
private String toInterface;
private List tos;
public Builder() {}
public Builder(GetNatRuleResult defaults) {
Objects.requireNonNull(defaults);
this.activeActiveDeviceBinding = defaults.activeActiveDeviceBinding;
this.description = defaults.description;
this.destinationTranslation = defaults.destinationTranslation;
this.destinations = defaults.destinations;
this.device = defaults.device;
this.disabled = defaults.disabled;
this.dynamicDestinationTranslation = defaults.dynamicDestinationTranslation;
this.folder = defaults.folder;
this.froms = defaults.froms;
this.groupTag = defaults.groupTag;
this.id = defaults.id;
this.name = defaults.name;
this.natType = defaults.natType;
this.service = defaults.service;
this.snippet = defaults.snippet;
this.sourceTranslation = defaults.sourceTranslation;
this.sources = defaults.sources;
this.tags = defaults.tags;
this.target = defaults.target;
this.tfid = defaults.tfid;
this.toInterface = defaults.toInterface;
this.tos = defaults.tos;
}
@CustomType.Setter
public Builder activeActiveDeviceBinding(String activeActiveDeviceBinding) {
if (activeActiveDeviceBinding == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "activeActiveDeviceBinding");
}
this.activeActiveDeviceBinding = activeActiveDeviceBinding;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder destinationTranslation(GetNatRuleDestinationTranslation destinationTranslation) {
if (destinationTranslation == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "destinationTranslation");
}
this.destinationTranslation = destinationTranslation;
return this;
}
@CustomType.Setter
public Builder destinations(List destinations) {
if (destinations == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "destinations");
}
this.destinations = destinations;
return this;
}
public Builder destinations(String... destinations) {
return destinations(List.of(destinations));
}
@CustomType.Setter
public Builder device(String device) {
if (device == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "device");
}
this.device = device;
return this;
}
@CustomType.Setter
public Builder disabled(Boolean disabled) {
if (disabled == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "disabled");
}
this.disabled = disabled;
return this;
}
@CustomType.Setter
public Builder dynamicDestinationTranslation(GetNatRuleDynamicDestinationTranslation dynamicDestinationTranslation) {
if (dynamicDestinationTranslation == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "dynamicDestinationTranslation");
}
this.dynamicDestinationTranslation = dynamicDestinationTranslation;
return this;
}
@CustomType.Setter
public Builder folder(String folder) {
if (folder == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "folder");
}
this.folder = folder;
return this;
}
@CustomType.Setter
public Builder froms(List froms) {
if (froms == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "froms");
}
this.froms = froms;
return this;
}
public Builder froms(String... froms) {
return froms(List.of(froms));
}
@CustomType.Setter
public Builder groupTag(String groupTag) {
if (groupTag == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "groupTag");
}
this.groupTag = groupTag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder natType(String natType) {
if (natType == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "natType");
}
this.natType = natType;
return this;
}
@CustomType.Setter
public Builder service(String service) {
if (service == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "service");
}
this.service = service;
return this;
}
@CustomType.Setter
public Builder snippet(String snippet) {
if (snippet == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "snippet");
}
this.snippet = snippet;
return this;
}
@CustomType.Setter
public Builder sourceTranslation(GetNatRuleSourceTranslation sourceTranslation) {
if (sourceTranslation == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "sourceTranslation");
}
this.sourceTranslation = sourceTranslation;
return this;
}
@CustomType.Setter
public Builder sources(List sources) {
if (sources == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "sources");
}
this.sources = sources;
return this;
}
public Builder sources(String... sources) {
return sources(List.of(sources));
}
@CustomType.Setter
public Builder tags(List tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "tags");
}
this.tags = tags;
return this;
}
public Builder tags(String... tags) {
return tags(List.of(tags));
}
@CustomType.Setter
public Builder target(GetNatRuleTarget target) {
if (target == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "target");
}
this.target = target;
return this;
}
@CustomType.Setter
public Builder tfid(String tfid) {
if (tfid == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "tfid");
}
this.tfid = tfid;
return this;
}
@CustomType.Setter
public Builder toInterface(String toInterface) {
if (toInterface == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "toInterface");
}
this.toInterface = toInterface;
return this;
}
@CustomType.Setter
public Builder tos(List tos) {
if (tos == null) {
throw new MissingRequiredPropertyException("GetNatRuleResult", "tos");
}
this.tos = tos;
return this;
}
public Builder tos(String... tos) {
return tos(List.of(tos));
}
public GetNatRuleResult build() {
final var _resultValue = new GetNatRuleResult();
_resultValue.activeActiveDeviceBinding = activeActiveDeviceBinding;
_resultValue.description = description;
_resultValue.destinationTranslation = destinationTranslation;
_resultValue.destinations = destinations;
_resultValue.device = device;
_resultValue.disabled = disabled;
_resultValue.dynamicDestinationTranslation = dynamicDestinationTranslation;
_resultValue.folder = folder;
_resultValue.froms = froms;
_resultValue.groupTag = groupTag;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.natType = natType;
_resultValue.service = service;
_resultValue.snippet = snippet;
_resultValue.sourceTranslation = sourceTranslation;
_resultValue.sources = sources;
_resultValue.tags = tags;
_resultValue.target = target;
_resultValue.tfid = tfid;
_resultValue.toInterface = toInterface;
_resultValue.tos = tos;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy