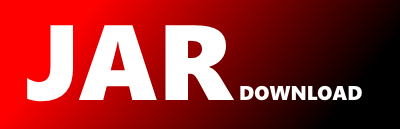
com.pulumi.scm.IpsecTunnelArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.inputs.IpsecTunnelAutoKeyArgs;
import com.pulumi.scm.inputs.IpsecTunnelTunnelMonitorArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IpsecTunnelArgs extends com.pulumi.resources.ResourceArgs {
public static final IpsecTunnelArgs Empty = new IpsecTunnelArgs();
/**
* Enable Anti-Replay check on this tunnel.
*
*/
@Import(name="antiReplay")
private @Nullable Output antiReplay;
/**
* @return Enable Anti-Replay check on this tunnel.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy