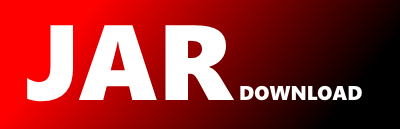
com.pulumi.scm.inputs.DecryptionRuleState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.scm.inputs.DecryptionRuleTypeArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DecryptionRuleState extends com.pulumi.resources.ResourceArgs {
public static final DecryptionRuleState Empty = new DecryptionRuleState();
/**
* The Action param. String must be one of these: `"decrypt"`, `"no-decrypt"`.
*
*/
@Import(name="action")
private @Nullable Output action;
/**
* @return The Action param. String must be one of these: `"decrypt"`, `"no-decrypt"`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy