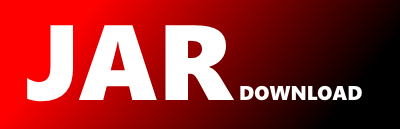
com.pulumi.scm.outputs.GetAuthenticationProfileResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.outputs.GetAuthenticationProfileLockout;
import com.pulumi.scm.outputs.GetAuthenticationProfileMethod;
import com.pulumi.scm.outputs.GetAuthenticationProfileMultiFactorAuth;
import com.pulumi.scm.outputs.GetAuthenticationProfileSingleSignOn;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetAuthenticationProfileResult {
/**
* @return The AllowList param.
*
*/
private List allowLists;
/**
* @return The Id param.
*
*/
private String id;
/**
* @return The Lockout param.
*
*/
private GetAuthenticationProfileLockout lockout;
/**
* @return The Method param.
*
*/
private GetAuthenticationProfileMethod method;
/**
* @return The MultiFactorAuth param.
*
*/
private GetAuthenticationProfileMultiFactorAuth multiFactorAuth;
/**
* @return The Name param.
*
*/
private String name;
/**
* @return The SingleSignOn param.
*
*/
private GetAuthenticationProfileSingleSignOn singleSignOn;
private String tfid;
/**
* @return The UserDomain param. String length must not exceed 63 characters.
*
*/
private String userDomain;
/**
* @return The UsernameModifier param. String must be one of these: `"%USERINPUT%"`, `"%USERINPUT%{@literal @}%USERDOMAIN%"`, `"%USERDOMAIN%\\%USERINPUT%"`.
*
*/
private String usernameModifier;
private GetAuthenticationProfileResult() {}
/**
* @return The AllowList param.
*
*/
public List allowLists() {
return this.allowLists;
}
/**
* @return The Id param.
*
*/
public String id() {
return this.id;
}
/**
* @return The Lockout param.
*
*/
public GetAuthenticationProfileLockout lockout() {
return this.lockout;
}
/**
* @return The Method param.
*
*/
public GetAuthenticationProfileMethod method() {
return this.method;
}
/**
* @return The MultiFactorAuth param.
*
*/
public GetAuthenticationProfileMultiFactorAuth multiFactorAuth() {
return this.multiFactorAuth;
}
/**
* @return The Name param.
*
*/
public String name() {
return this.name;
}
/**
* @return The SingleSignOn param.
*
*/
public GetAuthenticationProfileSingleSignOn singleSignOn() {
return this.singleSignOn;
}
public String tfid() {
return this.tfid;
}
/**
* @return The UserDomain param. String length must not exceed 63 characters.
*
*/
public String userDomain() {
return this.userDomain;
}
/**
* @return The UsernameModifier param. String must be one of these: `"%USERINPUT%"`, `"%USERINPUT%{@literal @}%USERDOMAIN%"`, `"%USERDOMAIN%\\%USERINPUT%"`.
*
*/
public String usernameModifier() {
return this.usernameModifier;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAuthenticationProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List allowLists;
private String id;
private GetAuthenticationProfileLockout lockout;
private GetAuthenticationProfileMethod method;
private GetAuthenticationProfileMultiFactorAuth multiFactorAuth;
private String name;
private GetAuthenticationProfileSingleSignOn singleSignOn;
private String tfid;
private String userDomain;
private String usernameModifier;
public Builder() {}
public Builder(GetAuthenticationProfileResult defaults) {
Objects.requireNonNull(defaults);
this.allowLists = defaults.allowLists;
this.id = defaults.id;
this.lockout = defaults.lockout;
this.method = defaults.method;
this.multiFactorAuth = defaults.multiFactorAuth;
this.name = defaults.name;
this.singleSignOn = defaults.singleSignOn;
this.tfid = defaults.tfid;
this.userDomain = defaults.userDomain;
this.usernameModifier = defaults.usernameModifier;
}
@CustomType.Setter
public Builder allowLists(List allowLists) {
if (allowLists == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "allowLists");
}
this.allowLists = allowLists;
return this;
}
public Builder allowLists(String... allowLists) {
return allowLists(List.of(allowLists));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lockout(GetAuthenticationProfileLockout lockout) {
if (lockout == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "lockout");
}
this.lockout = lockout;
return this;
}
@CustomType.Setter
public Builder method(GetAuthenticationProfileMethod method) {
if (method == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "method");
}
this.method = method;
return this;
}
@CustomType.Setter
public Builder multiFactorAuth(GetAuthenticationProfileMultiFactorAuth multiFactorAuth) {
if (multiFactorAuth == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "multiFactorAuth");
}
this.multiFactorAuth = multiFactorAuth;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder singleSignOn(GetAuthenticationProfileSingleSignOn singleSignOn) {
if (singleSignOn == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "singleSignOn");
}
this.singleSignOn = singleSignOn;
return this;
}
@CustomType.Setter
public Builder tfid(String tfid) {
if (tfid == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "tfid");
}
this.tfid = tfid;
return this;
}
@CustomType.Setter
public Builder userDomain(String userDomain) {
if (userDomain == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "userDomain");
}
this.userDomain = userDomain;
return this;
}
@CustomType.Setter
public Builder usernameModifier(String usernameModifier) {
if (usernameModifier == null) {
throw new MissingRequiredPropertyException("GetAuthenticationProfileResult", "usernameModifier");
}
this.usernameModifier = usernameModifier;
return this;
}
public GetAuthenticationProfileResult build() {
final var _resultValue = new GetAuthenticationProfileResult();
_resultValue.allowLists = allowLists;
_resultValue.id = id;
_resultValue.lockout = lockout;
_resultValue.method = method;
_resultValue.multiFactorAuth = multiFactorAuth;
_resultValue.name = name;
_resultValue.singleSignOn = singleSignOn;
_resultValue.tfid = tfid;
_resultValue.userDomain = userDomain;
_resultValue.usernameModifier = usernameModifier;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy