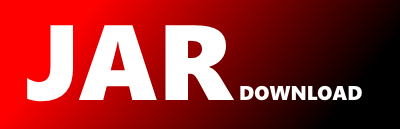
com.pulumi.scm.outputs.GetScepProfileResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.outputs.GetScepProfileAlgorithm;
import com.pulumi.scm.outputs.GetScepProfileCertificateAttributes;
import com.pulumi.scm.outputs.GetScepProfileScepChallenge;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetScepProfileResult {
/**
* @return The Algorithm param.
*
*/
private GetScepProfileAlgorithm algorithm;
/**
* @return The CaIdentityName param.
*
*/
private String caIdentityName;
/**
* @return The CertificateAttributes param.
*
*/
private GetScepProfileCertificateAttributes certificateAttributes;
/**
* @return The Digest param.
*
*/
private String digest;
/**
* @return The Fingerprint param.
*
*/
private String fingerprint;
/**
* @return The Id param.
*
*/
private String id;
/**
* @return alphanumeric string [ 0-9a-zA-Z._-]. String length must not exceed 31 characters.
*
*/
private String name;
/**
* @return The ScepCaCert param.
*
*/
private String scepCaCert;
/**
* @return The ScepChallenge param.
*
*/
private GetScepProfileScepChallenge scepChallenge;
/**
* @return The ScepClientCert param.
*
*/
private String scepClientCert;
/**
* @return The ScepUrl param.
*
*/
private String scepUrl;
/**
* @return The Subject param.
*
*/
private String subject;
private String tfid;
/**
* @return The UseAsDigitalSignature param.
*
*/
private Boolean useAsDigitalSignature;
/**
* @return The UseForKeyEncipherment param.
*
*/
private Boolean useForKeyEncipherment;
private GetScepProfileResult() {}
/**
* @return The Algorithm param.
*
*/
public GetScepProfileAlgorithm algorithm() {
return this.algorithm;
}
/**
* @return The CaIdentityName param.
*
*/
public String caIdentityName() {
return this.caIdentityName;
}
/**
* @return The CertificateAttributes param.
*
*/
public GetScepProfileCertificateAttributes certificateAttributes() {
return this.certificateAttributes;
}
/**
* @return The Digest param.
*
*/
public String digest() {
return this.digest;
}
/**
* @return The Fingerprint param.
*
*/
public String fingerprint() {
return this.fingerprint;
}
/**
* @return The Id param.
*
*/
public String id() {
return this.id;
}
/**
* @return alphanumeric string [ 0-9a-zA-Z._-]. String length must not exceed 31 characters.
*
*/
public String name() {
return this.name;
}
/**
* @return The ScepCaCert param.
*
*/
public String scepCaCert() {
return this.scepCaCert;
}
/**
* @return The ScepChallenge param.
*
*/
public GetScepProfileScepChallenge scepChallenge() {
return this.scepChallenge;
}
/**
* @return The ScepClientCert param.
*
*/
public String scepClientCert() {
return this.scepClientCert;
}
/**
* @return The ScepUrl param.
*
*/
public String scepUrl() {
return this.scepUrl;
}
/**
* @return The Subject param.
*
*/
public String subject() {
return this.subject;
}
public String tfid() {
return this.tfid;
}
/**
* @return The UseAsDigitalSignature param.
*
*/
public Boolean useAsDigitalSignature() {
return this.useAsDigitalSignature;
}
/**
* @return The UseForKeyEncipherment param.
*
*/
public Boolean useForKeyEncipherment() {
return this.useForKeyEncipherment;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScepProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private GetScepProfileAlgorithm algorithm;
private String caIdentityName;
private GetScepProfileCertificateAttributes certificateAttributes;
private String digest;
private String fingerprint;
private String id;
private String name;
private String scepCaCert;
private GetScepProfileScepChallenge scepChallenge;
private String scepClientCert;
private String scepUrl;
private String subject;
private String tfid;
private Boolean useAsDigitalSignature;
private Boolean useForKeyEncipherment;
public Builder() {}
public Builder(GetScepProfileResult defaults) {
Objects.requireNonNull(defaults);
this.algorithm = defaults.algorithm;
this.caIdentityName = defaults.caIdentityName;
this.certificateAttributes = defaults.certificateAttributes;
this.digest = defaults.digest;
this.fingerprint = defaults.fingerprint;
this.id = defaults.id;
this.name = defaults.name;
this.scepCaCert = defaults.scepCaCert;
this.scepChallenge = defaults.scepChallenge;
this.scepClientCert = defaults.scepClientCert;
this.scepUrl = defaults.scepUrl;
this.subject = defaults.subject;
this.tfid = defaults.tfid;
this.useAsDigitalSignature = defaults.useAsDigitalSignature;
this.useForKeyEncipherment = defaults.useForKeyEncipherment;
}
@CustomType.Setter
public Builder algorithm(GetScepProfileAlgorithm algorithm) {
if (algorithm == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "algorithm");
}
this.algorithm = algorithm;
return this;
}
@CustomType.Setter
public Builder caIdentityName(String caIdentityName) {
if (caIdentityName == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "caIdentityName");
}
this.caIdentityName = caIdentityName;
return this;
}
@CustomType.Setter
public Builder certificateAttributes(GetScepProfileCertificateAttributes certificateAttributes) {
if (certificateAttributes == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "certificateAttributes");
}
this.certificateAttributes = certificateAttributes;
return this;
}
@CustomType.Setter
public Builder digest(String digest) {
if (digest == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "digest");
}
this.digest = digest;
return this;
}
@CustomType.Setter
public Builder fingerprint(String fingerprint) {
if (fingerprint == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "fingerprint");
}
this.fingerprint = fingerprint;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder scepCaCert(String scepCaCert) {
if (scepCaCert == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "scepCaCert");
}
this.scepCaCert = scepCaCert;
return this;
}
@CustomType.Setter
public Builder scepChallenge(GetScepProfileScepChallenge scepChallenge) {
if (scepChallenge == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "scepChallenge");
}
this.scepChallenge = scepChallenge;
return this;
}
@CustomType.Setter
public Builder scepClientCert(String scepClientCert) {
if (scepClientCert == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "scepClientCert");
}
this.scepClientCert = scepClientCert;
return this;
}
@CustomType.Setter
public Builder scepUrl(String scepUrl) {
if (scepUrl == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "scepUrl");
}
this.scepUrl = scepUrl;
return this;
}
@CustomType.Setter
public Builder subject(String subject) {
if (subject == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "subject");
}
this.subject = subject;
return this;
}
@CustomType.Setter
public Builder tfid(String tfid) {
if (tfid == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "tfid");
}
this.tfid = tfid;
return this;
}
@CustomType.Setter
public Builder useAsDigitalSignature(Boolean useAsDigitalSignature) {
if (useAsDigitalSignature == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "useAsDigitalSignature");
}
this.useAsDigitalSignature = useAsDigitalSignature;
return this;
}
@CustomType.Setter
public Builder useForKeyEncipherment(Boolean useForKeyEncipherment) {
if (useForKeyEncipherment == null) {
throw new MissingRequiredPropertyException("GetScepProfileResult", "useForKeyEncipherment");
}
this.useForKeyEncipherment = useForKeyEncipherment;
return this;
}
public GetScepProfileResult build() {
final var _resultValue = new GetScepProfileResult();
_resultValue.algorithm = algorithm;
_resultValue.caIdentityName = caIdentityName;
_resultValue.certificateAttributes = certificateAttributes;
_resultValue.digest = digest;
_resultValue.fingerprint = fingerprint;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.scepCaCert = scepCaCert;
_resultValue.scepChallenge = scepChallenge;
_resultValue.scepClientCert = scepClientCert;
_resultValue.scepUrl = scepUrl;
_resultValue.subject = subject;
_resultValue.tfid = tfid;
_resultValue.useAsDigitalSignature = useAsDigitalSignature;
_resultValue.useForKeyEncipherment = useForKeyEncipherment;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy