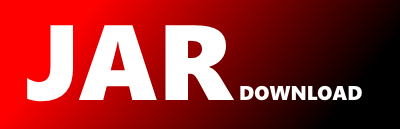
com.pulumi.scm.outputs.GetCertificateProfileListData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scm Show documentation
Show all versions of scm Show documentation
A Pulumi package for managing resources on Strata Cloud Manager.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.scm.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.scm.outputs.GetCertificateProfileListDataCaCertificate;
import com.pulumi.scm.outputs.GetCertificateProfileListDataUsernameField;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetCertificateProfileListData {
/**
* @return The BlockExpiredCert param.
*
*/
private Boolean blockExpiredCert;
/**
* @return The BlockTimeoutCert param.
*
*/
private Boolean blockTimeoutCert;
/**
* @return The BlockUnauthenticatedCert param.
*
*/
private Boolean blockUnauthenticatedCert;
/**
* @return The BlockUnknownCert param.
*
*/
private Boolean blockUnknownCert;
/**
* @return The CaCertificates param.
*
*/
private List caCertificates;
/**
* @return The CertStatusTimeout param.
*
*/
private String certStatusTimeout;
/**
* @return The CrlReceiveTimeout param.
*
*/
private String crlReceiveTimeout;
/**
* @return The Domain param.
*
*/
private String domain;
/**
* @return UUID of the resource.
*
*/
private String id;
/**
* @return Alphanumeric string [ 0-9a-zA-Z._-]. String length must not exceed 63 characters.
*
*/
private String name;
/**
* @return The OcspReceiveTimeout param.
*
*/
private String ocspReceiveTimeout;
/**
* @return The UseCrl param.
*
*/
private Boolean useCrl;
/**
* @return The UseOcsp param.
*
*/
private Boolean useOcsp;
/**
* @return The UsernameField param.
*
*/
private GetCertificateProfileListDataUsernameField usernameField;
private GetCertificateProfileListData() {}
/**
* @return The BlockExpiredCert param.
*
*/
public Boolean blockExpiredCert() {
return this.blockExpiredCert;
}
/**
* @return The BlockTimeoutCert param.
*
*/
public Boolean blockTimeoutCert() {
return this.blockTimeoutCert;
}
/**
* @return The BlockUnauthenticatedCert param.
*
*/
public Boolean blockUnauthenticatedCert() {
return this.blockUnauthenticatedCert;
}
/**
* @return The BlockUnknownCert param.
*
*/
public Boolean blockUnknownCert() {
return this.blockUnknownCert;
}
/**
* @return The CaCertificates param.
*
*/
public List caCertificates() {
return this.caCertificates;
}
/**
* @return The CertStatusTimeout param.
*
*/
public String certStatusTimeout() {
return this.certStatusTimeout;
}
/**
* @return The CrlReceiveTimeout param.
*
*/
public String crlReceiveTimeout() {
return this.crlReceiveTimeout;
}
/**
* @return The Domain param.
*
*/
public String domain() {
return this.domain;
}
/**
* @return UUID of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Alphanumeric string [ 0-9a-zA-Z._-]. String length must not exceed 63 characters.
*
*/
public String name() {
return this.name;
}
/**
* @return The OcspReceiveTimeout param.
*
*/
public String ocspReceiveTimeout() {
return this.ocspReceiveTimeout;
}
/**
* @return The UseCrl param.
*
*/
public Boolean useCrl() {
return this.useCrl;
}
/**
* @return The UseOcsp param.
*
*/
public Boolean useOcsp() {
return this.useOcsp;
}
/**
* @return The UsernameField param.
*
*/
public GetCertificateProfileListDataUsernameField usernameField() {
return this.usernameField;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCertificateProfileListData defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean blockExpiredCert;
private Boolean blockTimeoutCert;
private Boolean blockUnauthenticatedCert;
private Boolean blockUnknownCert;
private List caCertificates;
private String certStatusTimeout;
private String crlReceiveTimeout;
private String domain;
private String id;
private String name;
private String ocspReceiveTimeout;
private Boolean useCrl;
private Boolean useOcsp;
private GetCertificateProfileListDataUsernameField usernameField;
public Builder() {}
public Builder(GetCertificateProfileListData defaults) {
Objects.requireNonNull(defaults);
this.blockExpiredCert = defaults.blockExpiredCert;
this.blockTimeoutCert = defaults.blockTimeoutCert;
this.blockUnauthenticatedCert = defaults.blockUnauthenticatedCert;
this.blockUnknownCert = defaults.blockUnknownCert;
this.caCertificates = defaults.caCertificates;
this.certStatusTimeout = defaults.certStatusTimeout;
this.crlReceiveTimeout = defaults.crlReceiveTimeout;
this.domain = defaults.domain;
this.id = defaults.id;
this.name = defaults.name;
this.ocspReceiveTimeout = defaults.ocspReceiveTimeout;
this.useCrl = defaults.useCrl;
this.useOcsp = defaults.useOcsp;
this.usernameField = defaults.usernameField;
}
@CustomType.Setter
public Builder blockExpiredCert(Boolean blockExpiredCert) {
if (blockExpiredCert == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "blockExpiredCert");
}
this.blockExpiredCert = blockExpiredCert;
return this;
}
@CustomType.Setter
public Builder blockTimeoutCert(Boolean blockTimeoutCert) {
if (blockTimeoutCert == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "blockTimeoutCert");
}
this.blockTimeoutCert = blockTimeoutCert;
return this;
}
@CustomType.Setter
public Builder blockUnauthenticatedCert(Boolean blockUnauthenticatedCert) {
if (blockUnauthenticatedCert == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "blockUnauthenticatedCert");
}
this.blockUnauthenticatedCert = blockUnauthenticatedCert;
return this;
}
@CustomType.Setter
public Builder blockUnknownCert(Boolean blockUnknownCert) {
if (blockUnknownCert == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "blockUnknownCert");
}
this.blockUnknownCert = blockUnknownCert;
return this;
}
@CustomType.Setter
public Builder caCertificates(List caCertificates) {
if (caCertificates == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "caCertificates");
}
this.caCertificates = caCertificates;
return this;
}
public Builder caCertificates(GetCertificateProfileListDataCaCertificate... caCertificates) {
return caCertificates(List.of(caCertificates));
}
@CustomType.Setter
public Builder certStatusTimeout(String certStatusTimeout) {
if (certStatusTimeout == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "certStatusTimeout");
}
this.certStatusTimeout = certStatusTimeout;
return this;
}
@CustomType.Setter
public Builder crlReceiveTimeout(String crlReceiveTimeout) {
if (crlReceiveTimeout == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "crlReceiveTimeout");
}
this.crlReceiveTimeout = crlReceiveTimeout;
return this;
}
@CustomType.Setter
public Builder domain(String domain) {
if (domain == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "domain");
}
this.domain = domain;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder ocspReceiveTimeout(String ocspReceiveTimeout) {
if (ocspReceiveTimeout == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "ocspReceiveTimeout");
}
this.ocspReceiveTimeout = ocspReceiveTimeout;
return this;
}
@CustomType.Setter
public Builder useCrl(Boolean useCrl) {
if (useCrl == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "useCrl");
}
this.useCrl = useCrl;
return this;
}
@CustomType.Setter
public Builder useOcsp(Boolean useOcsp) {
if (useOcsp == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "useOcsp");
}
this.useOcsp = useOcsp;
return this;
}
@CustomType.Setter
public Builder usernameField(GetCertificateProfileListDataUsernameField usernameField) {
if (usernameField == null) {
throw new MissingRequiredPropertyException("GetCertificateProfileListData", "usernameField");
}
this.usernameField = usernameField;
return this;
}
public GetCertificateProfileListData build() {
final var _resultValue = new GetCertificateProfileListData();
_resultValue.blockExpiredCert = blockExpiredCert;
_resultValue.blockTimeoutCert = blockTimeoutCert;
_resultValue.blockUnauthenticatedCert = blockUnauthenticatedCert;
_resultValue.blockUnknownCert = blockUnknownCert;
_resultValue.caCertificates = caCertificates;
_resultValue.certStatusTimeout = certStatusTimeout;
_resultValue.crlReceiveTimeout = crlReceiveTimeout;
_resultValue.domain = domain;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.ocspReceiveTimeout = ocspReceiveTimeout;
_resultValue.useCrl = useCrl;
_resultValue.useOcsp = useOcsp;
_resultValue.usernameField = usernameField;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy