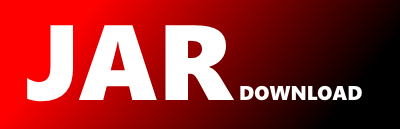
com.pulumi.signalfx.inputs.DashboardGroupState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalfx Show documentation
Show all versions of signalfx Show documentation
A Pulumi package for creating and managing SignalFx resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.signalfx.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.signalfx.inputs.DashboardGroupDashboardArgs;
import com.pulumi.signalfx.inputs.DashboardGroupImportQualifierArgs;
import com.pulumi.signalfx.inputs.DashboardGroupPermissionArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DashboardGroupState extends com.pulumi.resources.ResourceArgs {
public static final DashboardGroupState Empty = new DashboardGroupState();
/**
* Team IDs that have write access to this dashboard group. Remember to use an admin's token if using this feature and to include that admin's team (or user id in `authorized_writer_teams`). **Note:** Deprecated use `permissions` instead.
*
* @deprecated
* Please use permissions field now
*
*/
@Deprecated /* Please use permissions field now */
@Import(name="authorizedWriterTeams")
private @Nullable Output> authorizedWriterTeams;
/**
* @return Team IDs that have write access to this dashboard group. Remember to use an admin's token if using this feature and to include that admin's team (or user id in `authorized_writer_teams`). **Note:** Deprecated use `permissions` instead.
*
* @deprecated
* Please use permissions field now
*
*/
@Deprecated /* Please use permissions field now */
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy