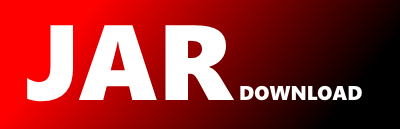
com.pulumi.signalfx.aws.Integration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalfx Show documentation
Show all versions of signalfx Show documentation
A Pulumi package for creating and managing SignalFx resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.signalfx.aws;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.signalfx.Utilities;
import com.pulumi.signalfx.aws.IntegrationArgs;
import com.pulumi.signalfx.aws.inputs.IntegrationState;
import com.pulumi.signalfx.aws.outputs.IntegrationCustomNamespaceSyncRule;
import com.pulumi.signalfx.aws.outputs.IntegrationMetricStatsToSync;
import com.pulumi.signalfx.aws.outputs.IntegrationNamespaceSyncRule;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* AWS CloudWatch integrations for Splunk Observability Cloud. For help with this integration see [Monitoring Amazon Web Services](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/aws/get-awstoc.html).
*
* This resource implements a part of a workflow. Use it with one of either `signalfx.aws.ExternalIntegration` or `signalfx.aws.TokenIntegration`.
*
* > **NOTE** When managing integrations, use a session token of an administrator to authenticate the Splunk Observability provider. See [Operations that require a session token for an administrator](https://dev.splunk.com/observability/docs/administration/authtokens#Operations-that-require-a-session-token-for-an-administrator).
*
* ## Example
*
*/
@ResourceType(type="signalfx:aws/integration:Integration")
public class Integration extends com.pulumi.resources.CustomResource {
/**
* The mechanism used to authenticate with AWS. Use one of `signalfx.aws.ExternalIntegration` or
* `signalfx.aws.TokenIntegration` to define this
*
*/
@Export(name="authMethod", refs={String.class}, tree="[0]")
private Output authMethod;
/**
* @return The mechanism used to authenticate with AWS. Use one of `signalfx.aws.ExternalIntegration` or
* `signalfx.aws.TokenIntegration` to define this
*
*/
public Output authMethod() {
return this.authMethod;
}
/**
* List of custom AWS CloudWatch namespaces to monitor. Custom namespaces contain custom metrics that you define in AWS; Splunk Observability Cloud imports the metrics so you can monitor them.
*
*/
@Export(name="customCloudwatchNamespaces", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> customCloudwatchNamespaces;
/**
* @return List of custom AWS CloudWatch namespaces to monitor. Custom namespaces contain custom metrics that you define in AWS; Splunk Observability Cloud imports the metrics so you can monitor them.
*
*/
public Output>> customCloudwatchNamespaces() {
return Codegen.optional(this.customCloudwatchNamespaces);
}
/**
* Each element controls the data collected by Splunk Observability Cloud for the specified namespace. Conflicts with the `custom_cloudwatch_namespaces` property.
*
*/
@Export(name="customNamespaceSyncRules", refs={List.class,IntegrationCustomNamespaceSyncRule.class}, tree="[0,1]")
private Output* @Nullable */ List> customNamespaceSyncRules;
/**
* @return Each element controls the data collected by Splunk Observability Cloud for the specified namespace. Conflicts with the `custom_cloudwatch_namespaces` property.
*
*/
public Output>> customNamespaceSyncRules() {
return Codegen.optional(this.customNamespaceSyncRules);
}
/**
* Flag that controls how Splunk Observability Cloud imports usage metrics from AWS to use with AWS Cost Optimizer. If `true`, Splunk Observability Cloud imports the metrics.
*
*/
@Export(name="enableAwsUsage", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableAwsUsage;
/**
* @return Flag that controls how Splunk Observability Cloud imports usage metrics from AWS to use with AWS Cost Optimizer. If `true`, Splunk Observability Cloud imports the metrics.
*
*/
public Output> enableAwsUsage() {
return Codegen.optional(this.enableAwsUsage);
}
/**
* Controls how Splunk Observability Cloud checks for large amounts of data for this AWS integration. If `true`, Splunk Observability Cloud monitors the amount of data coming in from the integration.
*
*/
@Export(name="enableCheckLargeVolume", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableCheckLargeVolume;
/**
* @return Controls how Splunk Observability Cloud checks for large amounts of data for this AWS integration. If `true`, Splunk Observability Cloud monitors the amount of data coming in from the integration.
*
*/
public Output> enableCheckLargeVolume() {
return Codegen.optional(this.enableCheckLargeVolume);
}
/**
* Enable the AWS logs synchronization. Note that this requires the inclusion of `"logs:DescribeLogGroups"`, `"logs:DeleteSubscriptionFilter"`, `"logs:DescribeSubscriptionFilters"`, `"logs:PutSubscriptionFilter"`, and `"s3:GetBucketLogging"`, `"s3:GetBucketNotification"`, `"s3:PutBucketNotification"` permissions. Additional permissions may be required to capture logs from specific AWS services.
*
*/
@Export(name="enableLogsSync", refs={Boolean.class}, tree="[0]")
private Output enableLogsSync;
/**
* @return Enable the AWS logs synchronization. Note that this requires the inclusion of `"logs:DescribeLogGroups"`, `"logs:DeleteSubscriptionFilter"`, `"logs:DescribeSubscriptionFilters"`, `"logs:PutSubscriptionFilter"`, and `"s3:GetBucketLogging"`, `"s3:GetBucketNotification"`, `"s3:PutBucketNotification"` permissions. Additional permissions may be required to capture logs from specific AWS services.
*
*/
public Output enableLogsSync() {
return this.enableLogsSync;
}
/**
* Whether the integration is enabled.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output enabled;
/**
* @return Whether the integration is enabled.
*
*/
public Output enabled() {
return this.enabled;
}
/**
* The `external_id` property from one of a `signalfx.aws.ExternalIntegration` or `signalfx.aws.TokenIntegration`
*
*/
@Export(name="externalId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> externalId;
/**
* @return The `external_id` property from one of a `signalfx.aws.ExternalIntegration` or `signalfx.aws.TokenIntegration`
*
*/
public Output> externalId() {
return Codegen.optional(this.externalId);
}
/**
* Flag that controls how Splunk Observability Cloud imports Cloud Watch metrics. If true, Splunk Observability Cloud imports Cloud Watch metrics from AWS.
*
*/
@Export(name="importCloudWatch", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> importCloudWatch;
/**
* @return Flag that controls how Splunk Observability Cloud imports Cloud Watch metrics. If true, Splunk Observability Cloud imports Cloud Watch metrics from AWS.
*
*/
public Output> importCloudWatch() {
return Codegen.optional(this.importCloudWatch);
}
/**
* The id of one of a `signalfx.aws.ExternalIntegration` or `signalfx.aws.TokenIntegration`.
*
*/
@Export(name="integrationId", refs={String.class}, tree="[0]")
private Output integrationId;
/**
* @return The id of one of a `signalfx.aws.ExternalIntegration` or `signalfx.aws.TokenIntegration`.
*
*/
public Output integrationId() {
return this.integrationId;
}
/**
* If you specify `auth_method = \"SecurityToken\"` in your request to create an AWS integration object, use this property to specify the key (this is typically equivalent to the `AWS_SECRET_ACCESS_KEY` environment variable).
*
*/
@Export(name="key", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> key;
/**
* @return If you specify `auth_method = \"SecurityToken\"` in your request to create an AWS integration object, use this property to specify the key (this is typically equivalent to the `AWS_SECRET_ACCESS_KEY` environment variable).
*
*/
public Output> key() {
return Codegen.optional(this.key);
}
/**
* Each element in the array is an object that contains an AWS namespace name, AWS metric name and a list of statistics that Splunk Observability Cloud collects for this metric. If you specify this property, Splunk Observability Cloud retrieves only specified AWS statistics when AWS metric streams are not used. When AWS metric streams are used this property specifies additional extended statistics to collect (please note that AWS metric streams API supports percentile stats only; other stats are ignored). If you don't specify this property, Splunk Observability Cloud retrieves the AWS standard set of statistics.
*
*/
@Export(name="metricStatsToSyncs", refs={List.class,IntegrationMetricStatsToSync.class}, tree="[0,1]")
private Output* @Nullable */ List> metricStatsToSyncs;
/**
* @return Each element in the array is an object that contains an AWS namespace name, AWS metric name and a list of statistics that Splunk Observability Cloud collects for this metric. If you specify this property, Splunk Observability Cloud retrieves only specified AWS statistics when AWS metric streams are not used. When AWS metric streams are used this property specifies additional extended statistics to collect (please note that AWS metric streams API supports percentile stats only; other stats are ignored). If you don't specify this property, Splunk Observability Cloud retrieves the AWS standard set of statistics.
*
*/
public Output>> metricStatsToSyncs() {
return Codegen.optional(this.metricStatsToSyncs);
}
/**
* Name of the integration.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the integration.
*
*/
public Output name() {
return this.name;
}
/**
* Name of the org token to be used for data ingestion. If not specified then default access token is used.
*
*/
@Export(name="namedToken", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namedToken;
/**
* @return Name of the org token to be used for data ingestion. If not specified then default access token is used.
*
*/
public Output> namedToken() {
return Codegen.optional(this.namedToken);
}
/**
* Each element in the array is an object that contains an AWS namespace name and a filter that controls the data that Splunk Observability Cloud collects for the namespace. Conflicts with the `services` property. If you don't specify either property, Splunk Observability Cloud syncs all data in all AWS namespaces.
*
*/
@Export(name="namespaceSyncRules", refs={List.class,IntegrationNamespaceSyncRule.class}, tree="[0,1]")
private Output* @Nullable */ List> namespaceSyncRules;
/**
* @return Each element in the array is an object that contains an AWS namespace name and a filter that controls the data that Splunk Observability Cloud collects for the namespace. Conflicts with the `services` property. If you don't specify either property, Splunk Observability Cloud syncs all data in all AWS namespaces.
*
*/
public Output>> namespaceSyncRules() {
return Codegen.optional(this.namespaceSyncRules);
}
/**
* AWS poll rate (in seconds). Value between `60` and `600`. Default: `300`.
*
*/
@Export(name="pollRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> pollRate;
/**
* @return AWS poll rate (in seconds). Value between `60` and `600`. Default: `300`.
*
*/
public Output> pollRate() {
return Codegen.optional(this.pollRate);
}
/**
* List of AWS regions that Splunk Observability Cloud should monitor. It cannot be empty.
*
*/
@Export(name="regions", refs={List.class,String.class}, tree="[0,1]")
private Output> regions;
/**
* @return List of AWS regions that Splunk Observability Cloud should monitor. It cannot be empty.
*
*/
public Output> regions() {
return this.regions;
}
/**
* Role ARN that you add to an existing AWS integration object. **Note**: Ensure you use the `arn` property of your role, not the id!
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> roleArn;
/**
* @return Role ARN that you add to an existing AWS integration object. **Note**: Ensure you use the `arn` property of your role, not the id!
*
*/
public Output> roleArn() {
return Codegen.optional(this.roleArn);
}
/**
* List of AWS services that you want Splunk Observability Cloud to monitor. Each element is a string designating an AWS service. Can be an empty list to import data for all supported services. Conflicts with `namespace_sync_rule`. See [Amazon Web Services](https://docs.splunk.com/Observability/gdi/get-data-in/integrations.html#amazon-web-services) for a list of valid values.
*
*/
@Export(name="services", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> services;
/**
* @return List of AWS services that you want Splunk Observability Cloud to monitor. Each element is a string designating an AWS service. Can be an empty list to import data for all supported services. Conflicts with `namespace_sync_rule`. See [Amazon Web Services](https://docs.splunk.com/Observability/gdi/get-data-in/integrations.html#amazon-web-services) for a list of valid values.
*
*/
public Output>> services() {
return Codegen.optional(this.services);
}
/**
* Indicates that Splunk Observability Cloud should sync metrics and metadata from custom AWS namespaces only (see the `custom_namespace_sync_rule` above). Defaults to `false`.
*
*/
@Export(name="syncCustomNamespacesOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> syncCustomNamespacesOnly;
/**
* @return Indicates that Splunk Observability Cloud should sync metrics and metadata from custom AWS namespaces only (see the `custom_namespace_sync_rule` above). Defaults to `false`.
*
*/
public Output> syncCustomNamespacesOnly() {
return Codegen.optional(this.syncCustomNamespacesOnly);
}
/**
* If you specify `auth_method = \"SecurityToken\"` in your request to create an AWS integration object, use this property to specify the token (this is typically equivalent to the `AWS_ACCESS_KEY_ID` environment variable).
*
*/
@Export(name="token", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> token;
/**
* @return If you specify `auth_method = \"SecurityToken\"` in your request to create an AWS integration object, use this property to specify the token (this is typically equivalent to the `AWS_ACCESS_KEY_ID` environment variable).
*
*/
public Output> token() {
return Codegen.optional(this.token);
}
/**
* Enable the use of Amazon Cloudwatch Metric Streams for ingesting metrics.<br>
* Note that this requires the inclusion of `"cloudwatch:ListMetricStreams"`,`"cloudwatch:GetMetricStream"`, `"cloudwatch:PutMetricStream"`, `"cloudwatch:DeleteMetricStream"`, `"cloudwatch:StartMetricStreams"`, `"cloudwatch:StopMetricStreams"` and `"iam:PassRole"` permissions.<br>
* Note you need to deploy additional resources on your AWS account to enable CloudWatch metrics streaming. Select one of the [CloudFormation templates](https://docs.splunk.com/Observability/gdi/get-data-in/connect/aws/aws-cloudformation.html) to deploy all the required resources.
*
*/
@Export(name="useMetricStreamsSync", refs={Boolean.class}, tree="[0]")
private Output useMetricStreamsSync;
/**
* @return Enable the use of Amazon Cloudwatch Metric Streams for ingesting metrics.<br>
* Note that this requires the inclusion of `"cloudwatch:ListMetricStreams"`,`"cloudwatch:GetMetricStream"`, `"cloudwatch:PutMetricStream"`, `"cloudwatch:DeleteMetricStream"`, `"cloudwatch:StartMetricStreams"`, `"cloudwatch:StopMetricStreams"` and `"iam:PassRole"` permissions.<br>
* Note you need to deploy additional resources on your AWS account to enable CloudWatch metrics streaming. Select one of the [CloudFormation templates](https://docs.splunk.com/Observability/gdi/get-data-in/connect/aws/aws-cloudformation.html) to deploy all the required resources.
*
*/
public Output useMetricStreamsSync() {
return this.useMetricStreamsSync;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Integration(String name) {
this(name, IntegrationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Integration(String name, IntegrationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Integration(String name, IntegrationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("signalfx:aws/integration:Integration", name, args == null ? IntegrationArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private Integration(String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("signalfx:aws/integration:Integration", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"externalId",
"key"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Integration get(String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Integration(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy