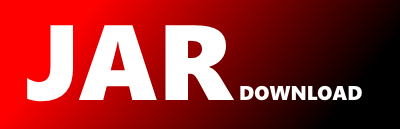
com.pulumi.signalfx.azure.Integration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalfx Show documentation
Show all versions of signalfx Show documentation
A Pulumi package for creating and managing SignalFx resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.signalfx.azure;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.signalfx.Utilities;
import com.pulumi.signalfx.azure.IntegrationArgs;
import com.pulumi.signalfx.azure.inputs.IntegrationState;
import com.pulumi.signalfx.azure.outputs.IntegrationCustomNamespacesPerService;
import com.pulumi.signalfx.azure.outputs.IntegrationResourceFilterRule;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Splunk Observability Cloud Azure integrations. For help with this integration see [Monitoring Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html).
*
* > **NOTE** When managing integrations, use a session token of an administrator to authenticate the Splunk Observability Cloud provider. See [Operations that require a session token for an administrator](https://dev.splunk.com/observability/docs/administration/authtokens#Operations-that-require-a-session-token-for-an-administrator). Otherwise you'll receive a 4xx error.
*
* ## Example
*
*/
@ResourceType(type="signalfx:azure/integration:Integration")
public class Integration extends com.pulumi.resources.CustomResource {
/**
* Additional Azure resource types that you want to sync with Observability Cloud.
*
*/
@Export(name="additionalServices", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> additionalServices;
/**
* @return Additional Azure resource types that you want to sync with Observability Cloud.
*
*/
public Output>> additionalServices() {
return Codegen.optional(this.additionalServices);
}
/**
* Azure application ID for the Splunk Observability Cloud app. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
@Export(name="appId", refs={String.class}, tree="[0]")
private Output appId;
/**
* @return Azure application ID for the Splunk Observability Cloud app. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
public Output appId() {
return this.appId;
}
/**
* Allows for more fine-grained control of syncing of custom namespaces, should the boolean convenience parameter `sync_guest_os_namespaces` be not enough. The customer may specify a map of services to custom namespaces. If they do so, for each service which is a key in this map, we will attempt to sync metrics from namespaces in the value list in addition to the default namespaces.
*
*/
@Export(name="customNamespacesPerServices", refs={List.class,IntegrationCustomNamespacesPerService.class}, tree="[0,1]")
private Output* @Nullable */ List> customNamespacesPerServices;
/**
* @return Allows for more fine-grained control of syncing of custom namespaces, should the boolean convenience parameter `sync_guest_os_namespaces` be not enough. The customer may specify a map of services to custom namespaces. If they do so, for each service which is a key in this map, we will attempt to sync metrics from namespaces in the value list in addition to the default namespaces.
*
*/
public Output>> customNamespacesPerServices() {
return Codegen.optional(this.customNamespacesPerServices);
}
/**
* Whether the integration is enabled.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output enabled;
/**
* @return Whether the integration is enabled.
*
*/
public Output enabled() {
return this.enabled;
}
/**
* What type of Azure integration this is. The allowed values are `\"azure_us_government\"` and `\"azure\"`. Defaults to `\"azure\"`.
*
*/
@Export(name="environment", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> environment;
/**
* @return What type of Azure integration this is. The allowed values are `\"azure_us_government\"` and `\"azure\"`. Defaults to `\"azure\"`.
*
*/
public Output> environment() {
return Codegen.optional(this.environment);
}
/**
* If enabled, Splunk Observability Cloud will sync also Azure Monitor data. If disabled, Splunk Observability Cloud will import only metadata. Defaults to true.
*
*/
@Export(name="importAzureMonitor", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> importAzureMonitor;
/**
* @return If enabled, Splunk Observability Cloud will sync also Azure Monitor data. If disabled, Splunk Observability Cloud will import only metadata. Defaults to true.
*
*/
public Output> importAzureMonitor() {
return Codegen.optional(this.importAzureMonitor);
}
/**
* Name of the integration.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the integration.
*
*/
public Output name() {
return this.name;
}
/**
* Name of the org token to be used for data ingestion. If not specified then default access token is used.
*
*/
@Export(name="namedToken", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namedToken;
/**
* @return Name of the org token to be used for data ingestion. If not specified then default access token is used.
*
*/
public Output> namedToken() {
return Codegen.optional(this.namedToken);
}
/**
* Azure poll rate (in seconds). Value between `60` and `600`. Default: `300`.
*
*/
@Export(name="pollRate", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> pollRate;
/**
* @return Azure poll rate (in seconds). Value between `60` and `600`. Default: `300`.
*
*/
public Output> pollRate() {
return Codegen.optional(this.pollRate);
}
/**
* List of rules for filtering Azure resources by their tags.
*
*/
@Export(name="resourceFilterRules", refs={List.class,IntegrationResourceFilterRule.class}, tree="[0,1]")
private Output* @Nullable */ List> resourceFilterRules;
/**
* @return List of rules for filtering Azure resources by their tags.
*
*/
public Output>> resourceFilterRules() {
return Codegen.optional(this.resourceFilterRules);
}
/**
* Azure secret key that associates the Splunk Observability Cloud app in Azure with the Azure tenant ID. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
@Export(name="secretKey", refs={String.class}, tree="[0]")
private Output secretKey;
/**
* @return Azure secret key that associates the Splunk Observability Cloud app in Azure with the Azure tenant ID. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
public Output secretKey() {
return this.secretKey;
}
/**
* List of Microsoft Azure service names for the Azure services you want Splunk Observability Cloud to monitor. Can be an empty list to import data for all supported services. See [Microsoft Azure services](https://docs.splunk.com/Observability/gdi/get-data-in/integrations.html#azure-integrations) for a list of valid values.
*
*/
@Export(name="services", refs={List.class,String.class}, tree="[0,1]")
private Output> services;
/**
* @return List of Microsoft Azure service names for the Azure services you want Splunk Observability Cloud to monitor. Can be an empty list to import data for all supported services. See [Microsoft Azure services](https://docs.splunk.com/Observability/gdi/get-data-in/integrations.html#azure-integrations) for a list of valid values.
*
*/
public Output> services() {
return this.services;
}
/**
* List of Azure subscriptions that Splunk Observability Cloud should monitor.
*
*/
@Export(name="subscriptions", refs={List.class,String.class}, tree="[0,1]")
private Output> subscriptions;
/**
* @return List of Azure subscriptions that Splunk Observability Cloud should monitor.
*
*/
public Output> subscriptions() {
return this.subscriptions;
}
/**
* If enabled, Splunk Observability Cloud will try to sync additional namespaces for VMs (including VMs in scale sets): telegraf/mem, telegraf/cpu, azure.vm.windows.guest (these are namespaces recommended by Azure when enabling their Diagnostic Extension). If there are no metrics there, no new datapoints will be ingested. Defaults to false.
*
*/
@Export(name="syncGuestOsNamespaces", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> syncGuestOsNamespaces;
/**
* @return If enabled, Splunk Observability Cloud will try to sync additional namespaces for VMs (including VMs in scale sets): telegraf/mem, telegraf/cpu, azure.vm.windows.guest (these are namespaces recommended by Azure when enabling their Diagnostic Extension). If there are no metrics there, no new datapoints will be ingested. Defaults to false.
*
*/
public Output> syncGuestOsNamespaces() {
return Codegen.optional(this.syncGuestOsNamespaces);
}
/**
* Azure ID of the Azure tenant. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
@Export(name="tenantId", refs={String.class}, tree="[0]")
private Output tenantId;
/**
* @return Azure ID of the Azure tenant. To learn how to get this ID, see the topic [Connect to Microsoft Azure](https://docs.splunk.com/observability/en/gdi/get-data-in/connect/azure/azure.html) in the product documentation.
*
*/
public Output tenantId() {
return this.tenantId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Integration(String name) {
this(name, IntegrationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Integration(String name, IntegrationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Integration(String name, IntegrationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("signalfx:azure/integration:Integration", name, args == null ? IntegrationArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private Integration(String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("signalfx:azure/integration:Integration", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"appId",
"environment",
"secretKey"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Integration get(String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Integration(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy