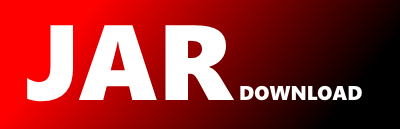
com.pulumi.signalfx.gcp.IntegrationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalfx Show documentation
Show all versions of signalfx Show documentation
A Pulumi package for creating and managing SignalFx resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.signalfx.gcp;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.signalfx.gcp.inputs.IntegrationProjectServiceKeyArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IntegrationArgs extends com.pulumi.resources.ResourceArgs {
public static final IntegrationArgs Empty = new IntegrationArgs();
/**
* List of additional GCP service domain names that Splunk Observability Cloud will monitor. See [Custom Metric Type Domains documentation](https://dev.splunk.com/observability/docs/integrations/gcp_integration_overview/#Custom-metric-type-domains)
*
*/
@Import(name="customMetricTypeDomains")
private @Nullable Output> customMetricTypeDomains;
/**
* @return List of additional GCP service domain names that Splunk Observability Cloud will monitor. See [Custom Metric Type Domains documentation](https://dev.splunk.com/observability/docs/integrations/gcp_integration_overview/#Custom-metric-type-domains)
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy