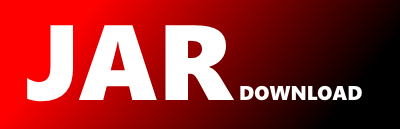
com.pulumi.snowflake.Account Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowflake Show documentation
Show all versions of snowflake Show documentation
A Pulumi package for creating and managing snowflake cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.snowflake;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.snowflake.AccountArgs;
import com.pulumi.snowflake.Utilities;
import com.pulumi.snowflake.inputs.AccountState;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Import
*
* ```sh
* $ pulumi import snowflake:index/account:Account account <account_locator>
* ```
*
*/
@ResourceType(type="snowflake:index/account:Account")
public class Account extends com.pulumi.resources.CustomResource {
/**
* Login name of the initial administrative user of the account. A new user is created in the new account with this name and password and granted the ACCOUNTADMIN role in the account. A login name can be any string consisting of letters, numbers, and underscores. Login names are always case-insensitive.
*
*/
@Export(name="adminName", refs={String.class}, tree="[0]")
private Output adminName;
/**
* @return Login name of the initial administrative user of the account. A new user is created in the new account with this name and password and granted the ACCOUNTADMIN role in the account. A login name can be any string consisting of letters, numbers, and underscores. Login names are always case-insensitive.
*
*/
public Output adminName() {
return this.adminName;
}
/**
* Password for the initial administrative user of the account. Optional if the `ADMIN_RSA_PUBLIC_KEY` parameter is specified. For more information about passwords in Snowflake, see [Snowflake-provided Password Policy](https://docs.snowflake.com/en/sql-reference/sql/create-account.html#:~:text=Snowflake%2Dprovided%20Password%20Policy).
*
*/
@Export(name="adminPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> adminPassword;
/**
* @return Password for the initial administrative user of the account. Optional if the `ADMIN_RSA_PUBLIC_KEY` parameter is specified. For more information about passwords in Snowflake, see [Snowflake-provided Password Policy](https://docs.snowflake.com/en/sql-reference/sql/create-account.html#:~:text=Snowflake%2Dprovided%20Password%20Policy).
*
*/
public Output> adminPassword() {
return Codegen.optional(this.adminPassword);
}
/**
* Assigns a public key to the initial administrative user of the account in order to implement [key pair authentication](https://docs.snowflake.com/en/sql-reference/sql/create-account.html#:~:text=key%20pair%20authentication) for the user. Optional if the `ADMIN_PASSWORD` parameter is specified.
*
*/
@Export(name="adminRsaPublicKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> adminRsaPublicKey;
/**
* @return Assigns a public key to the initial administrative user of the account in order to implement [key pair authentication](https://docs.snowflake.com/en/sql-reference/sql/create-account.html#:~:text=key%20pair%20authentication) for the user. Optional if the `ADMIN_PASSWORD` parameter is specified.
*
*/
public Output> adminRsaPublicKey() {
return Codegen.optional(this.adminRsaPublicKey);
}
/**
* Specifies a comment for the account.
*
*/
@Export(name="comment", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> comment;
/**
* @return Specifies a comment for the account.
*
*/
public Output> comment() {
return Codegen.optional(this.comment);
}
/**
* [Snowflake Edition](https://docs.snowflake.com/en/user-guide/intro-editions.html) of the account. Valid values are: STANDARD | ENTERPRISE | BUSINESS_CRITICAL
*
*/
@Export(name="edition", refs={String.class}, tree="[0]")
private Output edition;
/**
* @return [Snowflake Edition](https://docs.snowflake.com/en/user-guide/intro-editions.html) of the account. Valid values are: STANDARD | ENTERPRISE | BUSINESS_CRITICAL
*
*/
public Output edition() {
return this.edition;
}
/**
* Email address of the initial administrative user of the account. This email address is used to send any notifications about the account.
*
*/
@Export(name="email", refs={String.class}, tree="[0]")
private Output email;
/**
* @return Email address of the initial administrative user of the account. This email address is used to send any notifications about the account.
*
*/
public Output email() {
return this.email;
}
/**
* First name of the initial administrative user of the account
*
*/
@Export(name="firstName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> firstName;
/**
* @return First name of the initial administrative user of the account
*
*/
public Output> firstName() {
return Codegen.optional(this.firstName);
}
/**
* Fully qualified name of the resource. For more information, see [object name resolution](https://docs.snowflake.com/en/sql-reference/name-resolution).
*
*/
@Export(name="fullyQualifiedName", refs={String.class}, tree="[0]")
private Output fullyQualifiedName;
/**
* @return Fully qualified name of the resource. For more information, see [object name resolution](https://docs.snowflake.com/en/sql-reference/name-resolution).
*
*/
public Output fullyQualifiedName() {
return this.fullyQualifiedName;
}
/**
* Specifies the number of days to wait before dropping the account. The default is 3 days.
*
*/
@Export(name="gracePeriodInDays", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> gracePeriodInDays;
/**
* @return Specifies the number of days to wait before dropping the account. The default is 3 days.
*
*/
public Output> gracePeriodInDays() {
return Codegen.optional(this.gracePeriodInDays);
}
/**
* Indicates whether the ORGADMIN role is enabled in an account. If TRUE, the role is enabled.
*
*/
@Export(name="isOrgAdmin", refs={Boolean.class}, tree="[0]")
private Output isOrgAdmin;
/**
* @return Indicates whether the ORGADMIN role is enabled in an account. If TRUE, the role is enabled.
*
*/
public Output isOrgAdmin() {
return this.isOrgAdmin;
}
/**
* Last name of the initial administrative user of the account
*
*/
@Export(name="lastName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lastName;
/**
* @return Last name of the initial administrative user of the account
*
*/
public Output> lastName() {
return Codegen.optional(this.lastName);
}
/**
* Specifies whether the new user created to administer the account is forced to change their password upon first login into the account.
*
*/
@Export(name="mustChangePassword", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> mustChangePassword;
/**
* @return Specifies whether the new user created to administer the account is forced to change their password upon first login into the account.
*
*/
public Output> mustChangePassword() {
return Codegen.optional(this.mustChangePassword);
}
/**
* Specifies the identifier (i.e. name) for the account; must be unique within an organization, regardless of which Snowflake Region the account is in. In addition, the identifier must start with an alphabetic character and cannot contain spaces or special characters except for underscores (_). Note that if the account name includes underscores, features that do not accept account names with underscores (e.g. Okta SSO or SCIM) can reference a version of the account name that substitutes hyphens (-) for the underscores.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the identifier (i.e. name) for the account; must be unique within an organization, regardless of which Snowflake Region the account is in. In addition, the identifier must start with an alphabetic character and cannot contain spaces or special characters except for underscores (_). Note that if the account name includes underscores, features that do not accept account names with underscores (e.g. Okta SSO or SCIM) can reference a version of the account name that substitutes hyphens (-) for the underscores.
*
*/
public Output name() {
return this.name;
}
/**
* ID of the Snowflake Region where the account is created. If no value is provided, Snowflake creates the account in the same Snowflake Region as the current account (i.e. the account in which the CREATE ACCOUNT statement is executed.)
*
*/
@Export(name="region", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> region;
/**
* @return ID of the Snowflake Region where the account is created. If no value is provided, Snowflake creates the account in the same Snowflake Region as the current account (i.e. the account in which the CREATE ACCOUNT statement is executed.)
*
*/
public Output> region() {
return Codegen.optional(this.region);
}
/**
* ID of the Snowflake Region where the account is created. If no value is provided, Snowflake creates the account in the same Snowflake Region as the current account (i.e. the account in which the CREATE ACCOUNT statement is executed.)
*
*/
@Export(name="regionGroup", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> regionGroup;
/**
* @return ID of the Snowflake Region where the account is created. If no value is provided, Snowflake creates the account in the same Snowflake Region as the current account (i.e. the account in which the CREATE ACCOUNT statement is executed.)
*
*/
public Output> regionGroup() {
return Codegen.optional(this.regionGroup);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Account(java.lang.String name) {
this(name, AccountArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Account(java.lang.String name, AccountArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Account(java.lang.String name, AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("snowflake:index/account:Account", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Account(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("snowflake:index/account:Account", name, state, makeResourceOptions(options, id), false);
}
private static AccountArgs makeArgs(AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccountArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"adminPassword",
"adminRsaPublicKey",
"email",
"firstName",
"lastName"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Account get(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Account(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy