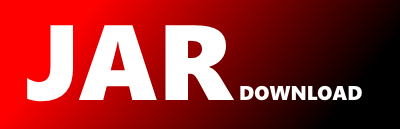
com.pulumi.snowflake.DatabaseOldArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowflake Show documentation
Show all versions of snowflake Show documentation
A Pulumi package for creating and managing snowflake cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.snowflake;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.snowflake.inputs.DatabaseOldReplicationConfigurationArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DatabaseOldArgs extends com.pulumi.resources.ResourceArgs {
public static final DatabaseOldArgs Empty = new DatabaseOldArgs();
/**
* Specifies a comment for the database.
*
*/
@Import(name="comment")
private @Nullable Output comment;
/**
* @return Specifies a comment for the database.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy