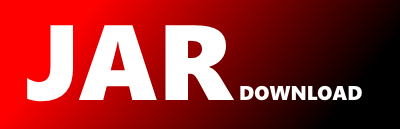
com.pulumi.snowflake.GrantPrivilegesToAccountRoleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowflake Show documentation
Show all versions of snowflake Show documentation
A Pulumi package for creating and managing snowflake cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.snowflake;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.snowflake.inputs.GrantPrivilegesToAccountRoleOnAccountObjectArgs;
import com.pulumi.snowflake.inputs.GrantPrivilegesToAccountRoleOnSchemaArgs;
import com.pulumi.snowflake.inputs.GrantPrivilegesToAccountRoleOnSchemaObjectArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GrantPrivilegesToAccountRoleArgs extends com.pulumi.resources.ResourceArgs {
public static final GrantPrivilegesToAccountRoleArgs Empty = new GrantPrivilegesToAccountRoleArgs();
/**
* The fully qualified name of the account role to which privileges will be granted.
*
*/
@Import(name="accountRoleName", required=true)
private Output accountRoleName;
/**
* @return The fully qualified name of the account role to which privileges will be granted.
*
*/
public Output accountRoleName() {
return this.accountRoleName;
}
/**
* Grant all privileges on the account role.
*
*/
@Import(name="allPrivileges")
private @Nullable Output allPrivileges;
/**
* @return Grant all privileges on the account role.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy