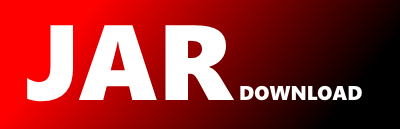
com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowflake Show documentation
Show all versions of snowflake Show documentation
A Pulumi package for creating and managing snowflake cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.snowflake.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputAuthType;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputComment;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputEnabled;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAccessTokenValidity;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAllowedScope;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAuthorizationEndpoint;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthClientAuthMethod;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthClientId;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthGrant;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthRefreshTokenValidity;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthTokenEndpoint;
import com.pulumi.snowflake.outputs.ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputParentIntegration;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput {
private @Nullable List authTypes;
private @Nullable List comments;
private @Nullable List enableds;
private @Nullable List oauthAccessTokenValidities;
private @Nullable List oauthAllowedScopes;
private @Nullable List oauthAuthorizationEndpoints;
private @Nullable List oauthClientAuthMethods;
private @Nullable List oauthClientIds;
private @Nullable List oauthGrants;
private @Nullable List oauthRefreshTokenValidities;
private @Nullable List oauthTokenEndpoints;
private @Nullable List parentIntegrations;
private ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput() {}
public List authTypes() {
return this.authTypes == null ? List.of() : this.authTypes;
}
public List comments() {
return this.comments == null ? List.of() : this.comments;
}
public List enableds() {
return this.enableds == null ? List.of() : this.enableds;
}
public List oauthAccessTokenValidities() {
return this.oauthAccessTokenValidities == null ? List.of() : this.oauthAccessTokenValidities;
}
public List oauthAllowedScopes() {
return this.oauthAllowedScopes == null ? List.of() : this.oauthAllowedScopes;
}
public List oauthAuthorizationEndpoints() {
return this.oauthAuthorizationEndpoints == null ? List.of() : this.oauthAuthorizationEndpoints;
}
public List oauthClientAuthMethods() {
return this.oauthClientAuthMethods == null ? List.of() : this.oauthClientAuthMethods;
}
public List oauthClientIds() {
return this.oauthClientIds == null ? List.of() : this.oauthClientIds;
}
public List oauthGrants() {
return this.oauthGrants == null ? List.of() : this.oauthGrants;
}
public List oauthRefreshTokenValidities() {
return this.oauthRefreshTokenValidities == null ? List.of() : this.oauthRefreshTokenValidities;
}
public List oauthTokenEndpoints() {
return this.oauthTokenEndpoints == null ? List.of() : this.oauthTokenEndpoints;
}
public List parentIntegrations() {
return this.parentIntegrations == null ? List.of() : this.parentIntegrations;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List authTypes;
private @Nullable List comments;
private @Nullable List enableds;
private @Nullable List oauthAccessTokenValidities;
private @Nullable List oauthAllowedScopes;
private @Nullable List oauthAuthorizationEndpoints;
private @Nullable List oauthClientAuthMethods;
private @Nullable List oauthClientIds;
private @Nullable List oauthGrants;
private @Nullable List oauthRefreshTokenValidities;
private @Nullable List oauthTokenEndpoints;
private @Nullable List parentIntegrations;
public Builder() {}
public Builder(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput defaults) {
Objects.requireNonNull(defaults);
this.authTypes = defaults.authTypes;
this.comments = defaults.comments;
this.enableds = defaults.enableds;
this.oauthAccessTokenValidities = defaults.oauthAccessTokenValidities;
this.oauthAllowedScopes = defaults.oauthAllowedScopes;
this.oauthAuthorizationEndpoints = defaults.oauthAuthorizationEndpoints;
this.oauthClientAuthMethods = defaults.oauthClientAuthMethods;
this.oauthClientIds = defaults.oauthClientIds;
this.oauthGrants = defaults.oauthGrants;
this.oauthRefreshTokenValidities = defaults.oauthRefreshTokenValidities;
this.oauthTokenEndpoints = defaults.oauthTokenEndpoints;
this.parentIntegrations = defaults.parentIntegrations;
}
@CustomType.Setter
public Builder authTypes(@Nullable List authTypes) {
this.authTypes = authTypes;
return this;
}
public Builder authTypes(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputAuthType... authTypes) {
return authTypes(List.of(authTypes));
}
@CustomType.Setter
public Builder comments(@Nullable List comments) {
this.comments = comments;
return this;
}
public Builder comments(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputComment... comments) {
return comments(List.of(comments));
}
@CustomType.Setter
public Builder enableds(@Nullable List enableds) {
this.enableds = enableds;
return this;
}
public Builder enableds(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputEnabled... enableds) {
return enableds(List.of(enableds));
}
@CustomType.Setter
public Builder oauthAccessTokenValidities(@Nullable List oauthAccessTokenValidities) {
this.oauthAccessTokenValidities = oauthAccessTokenValidities;
return this;
}
public Builder oauthAccessTokenValidities(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAccessTokenValidity... oauthAccessTokenValidities) {
return oauthAccessTokenValidities(List.of(oauthAccessTokenValidities));
}
@CustomType.Setter
public Builder oauthAllowedScopes(@Nullable List oauthAllowedScopes) {
this.oauthAllowedScopes = oauthAllowedScopes;
return this;
}
public Builder oauthAllowedScopes(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAllowedScope... oauthAllowedScopes) {
return oauthAllowedScopes(List.of(oauthAllowedScopes));
}
@CustomType.Setter
public Builder oauthAuthorizationEndpoints(@Nullable List oauthAuthorizationEndpoints) {
this.oauthAuthorizationEndpoints = oauthAuthorizationEndpoints;
return this;
}
public Builder oauthAuthorizationEndpoints(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthAuthorizationEndpoint... oauthAuthorizationEndpoints) {
return oauthAuthorizationEndpoints(List.of(oauthAuthorizationEndpoints));
}
@CustomType.Setter
public Builder oauthClientAuthMethods(@Nullable List oauthClientAuthMethods) {
this.oauthClientAuthMethods = oauthClientAuthMethods;
return this;
}
public Builder oauthClientAuthMethods(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthClientAuthMethod... oauthClientAuthMethods) {
return oauthClientAuthMethods(List.of(oauthClientAuthMethods));
}
@CustomType.Setter
public Builder oauthClientIds(@Nullable List oauthClientIds) {
this.oauthClientIds = oauthClientIds;
return this;
}
public Builder oauthClientIds(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthClientId... oauthClientIds) {
return oauthClientIds(List.of(oauthClientIds));
}
@CustomType.Setter
public Builder oauthGrants(@Nullable List oauthGrants) {
this.oauthGrants = oauthGrants;
return this;
}
public Builder oauthGrants(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthGrant... oauthGrants) {
return oauthGrants(List.of(oauthGrants));
}
@CustomType.Setter
public Builder oauthRefreshTokenValidities(@Nullable List oauthRefreshTokenValidities) {
this.oauthRefreshTokenValidities = oauthRefreshTokenValidities;
return this;
}
public Builder oauthRefreshTokenValidities(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthRefreshTokenValidity... oauthRefreshTokenValidities) {
return oauthRefreshTokenValidities(List.of(oauthRefreshTokenValidities));
}
@CustomType.Setter
public Builder oauthTokenEndpoints(@Nullable List oauthTokenEndpoints) {
this.oauthTokenEndpoints = oauthTokenEndpoints;
return this;
}
public Builder oauthTokenEndpoints(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputOauthTokenEndpoint... oauthTokenEndpoints) {
return oauthTokenEndpoints(List.of(oauthTokenEndpoints));
}
@CustomType.Setter
public Builder parentIntegrations(@Nullable List parentIntegrations) {
this.parentIntegrations = parentIntegrations;
return this;
}
public Builder parentIntegrations(ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutputParentIntegration... parentIntegrations) {
return parentIntegrations(List.of(parentIntegrations));
}
public ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput build() {
final var _resultValue = new ApiAuthenticationIntegrationWithAuthorizationCodeGrantDescribeOutput();
_resultValue.authTypes = authTypes;
_resultValue.comments = comments;
_resultValue.enableds = enableds;
_resultValue.oauthAccessTokenValidities = oauthAccessTokenValidities;
_resultValue.oauthAllowedScopes = oauthAllowedScopes;
_resultValue.oauthAuthorizationEndpoints = oauthAuthorizationEndpoints;
_resultValue.oauthClientAuthMethods = oauthClientAuthMethods;
_resultValue.oauthClientIds = oauthClientIds;
_resultValue.oauthGrants = oauthGrants;
_resultValue.oauthRefreshTokenValidities = oauthRefreshTokenValidities;
_resultValue.oauthTokenEndpoints = oauthTokenEndpoints;
_resultValue.parentIntegrations = parentIntegrations;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy