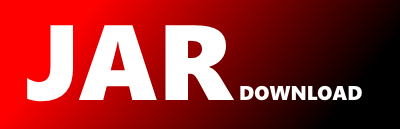
com.pulumi.snowflake.outputs.StreamOnTableBefore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowflake Show documentation
Show all versions of snowflake Show documentation
A Pulumi package for creating and managing snowflake cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.snowflake.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class StreamOnTableBefore {
/**
* @return Specifies the difference in seconds from the current time to use for Time Travel, in the form -N where N can be an integer or arithmetic expression (e.g. -120 is 120 seconds, -30*60 is 1800 seconds or 30 minutes).
*
*/
private @Nullable String offset;
/**
* @return Specifies the query ID of a statement to use as the reference point for Time Travel. This parameter supports any statement of one of the following types: DML (e.g. INSERT, UPDATE, DELETE), TCL (BEGIN, COMMIT transaction), SELECT.
*
*/
private @Nullable String statement;
/**
* @return Specifies the identifier (i.e. name) for an existing stream on the queried table or view. The current offset in the stream is used as the AT point in time for returning change data for the source object.
*
*/
private @Nullable String stream;
/**
* @return Specifies an exact date and time to use for Time Travel. The value must be explicitly cast to a TIMESTAMP, TIMESTAMP*LTZ, TIMESTAMP*NTZ, or TIMESTAMP_TZ data type.
*
*/
private @Nullable String timestamp;
private StreamOnTableBefore() {}
/**
* @return Specifies the difference in seconds from the current time to use for Time Travel, in the form -N where N can be an integer or arithmetic expression (e.g. -120 is 120 seconds, -30*60 is 1800 seconds or 30 minutes).
*
*/
public Optional offset() {
return Optional.ofNullable(this.offset);
}
/**
* @return Specifies the query ID of a statement to use as the reference point for Time Travel. This parameter supports any statement of one of the following types: DML (e.g. INSERT, UPDATE, DELETE), TCL (BEGIN, COMMIT transaction), SELECT.
*
*/
public Optional statement() {
return Optional.ofNullable(this.statement);
}
/**
* @return Specifies the identifier (i.e. name) for an existing stream on the queried table or view. The current offset in the stream is used as the AT point in time for returning change data for the source object.
*
*/
public Optional stream() {
return Optional.ofNullable(this.stream);
}
/**
* @return Specifies an exact date and time to use for Time Travel. The value must be explicitly cast to a TIMESTAMP, TIMESTAMP*LTZ, TIMESTAMP*NTZ, or TIMESTAMP_TZ data type.
*
*/
public Optional timestamp() {
return Optional.ofNullable(this.timestamp);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(StreamOnTableBefore defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String offset;
private @Nullable String statement;
private @Nullable String stream;
private @Nullable String timestamp;
public Builder() {}
public Builder(StreamOnTableBefore defaults) {
Objects.requireNonNull(defaults);
this.offset = defaults.offset;
this.statement = defaults.statement;
this.stream = defaults.stream;
this.timestamp = defaults.timestamp;
}
@CustomType.Setter
public Builder offset(@Nullable String offset) {
this.offset = offset;
return this;
}
@CustomType.Setter
public Builder statement(@Nullable String statement) {
this.statement = statement;
return this;
}
@CustomType.Setter
public Builder stream(@Nullable String stream) {
this.stream = stream;
return this;
}
@CustomType.Setter
public Builder timestamp(@Nullable String timestamp) {
this.timestamp = timestamp;
return this;
}
public StreamOnTableBefore build() {
final var _resultValue = new StreamOnTableBefore();
_resultValue.offset = offset;
_resultValue.statement = statement;
_resultValue.stream = stream;
_resultValue.timestamp = timestamp;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy