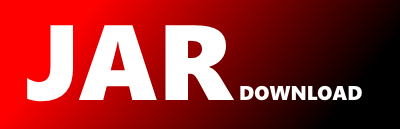
com.pulumi.splunk.inputs.AppsLocalState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of splunk Show documentation
Show all versions of splunk Show documentation
A Pulumi package for creating and managing splunk cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.splunk.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.splunk.inputs.AppsLocalAclArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AppsLocalState extends com.pulumi.resources.ResourceArgs {
public static final AppsLocalState Empty = new AppsLocalState();
/**
* The app/user context that is the namespace for the resource
*
*/
@Import(name="acl")
private @Nullable Output acl;
/**
* @return The app/user context that is the namespace for the resource
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy