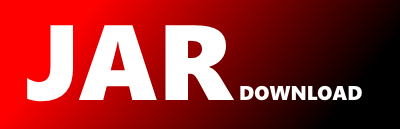
com.pulumi.splunk.AuthenticationUsersArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of splunk Show documentation
Show all versions of splunk Show documentation
A Pulumi package for creating and managing splunk cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.splunk;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AuthenticationUsersArgs extends com.pulumi.resources.ResourceArgs {
public static final AuthenticationUsersArgs Empty = new AuthenticationUsersArgs();
/**
* User default app. Overrides the default app inherited from the user roles.
*
*/
@Import(name="defaultApp")
private @Nullable Output defaultApp;
/**
* @return User default app. Overrides the default app inherited from the user roles.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy